How to stop a thread
Earlier, static method Thread.stop() was used to stop a thread, but that led to a lot of complexities, and therefore it was deprecated later. Not only that but methods like Thread.suspend() and Thread.resume() were also deprecated after java 1.1. Now, if we cannot use these methods, then how to stop a thread.
Stop a thread using a flag
Using a flag, we can stop a thread. As long as, that flag is true, the thread will keep on running. The moment, it becomes false, the thread will stop. Let’s see the example
package com.sks;
import java.util.concurrent.atomic.AtomicBoolean;
public class StoppingThreadExample implements Runnable {
private Thread worker;
private final AtomicBoolean shouldRun = new AtomicBoolean(false);
public void run() {
shouldRun.set(true);
while(shouldRun.get()) {
System.out.println("Thread is running");
}
System.out.println("The thread is stopped");
}
public void start() {
worker = new Thread(this);
worker.start();
}
public void stop() {
shouldRun.set(false);
}
public static void main(String[] args) {
StoppingThreadExample t1 = new StoppingThreadExample();
t1.start();
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
t1.stop();
}
}
Output:
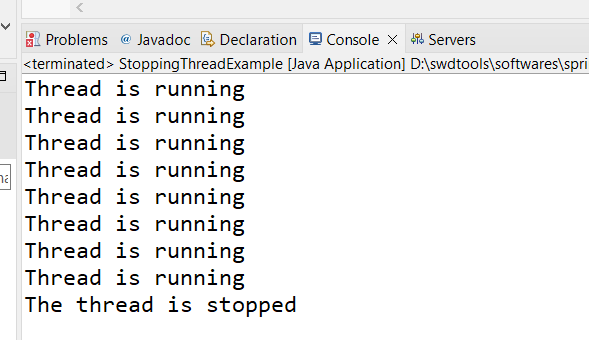