How to solve diamond problem with java 8 default method
Because of diamond problem, java doesn’t allow multiple inheritance via class. Meaning, one class cannot extend multiple classes at the same time. Let’s see what diamond problem is by looking at below diagram (Assuming multiple inheritance was allowed via classes in java)
- One interface called Screen has an abstract method called display()
- Two classes implement Screen interface and provide their own definition of display() method.
- One more class extends both of the above classes. Implicitly, both overridden methods will be available in this child class. Now, if tries to call display() method using its own instance, JVM confuses which method to call.
- This is known as Diamond problem. And because of this multiple inheritance via classes was forbidden in java.
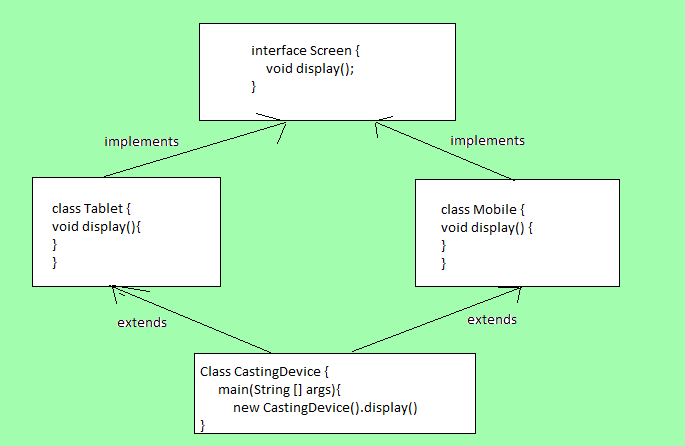
Default method in interface and diamond problem
Now, with introduction of default methods in java 8, the same diamond problem would have arisen, but java8 has handled it. Let’s see one example
package com.javatrainingschool;
public interface ScreenTouch {
default void display(){
System.out.println("This is screen touch input.");
}
}
package com.javatrainingschool;
public interface KeypadTouch {
default void display(){
System.out.println("This is keypad input.");
}
}
package com.javatrainingschool;
public class Laptop implements ScreenTouch, KeypadTouch {
//We must override the default method in this case
public void display(){
System.out.println("This is Laptop input.");
}
public static void main(String [] args){
Laptop laptop = new Laptop();
laptop.display();
}
}
Output :
This is Laptop input.