Java packages and Imports
Java packages are used to club one or more similar types of classes and/or interfaces into one folder structure. This helps in clear segregation and maintenance of classes and interfaces.
A java package is nothing but a folder structure where classes and interfaces are stored.
Need of packages?
- If we don’t use packages, then maintaining our classes and interfaces will be very difficult.
- Every class name should be unique. No two classes or interfaces can have same name.
- To locate a particular class is also a difficult task if there are hundreds of classes in a project.
- There is no clear segregation of same kinds of classes.
Solution to all these problems is java packages.
How to define a package?
package com.javatrainingschool;
public class Tea {
//Elaichi Tea, Ginger Tea, Kesar Tea etc
private String name;
//Small, medium, large
private String size;
}
In the above example, we have defined a class named ‘Tea’ in a package ‘com.javatrainingschool’. If you look at the directory structure at your windows machine, you would see something like below

Here, we can see that under the src folder, according to the package naming ‘com.javatrainingschool’ , there are two folders are created : com -> javatrainingschool.
Note -> Folders are seperated with ‘.’ in the package name. Example, if package name is com.javatrainingschool.model, there will be three folders created, namely com -> javatrainingschool -> model
Important points about packages
- package naming convention is that all letters in name of a package should be small.
- package name can be as small as a single word such as ‘com’ and can also a big name containing many words, e.g. ‘com.javatrainingschool.model.data.popular’
- A package should be used to package similar kinds of classes/interfaces
- A package can have both, classes and interfaces
- A package name should be descriptive enough
- A package cannot have two classes or interfaces with the same name. But different packages in the same project can have classes and interfaces with the same name. Such classes are identified with their package names.
- Thus packages removes possibility of naming collision
Below is another diagram which shows two different packages : com.sks and com.javatrainingschool
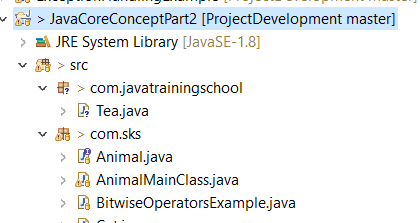
How to access classes in one package from classes in another package
This is a very common scenario where we need to access classes in one package from classes in another package. There are 3 ways of doing it as mentioned below:
Java Imports
- import <package-name>.*;
- import <package-name>.<class-name>;
- By use of fully classified class name inside the class e.g. com.javatrainingschool.Tea;
Let’s take the below examples to understand these concepts
package com.javatrainingschool;
import com.sks.*;
public class Tea {
private String name;
private String size;
public static void main(String[] args) {
Tea tea = new Tea();
Coffee coffee = new Coffee();
}
}
In the above example, we can see the import statement com.sks.*; It means we have imported all the classes which are there in the package com.sks. Since Coffee class lies in com.sks package, therefore it becomes available in this class ‘Tea’.
Note -> We don’t have to import the classes in the same package.
Note -> All java language api classes and interfaces are defined in some packages. E.g. java.lang.String, java.util.List
package com.javatrainingschool;
import com.sks.Coffee;
public class Tea {
private String name;
private String size;
public static void main(String[] args) {
Tea tea = new Tea();
Coffee coffee = new Coffee();
}
}
This is the same example as above. The only difference is the way we have imported Coffee class. Here, look at the import statement.
import com.sks.Coffee; This is another way in which we can directly import a particular class using its name.
Importing a class without using ‘import’ statement
package com.javatrainingschool;
public class Tea {
private String name;
private String size;
public static void main(String[] args) {
Tea tea = new Tea();
//Fully qualified class name
com.sks.Coffee coffee = new com.sks.Coffee();
}
}
In the above example, in stead of using the import statement, we have used the fully qualified class name ‘com.sks.Coffee’.
Subpackages
Let’s have a look at package names assuming each package has classes
- com.javatrainingschool
- com.javatrainingschool.model
- com.javatrainingschool.model.data
- com.javatrainingschool.model.data.popular
In the above example, if we consider com.javatrainingschool a package, then rest all packages that start with com.javatrainingschool. are subpackages of com.javatrainingschool.
Similarly all the packages that start with com.javatrainingschool.model. are subpackages of com.javatrainingschool.model package
Note -> subpackage is relative to a package
Static Import
Static imports are used to import static data members and methods of a class. Let’s have a look at the below example.
package com.javatrainingschool;
import static com.sks.Coffee.colour;
public class Tea {
private String name;
private String size;
public static void main(String[] args) {
Tea tea = new Tea();
String coffeeColour = colour;
}
}
Note -> java.lang package is imported by default. That is why all the classes, interfaces in that package can be accessed directly in our classes. such as String, StringBuffer, Thread, Runnable, System, NullPointerException, Integer etc.