Method Overloading
Method overloading is a concept in which a class has more than one methods with same name but different signature.
- Different signature means either different number of parameters.
- Or if number of parameters are same, then types of parameters are different
- Return type is not part of a method signature, and therefore, it is not considered in method overloading.

Let’s understand this using below example
package com.javatrainingschool;
public class MethodOverloadingExample {
public void displayName () {
System.out.println("Welcome sir/madam.");
}
public void displayName (String name) {
System.out.println("Welcome " + name);
}
public void displayName (String name, String surName) {
System.out.println("Welcome " + name + " " + surName);
}
public void displayName (String name, int age) {
System.out.println("Welcome " + name + ". Although it's not good to display your age, but it is " + age);
}
public static void main(String[] args) {
MethodOverloadingExample obj = new MethodOverloadingExample();
obj.displayName();
obj.displayName("Ranchhoddas");
obj.displayName("Ranchhoddas", "Rancho");
obj.displayName("Ranchhoddas", 35);
}
}
Output :
Welcome sir/madam.
Welcome Ranchhoddas
Welcome Ranchhoddas Rancho
Welcome Ranchhoddas. Although it's not good to display your age, but it is 35
In the above example, we can see that there are four methods with same name i.e. displayName() but they have different signature.
First 3 of them have different number of parameters. Third and fourth methods have same number of parameters i.e. 2, but they different types of parameters. Therefore, this is an example of method overloading.
Method call resolution
It is important to note that since all overloaded methods have same name, how it is decided which method will be invoked when. The answer is pretty straight forward. The call resolution of methods happen based on the type of parameters.
So, when displayName() is called, it invokes displayName() method without any paratmeter.
When displayName(“Ranchhoddas”) is called, it invokes displayName(String name) method, which takes one String parameter.
When displayName(“Ranchhoddas”, “Rancho”) is called, it invokes displayName(String name, String surName) method, which takes two String parameters.
When displayName(“Ranchhoddas”, 35) is called, it invokes displayName(String name, int age) method, which takes one String parameter and one int parameter.
Quiz : Can you overload main method?
Answer : Yes, you can. But entry point of your program will always be public static void main(String [] args);
Quiz: Can constructors be overloaded?
Answer : Yes, since all the constructors of a class have same name, they are overloaded.
Overloading Example
In the below example, we are calculating areas of different shapes by using method overloading concept.
package com.sks.polymorphism;
public class Area {
//calcualte the area of a circle
public double calculateArea(int radius) {
return 3.14 * radius * radius;
}
//calcualte the area of a reactangle
public int calculateArea(int length, int breadth) {
return length * breadth;
}
//calculate the are of a square
public float calculateArea(float side) {
return side * side;
}
public static void main(String[] args) {
Area obj = new Area();
float sqArea = obj.calculateArea(4.0f);
double circleArea = obj.calculateArea(3);
int rectArea = obj.calculateArea(12, 4);
System.out.println("Circle area : " + circleArea);
System.out.println("Rectangle area : " + rectArea);
System.out.println("Square area : " + sqArea);
}
}
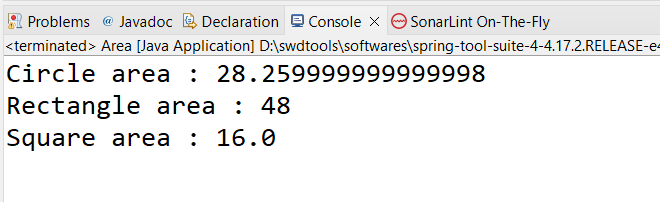