Arrays in Java
An array in java is a type of linear data structure which stores elements in continuous memeory locations. There are few points which are important when we talk about arrays in java.
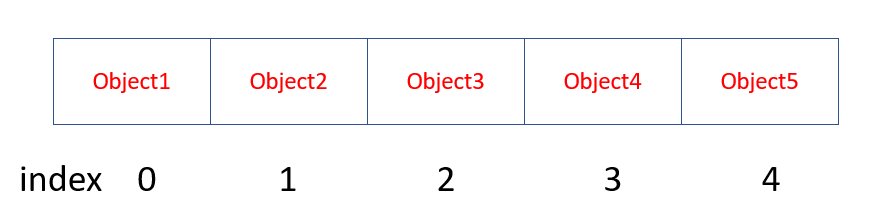
- Array stores data in continuous memory locations
- Array can store primitive data type variables as well as objects
- Arrays are fixed in size. Once declared, their size can’t be altered.
- Arrays store similar data types/objects
- Memory locations are called indexes
- We can retrieve or manipulate data at any index in an array
How to declare an array
Syntax:
<data-type> [] <var-name> = new <data-type> [<size-of-the-array>];
or,
<data-type> <var-name> [] = new <data-type> [<size-of-the-array>];
Below are few examples:
int [] intArray = new int [3]; //this is an integer array which can store upto 3 elements
char [] charArray = new char [5];
String [] strArray = new String [6];
Doctor doctors [] = new Doctor [4]; //this is an array of doctors where Doctor is a custom class
Initializing an array
We can also give initial values to the array at the time of declaring them. Let’s see how
Note -> Please note that while initializing an array upfront, you don’t have to mention the size of the array.
package com.javatrainingschool;
public class ArrayExample {
public static void main(String[] args) {
int [] intArr = new int [] {10, 11, 12, 54};
String [] strArr = new String [] {"Meera", "Kabir", "Ravidas"};
}
}
Array indexes
Array is an indexed based collection of elements. It means, elements are stored in increasing number of indexes. The first index starts with ‘0’. Which means the first element goes at index number 0. Second element goes at index 1, third at index 2, and nth element goes at index (n-1).
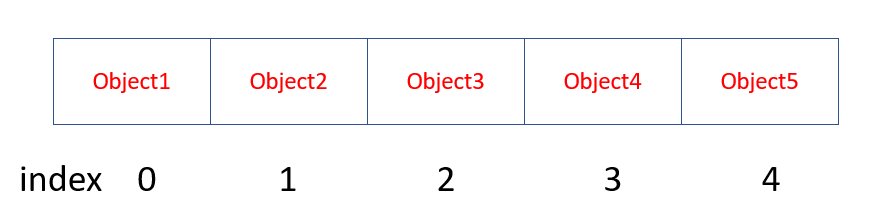
Adding elements to an array
package com.javatrainingschool;
public class ArrayExample {
public static void main(String[] args) {
int [] intArr = new int [4];
intArr[0] = 10;
intArr[1] = 20;
intArr[2] = 30;
intArr[3] = 40;
String strArr [] = new String [3];
strArr[0] = "Arjun";
strArr[1] = "Shankar";
strArr[2] = "Javed";
}
}
Adding a custom object to an array
We have a class called Beverage. We will add objects of this class to an array.
package com.javatrainingschool;
public class Beverage {
private int id;
private String name;
private String type;
public Beverage(int id, String name, String type) {
super();
this.id = id;
this.name = name;
this.type = type;
}
}
package com.javatrainingschool;
public class ArrayExample {
public static void main(String[] args) {
Beverage [] beverages = new Beverage [3];
Beverage softDrink = new Beverage(1, "Soft drink", "Cold");
Beverage coffee = new Beverage(2, "Coffee", "Hot");
Beverage cocktail = new Beverage(3, "Cocktail", "Cold");
beverages[0] = softDrink;
beverages[1] = coffee;
beverages[2] = cocktail;
}
}
Getting the size of an array
There is a keyword ‘length’ which can be used to get the size of an array. Let’s see an example
package com.javatrainingschool;
public class ArrayExample {
public static void main(String[] args) {
int [] intArr = new int [] {12, 13, 14, 15, 16};
System.out.println("Size of the array = " + intArr.length);
}
}
Output :
Size of the array = 5
Retrieving the elements of an array
Retrieval can be done in two ways:
- Using indexes
- Using for-each loop
Using indexes :
package com.javatrainingschool;
public class ArrayExample {
public static void main(String[] args) {
int [] intArr = new int [] {12, 13, 14, 15, 16};
int firstVal = intArr[0];
int valAtIndexTwo = intArr[2];
System.out.println("First element in the array : " + firstVal);
System.out.println("Element at index two in the array : " + valAtIndexTwo);
}
}
Output :
First element in the array : 12
Element at index two in the array : 14
Using for-each loop :
package com.javatrainingschool;
public class ArrayExample {
public static void main(String[] args) {
int [] intArr = new int [] {12, 13, 14, 15, 16};
int index = 0;
for(int value : intArr) {
System.out.println("Value at index " + index + " : " + value);
index++;
}
}
}
Output :
Value at index 0 : 12
Value at index 1 : 13
Value at index 2 : 14
Value at index 3 : 15
Value at index 4 : 16
ArrayIndexOutOfBoundsException
If, mistakenly, someone tries to fetch the value from an idex which is greater than the size of the array, then an exception called ‘ArrayindexOutOfBoundsException’ is thrown. For example, if size of an array is 4, and someone tries to fetch value at index 4 or of greater value, then exception will be thrown. Let’s understand this with an example.
package com.javatrainingschool;
public class ArrayExample {
public static void main(String[] args) {
int [] intArr = new int [] {12, 13, 14, 15, 16};
System.out.println("Size of the array : " + intArr.length);
int valAtIndexFive = intArr[5]; //intArr has max index value as 4. This will cause exception
System.out.println("Value of index five = " + valAtIndexFive);
}
}
Output :
Size of the array : 5
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 5
at com.sks.ArrayExample.main(ArrayExample.java:12)
Multidimensional arrays
In multidimensional arrays, data is stored as a matrix, in the form of rows and columns. So, in order to locate an element, we have to provide both row number and column number.
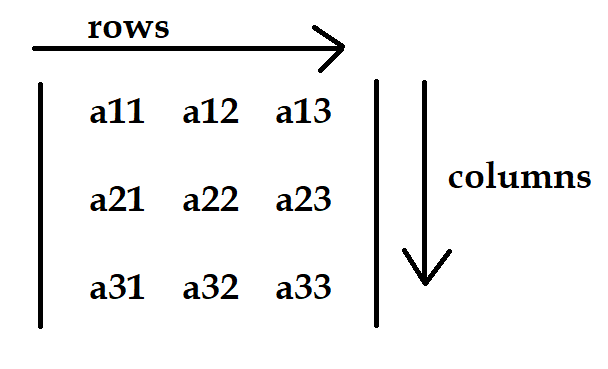
Note -> Multidimensional arrays can be visualized as arrays within an array. It can be understood by the below diagram.
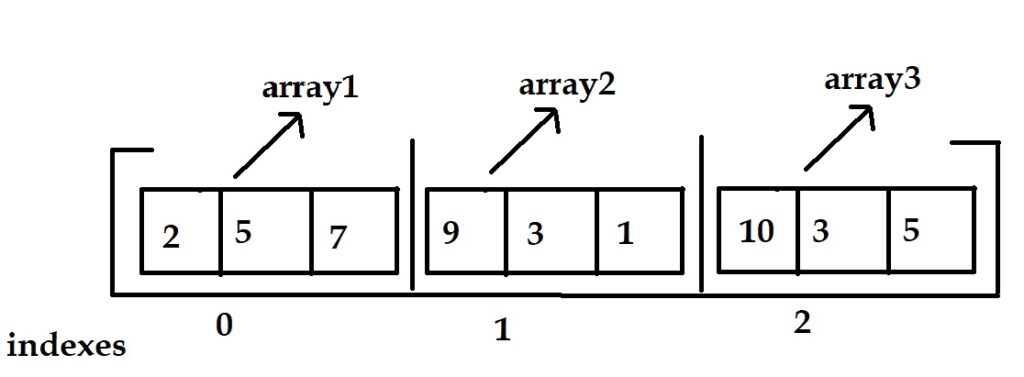
Syntax to define a multidimensional array :
int [][] twoDArray = new int[2][3]; //This array has two rows and three columns
String [][] strMultiDArray = new String[3][2]; //This array has three rows and two columns
Initializing a multidimensional array
package com.sks;
public class ArrayExample {
public static void main(String[] args) {
//This array has three rows and three columns
int [][] intArr = new int [] []{{12, 13, 14}, {15, 16, 17}, {18, 19, 20}};
}
}
package com.sks;
public class ArrayExample {
public static void main(String[] args) {
int [][] intArr = new int [2] [3];
intArr[0][0] = 10;
intArr[0][1] = 20;
intArr[0][2] = 30;
intArr[1][0] = 40;
intArr[1][1] = 50;
intArr[1][2] = 60;
System.out.println(intArr[0][2]);
System.out.println(intArr[1][0]);
}
}
Output :
30
40
Retrieving the elements from multidimensional array
The below code prints the array in matrix form using nested for-each loop
package com.sks;
public class ArrayExample {
public static void main(String[] args) {
int [][] intArr = new int [3] [3];
intArr[0][0] = 10;
intArr[0][1] = 20;
intArr[0][2] = 30;
intArr[1][0] = 40;
intArr[1][1] = 50;
intArr[1][2] = 60;
intArr[2][0] = 70;
intArr[2][1] = 80;
intArr[2][2] = 90;
for(int[] arr : intArr) {
System.out.print("|");
for(int val : arr) {
System.out.print(val + " ");
}
System.out.print("|");
System.out.println();
}
}
}
Output :
|10 20 30 |
|40 50 60 |
|70 80 90 |
Jagged array
Jagged array is a multidimensional array in which member arrays are of different sizes. It means, every row contains a different number of columns. Below is an example
package com.sks;
public class JaggedArrayExample {
public static void main(String[] args) {
int[][] twoDArray = new int[2][];
twoDArray[0] = new int[3]; //first row contains three columns
twoDArray[1] = new int[4]; //second row contains four columns
int value = 1;
for (int row = 0; row < twoDArray.length; row++) {
for (int col = 0; col < twoDArray[row].length; col++) {
twoDArray[row][col] = value++;
}
}
//print values in the array
for (int row = 0; row < twoDArray.length; row++) {
System.out.print("|");
for (int col = 0; col < twoDArray[row].length; col++) {
System.out.print(twoDArray[row][col] + " ");
}
System.out.println("|");
}
}
}