ExecutorService and Future
In Runnable interface, we have run method which doesn’t return any value. Sometimes, we need to return some value. In that situation, there is an interface called Callable which has call method.
Signature of call() method
V call() throws Exception;
Callable interface
package java.util.concurrent;
@FunctionalInterface
public interface Callable<V> {
/**
* Computes a result, or throws an exception if unable to do so.
*
* @return computed result
* @throws Exception if unable to compute a result
*/
V call() throws Exception;
}
Executor Service Example
Write a callable task class
package com.sks.executor;
import java.util.concurrent.Callable;
public class CallableTask implements Callable<String> {
@Override
public String call() throws Exception {
Thread.sleep(4000);
return " this line is executed by : " + Thread.currentThread().getName();
}
}
Test Class
package com.sks.executor;
import java.util.concurrent.*;
public class CallableTest {
public static void main(String[] args) {
Callable task = new CallableTask();
ExecutorService service = Executors.newFixedThreadPool(10);
Future<String> value = service.submit(task);
System.out.println("main proceeded");
String result = null;
try {
result = value.get();
} catch (InterruptedException e) {
throw new RuntimeException(e);
} catch (ExecutionException e) {
throw new RuntimeException(e);
} finally {
service.shutdown();
}
System.out.println(result);
System.out.println("main ended gracefully");
}
}
Output:
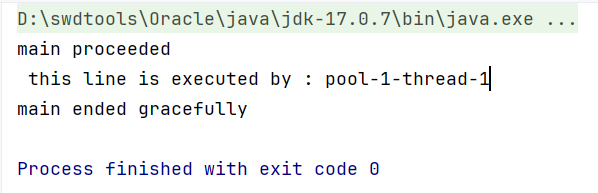
- Home
- Java
- Java Fundamentals
- What is Java
- History of Java
- Java Version History
- Local Environment Set-up
- First Java Program
- How to set ‘Path’ env variable
- JDK, JRE, and JVM
- Object Oriented Programming
- Java Data Types
- Classes in Java
- Objects in Java
- Interfaces in java
- Class attributes and methods
- Methods In Java
- Variables and Constants in Java
- Java packages and Imports
- Access Modifiers In Java
- Java Operators
- Constructors in Java
- Control Statements – If else
- Control Statements – Loops
- Arrays in Java
- Java Abstraction
- Java Inheritance
- Polymorphism
- Java Exception Handling
- Strings in Java
- File IO
- Java Miscellaneous
- Design Patterns
- Java Fundamentals
- Collections
- Multithreading
- Java New Features
- Servlet
- JSP
- Spring
- Hibernate
- Architecture
- Hibernate Example
- First Hibernate Application (using xml configuration)
- First Hibernate Application (using annotations)
- JPA/HB – annotations
- Hibernate Identifiers
- Hibernate Generator Classes
- Save vs saveOrUpdate vs persist in Hibernate
- Inheritance Mapping in Hibernate
- Inheritance Mapping using annotations
- Hibernate Mapping
- Hibernate Query Language (HQL)
- HCQL Hibernate Criteria Query Language
- Hibernate Named Query
- Hibernate Caching
- Second Level Cache
- Spring Boot
- Spring Boot Basics
- Spring Boot Web
- Spring Boot Exception Handling
- Service discovery using Netflix Eureka
- Springboot OpenAPI/Swagger3
- Zuul Proxy Server + Routing
- Spring Cloud Gateway
- Spring Boot Security
- Circuit Breaker using Spring Boot Hystrix
- Interservice Communication
- Spring Boot Hateoas Links Example
- Lombok api
- Spring Boot with Mongo DB
- Load Balancer in Springboot
- Spring Boot Testing
- Spring Web Flux
- Database
- Web Service
- HTML
- Blog & Programs
- Docker
- Trainings
- Home
- Java
- Java Fundamentals
- What is Java
- History of Java
- Java Version History
- Local Environment Set-up
- First Java Program
- How to set ‘Path’ env variable
- JDK, JRE, and JVM
- Object Oriented Programming
- Java Data Types
- Classes in Java
- Objects in Java
- Interfaces in java
- Class attributes and methods
- Methods In Java
- Variables and Constants in Java
- Java packages and Imports
- Access Modifiers In Java
- Java Operators
- Constructors in Java
- Control Statements – If else
- Control Statements – Loops
- Arrays in Java
- Java Abstraction
- Java Inheritance
- Polymorphism
- Java Exception Handling
- Strings in Java
- File IO
- Java Miscellaneous
- Design Patterns
- Java Fundamentals
- Collections
- Multithreading
- Java New Features
- Servlet
- JSP
- Spring
- Hibernate
- Architecture
- Hibernate Example
- First Hibernate Application (using xml configuration)
- First Hibernate Application (using annotations)
- JPA/HB – annotations
- Hibernate Identifiers
- Hibernate Generator Classes
- Save vs saveOrUpdate vs persist in Hibernate
- Inheritance Mapping in Hibernate
- Inheritance Mapping using annotations
- Hibernate Mapping
- Hibernate Query Language (HQL)
- HCQL Hibernate Criteria Query Language
- Hibernate Named Query
- Hibernate Caching
- Second Level Cache
- Spring Boot
- Spring Boot Basics
- Spring Boot Web
- Spring Boot Exception Handling
- Service discovery using Netflix Eureka
- Springboot OpenAPI/Swagger3
- Zuul Proxy Server + Routing
- Spring Cloud Gateway
- Spring Boot Security
- Circuit Breaker using Spring Boot Hystrix
- Interservice Communication
- Spring Boot Hateoas Links Example
- Lombok api
- Spring Boot with Mongo DB
- Load Balancer in Springboot
- Spring Boot Testing
- Spring Web Flux
- Database
- Web Service
- HTML
- Blog & Programs
- Docker
- Trainings