Inheritance
Inheritance is a concept in which properties and behaviour of one class are inherited by another class. The class whose properties are inherited is called parent class, whereas the class that inherits properties and behaviour is called child class.
In programming world, Inheritance is a way of making relationships between classes and use these relationships to make coherent programs. Code reusability is another aspect of inheritance. Let’s take few examples to understand
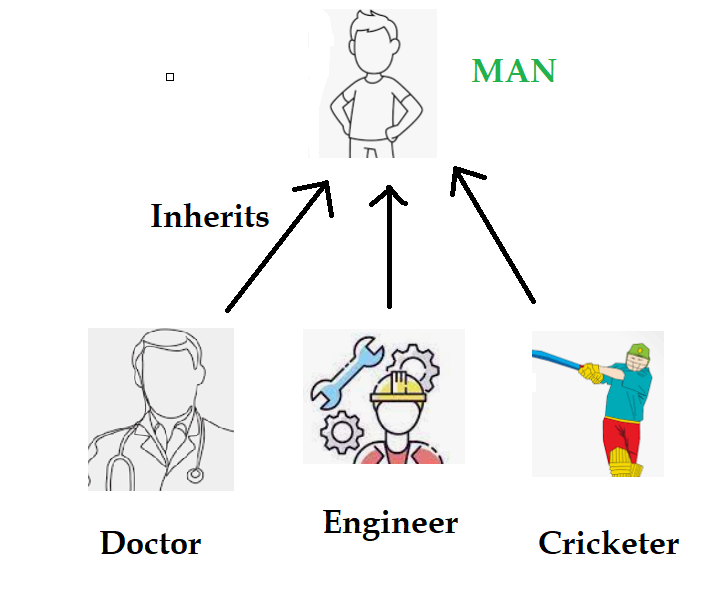
In the above example, we can see a Doctor, an engineer, and a cricketer, all of them inherits some properties of a man like two eyes, two hands, one nose etc, and some of the behaviour of a man like eating, sleeping, breathig etc. At the same time, doctors, engineers, and cricketers have their own properties and behaviour as well. This phenomenon is known as inheritance.
IS-A relationship
Inheritance represents IS-A relationship between classes. For example, in the above diagram where Doctor, Engineer, and Cricketer is extending Man class, we can say Doctor IS-A Man, Engineer IS-A Man, Cricketer IS-A Man.
Some important terms used in inheritance
- Parent class or Super class : It is the class whose properties and behaviour are inherited.
- Child class or subclass : It is the class which inherits properties and behaviour from a parent class
- extends : It is the keyword which is used to establish relationship between a parent class and a child class
Syntax to define inheritance
class One extends Two
To establish the parent child relationship between the two classes, we have to use keyword ‘extends’. In the above example, class One is the child class of class Two.
In the below example, we will establish a relationship between the two classes: Man and Doctor, where Doctor extends Man class.
package com.javatrainingschool;
public class Man {
String name;
String address;
String gender;
public Man(String name, String address, String gender) {
super();
this.name = name;
this.address = address;
this.gender = gender;
}
public void breathe(){
System.out.println("Man breathes.");
}
}
package com.javatrainingschool;
public class Doctor extends Man {
//all non private properties of man class are inherited here
public Doctor(String name, String address, String gender) {
super(name, address, gender);
}
public static void main(String[] args) {
Doctor doctor = new Doctor("Anjali", "Test Address", "Female");
System.out.println(doctor.name);
System.out.println(doctor.address);
System.out.println(doctor.gender);
}
}
Output :
Anjali
Test Address
Female
In the above example Doctor class extends man class. Here, Man become parent or super class. Whereas, Doctor class becomes child or subclass of Man class. Points to remeber here
- Man is parent class. Doctor is child class.
- Properties name and address of Man class are inherited by Doctor class.
- Method breathe() is also inherited by Doctor class.
- Man is a generalized class, whereas Doctor is a specialized form of Man class.
Rules of inheritance
There are certain rules of inheritance. Let’s have a look at them.
- One class can be extended by any number of subclasses.
- One class can extend only one class at a time.
- public, protected members are inherited by the child class.
- Default members are inherited if the child class lies in the same package as parent class.
- Private members cannot be inherited.
- Constructors, static initializers, and instance initializers are not inherited.
- Static members can also be accessed from the child classes if they are not private.
Types of inheritance
- Single
- Multiple
- Hierarchical
- Multilevel
- Hybrid
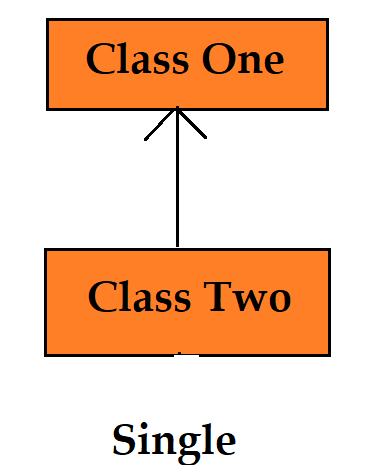
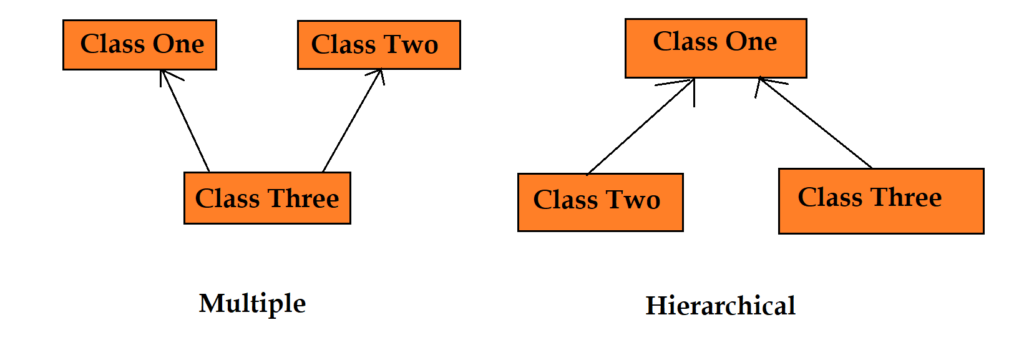
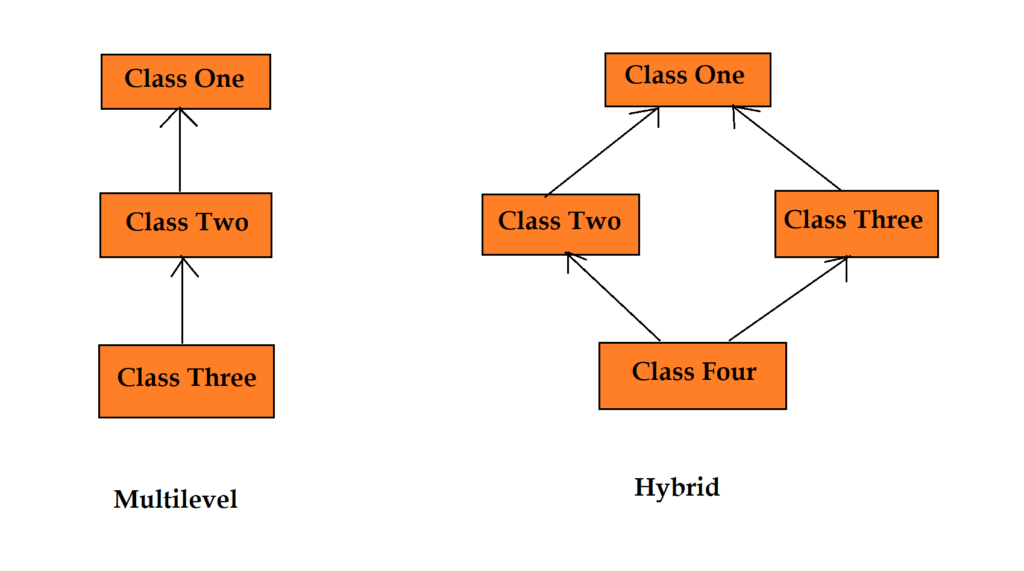
Note -> Multiple and Hybrid type inheritance is not allowed in Java. Hybrid type is also known as Diamond problem. To understand more about Diamond problem, click here.
Example of Single Type Inheritance
We have already seen this example above where one class extend another class. In our example, Doctor class extends Man class.
Example of Multilevel Type Inheritance
In this example, Man is the top level class. Doctor is the child class of Man, and Dermatologist is the child class of Doctor. This is an example of multilevel inheritance.
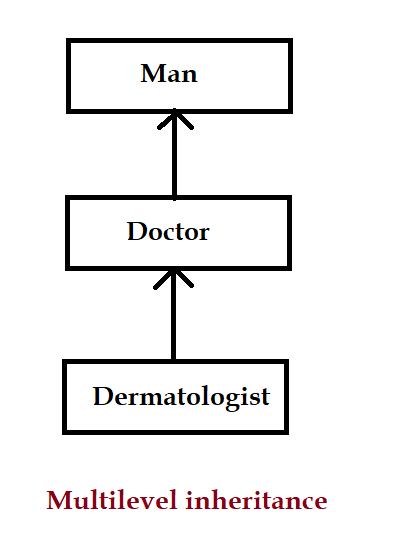
Man Class
package com.javatrainingschool;
public class Man {
String name;
String address;
String gender;
public Man(String name, String address, String gender) {
super();
this.name = name;
this.address = address;
this.gender = gender;
}
public void breathe(){
System.out.println("Man breathes.");
}
//getter and setter methods
}
Doctor class that extends Man
package com.javatrainingschool;
public class Doctor extends Man {
private String hospital;
public Doctor(String hospital, String name, String address, String gender) {
super(name, address, gender);
this.hospital = hospital;
}
public String getHospital() {
return hospital;
}
public void setHospital(String hospital) {
this.hospital = hospital;
}
}
Dermatologist class that extends Doctor
package com.javatrainingschool;
public class Dermatologist extends Doctor {
private String department;
public Dermatologist(String hospital, String name, String address, String gender, String department) {
super(hospital, name, address, gender);
this.department = department;
}
public String getDepartment() {
return department;
}
public void setDepartment(String department) {
this.department = department;
}
}
Main Class
package com.javatrainingschool;
public class MainClass {
public static void main(String[] args) {
Dermatologist doctor = new Dermatologist("XYZ", "K Singh", "Kanpur", "Female", "Dermatology");
System.out.println(doctor.getHospital());
System.out.println(doctor.getName());
System.out.println(doctor.getAddress());
System.out.println(doctor.getGender());
System.out.println(doctor.getDepartment());
doctor.breathe();
}
}
Output :
XYZ
K Singh
Kanpur
Female
Dermatology
Man breathes.