Cookies in Servlets
Cookies are small pieces of data generated and sent by the web server to the client. Cookies are sent in the response header and kept by the browser. Each web client can be assigned a unique session ID by a web server. Cookies are used to manage the ongoing user session. However, cookies can be turned off by the client.
Note1 -> The biggest drawback of using cookies is that if the client has turned off cookies, it will not work.
Note2 -> Cookies are maintained at client side
How cookie works
Due to stateless nature of http, each request from the same user is also considered as a new request. In session tracking using cookies technique, The server adds a cookie with the response that is sent to the client.
Cookie information is stored in the cache of the browser. Now, if a request is sent by the same user, cookie is added with request by default. Thus the server recognizes the request coming from the same user and the session is maintained.
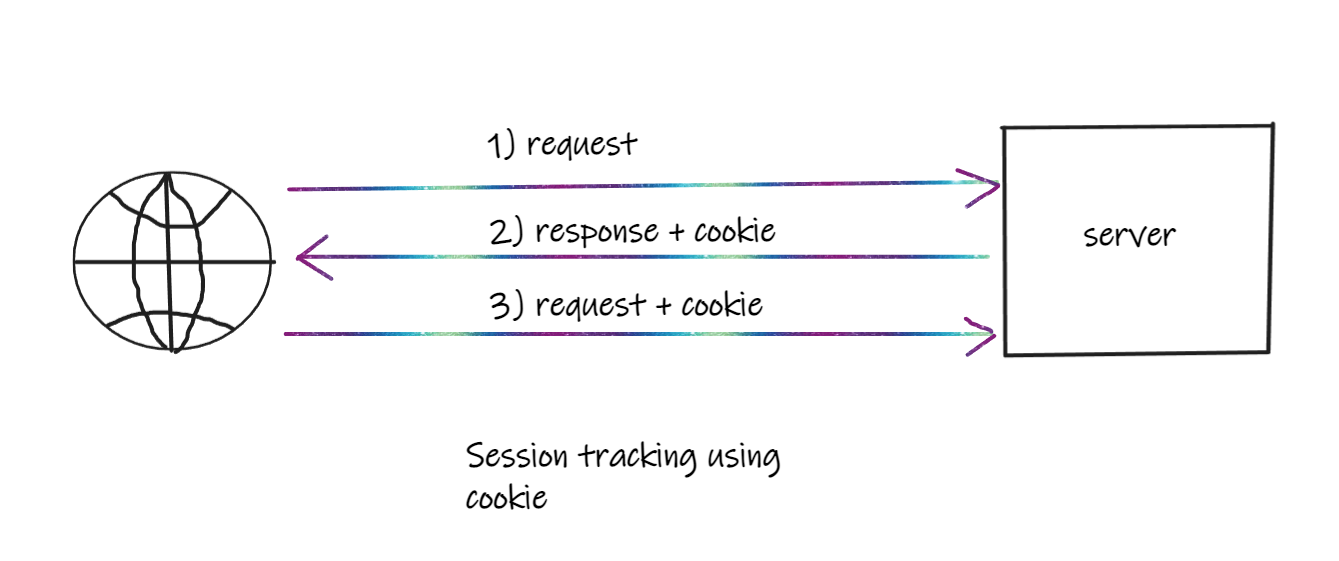
Cookie types
There are two types of cookies:
- Persistent – It is valid for multiple sessions. It is not removed from the browser each time when user closes the browser. It is removed only if user logs out or signs out.
- Non-persistent – It is valid for single session only. It is removed each time when user closes the browser.
Constructors
Cookie()
Cookie(String name, String value)
Commonly used methods of Cookie class
Method | Description |
---|---|
public String getName() | Gets the name of the cookie. |
public String getValue() | Get the value of the cookie |
public void setName(String name) | Sets the name |
public void setValue(String value) | Sets the value |
public void setMaxAge(int expiry) | Sets the max age of the cookie in seconds |
How to create cookie object
Cookie ck = new Cookie("user","Saras Singh");
response.addCookie(ck); //adding cookie in the response object
Cookie Example
cookiesExample.html
<html>
<form action="servletOne" method="post">
Name:<input type="text" name="userName" /><br><br>
<input type="submit" value="Test Cookies" />
</form>
</html>
ServletOne.java
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class ServletOne extends HttpServlet {
public void doPost(HttpServletRequest request, HttpServletResponse response) {
try {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
String userName = request.getParameter("userName");
out.print("Welcome " + userName);
Cookie ck = new Cookie("uname", userName);
response.addCookie(ck); // adding cookie in the response
RequestDispatcher rd = request.getRequestDispatcher("servletTwo");
rd.forward(request, response);
out.close();
} catch (Exception e) {
System.out.println(e);
}
}
}
ServletTwo.java
import java.io.PrintWriter;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ServletTwo extends HttpServlet {
public void doPost(HttpServletRequest request, HttpServletResponse response) {
try {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
Cookie[] cookies = request.getCookies();
out.print("Hello " + cookies[0].getValue());
out.close();
} catch (Exception ex) {
System.out.println(ex);
}
}
}
web.xml
<web-app>
<servlet>
<servlet-class>ServletOne</servlet-class>
<servlet-name>servletOne</servlet-name>
</servlet>
<servlet-mapping>
<servlet-name>servletOne</servlet-name>
<url-pattern>/servletOne</url-pattern>
</servlet-mapping>
<servlet>
<servlet-class>ServletTwo</servlet-class>
<servlet-name>servletTwo</servlet-name>
</servlet>
<servlet-mapping>
<servlet-name>servletTwo</servlet-name>
<url-pattern>/servletTwo</url-pattern>
</servlet-mapping>
</web-app>
Deploy, run and test the application
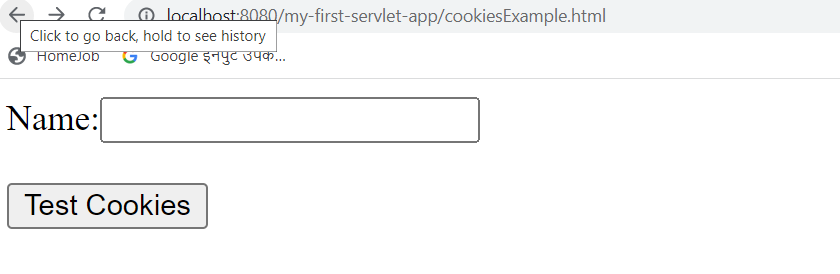
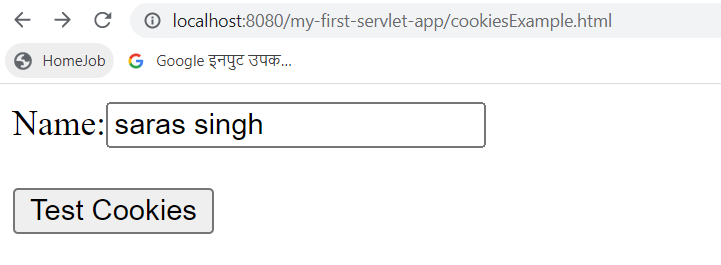
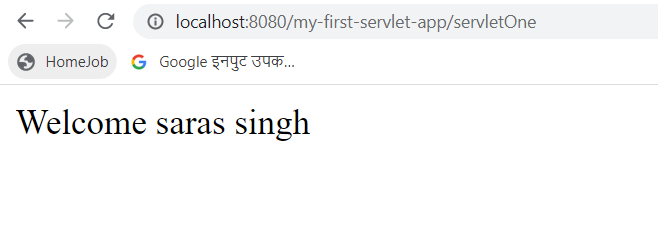