Object Cloning In Java
In Java, cloning refers to the process of creating a new object with the same state as an existing object. This process is commonly used when you want to create a new object that is similar to an existing object, without having to go through the process of initializing the new object’s properties one by one.
To clone an object in Java, the class of the object being cloned must implement the Cloneable interface and override the clone() method, which is declared as protected in the Object class. The clone() method is used to create a new instance of the object with the same values as the original object.
Creating Copy of Java Object
We can create a replica or copy of java object by
- Creating a copy of object in a different memory location. This is called a Deep copy.
- Creating a new reference that points to the same memory location. This is also called a Shallow copy.
Types of Object Cloning
- Shallow Cloning
- Deep Cloning
Shallow Cloning
Shallow cloning is the default type of cloning that is provided by Java. When an object is shallow cloned, a new object is created with the same fields as the original object. However, any object references within the object being cloned will be copied by reference and not by value. This means that the new object will contain references to the same objects as the original object. Therefore, if any changes are made to the referenced objects, those changes will be reflected in both the original and cloned objects.
To perform shallow cloning in Java, you simply need to implement the Cloneable interface and override the clone() method. The clone() method will return a new instance of the object with the same field values as the original object.
package com.javatrainingschool;
class Department {
String id;
String designation;
public Department(String empId, String desc) {
this.id = empId;
this.designation = desc;
}
}
Employee.java
package com.javatrainingschool;
class Employee implements Cloneable {
int id;
String name;
String bloodGroup;
Department dept;
public Employee(int id, String name, String BG, Department dept) {
this.id = id;
this.name = name;
this.bloodGroup = BG;
this.dept = dept;
}
// Default version of clone() method. It creates shallow copy of an object.
protected Object clone() throws CloneNotSupportedException {
return super.clone();
}
}
ShallowCopyInJava.java
package com.javatrainingschool;
public class ShallowCopyInJava {
public static void main(String[] args) {
Department dept1 = new Department("A1", "Cloud");
Employee e1 = new Employee(7012, "Manu","AB+", dept1);
Employee e2 = null;
try {
// Creating a clone of e1 and assigning it to e2
e2 = (Employee) e1.clone();
} catch (CloneNotSupportedException e) {
e.printStackTrace();
}
// Printing the designation of 'e1'
System.out.println(e1.dept.designation); // Output : Cloud
// Changing the designation of 'emp2'
e2.dept.designation = "Testing";
// This change will be reflected in original Employee 'e1'
System.out.println(e1.dept.designation); // Output : Testing
}
}
Output:
Cloud
Testing
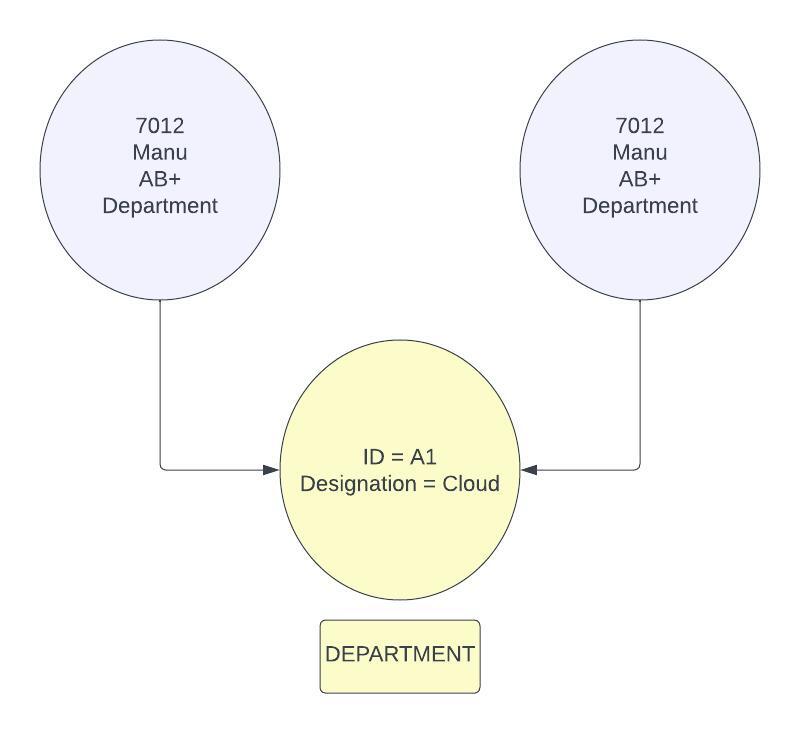
Deep Cloning
Deep cloning is a type of cloning where a new object is created with the same field values as the original object, but all object references within the object being cloned are also cloned, creating a completely new set of objects. This means that any changes made to the cloned object or its referenced objects will not affect the original object.
To perform deep cloning in Java, you need to implement custom cloning logic that traverses and clones all object references within the object being cloned.
Department.java
package com.javatrainingschool;
class Department implements Cloneable {
String id;
String designation;
public Department(String empId, String desc) {
this.id = empId;
this.designation = desc;
}
protected Object clone() throws CloneNotSupportedException {
return super.clone();
}
}
Employee.java
package com.javatrainingschool;
class Employee implements Cloneable {
int id;
String name;
String bloodGroup;
Department dept;
public Employee(int id, String name, String BG, Department dept) {
this.id = id;
this.name = name;
this.bloodGroup = BG;
this.dept = dept;
}
// Default version of clone() method. It creates shallow copy of an object.
protected Object clone() throws CloneNotSupportedException {
Employee emp1= (Employee) super.clone();
emp1.dept= (Department) dept.clone();
return emp1;
}
}
DeepCopyInJava.java
package com.javatrainingschool;
public class DeepCopyInJava {
public static void main(String[] args) {
Department dept1 = new Department("A1", "Cloud");
Employee e1 = new Employee(7012, "Manu","AB+", dept1);
Employee e2 = null;
try {
// Creating a clone of e1 and assigning it to e2
e2 = (Employee) e1.clone();
} catch (CloneNotSupportedException e) {
e.printStackTrace();
}
// Printing the designation of 'e1'
System.out.println(e1.dept.designation); // Output : Cloud
// Changing the designation of 'e2'
e2.dept.designation = "Testing";
// This change will be reflected in original Employee 'e1'
System.out.println(e1.dept.designation); // Output : Cloud
}
}
Output:
Cloud
Cloud
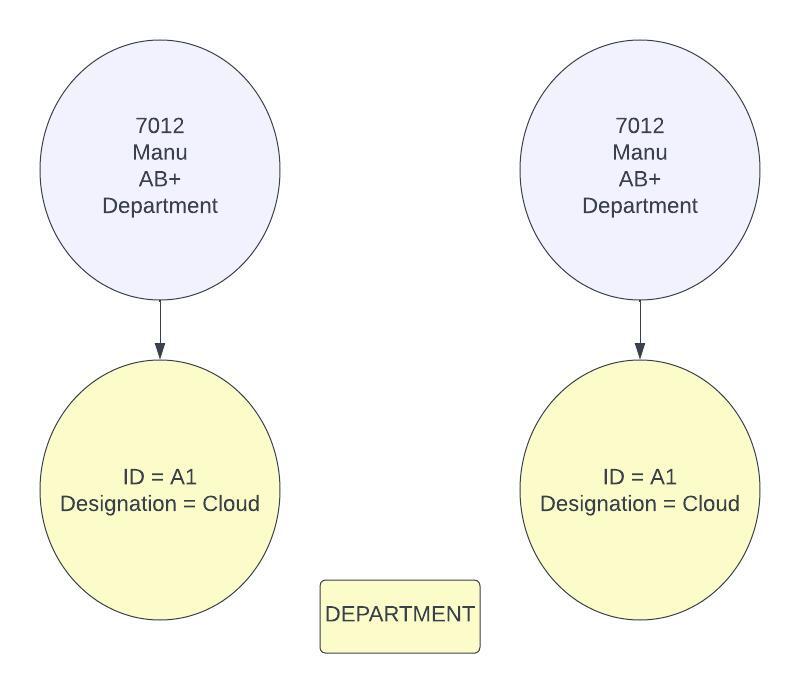
NOTE:
The clone method in Object class is protected in nature, so not all classes can use the clone() method. You need to implement Cloneable interface and override the clone method. If the Cloneable interface is not implemented then you will get CloneNotSupportedException.super.clone () will return shallow copy as per implementation in Object class.
Difference between Shallow Cloning and Deep Cloning
Shallow Cloning | Deep Cloning |
The cloned object and the source object are not entirely separate from each other. | Cloned objects and source objects are completely independent of each other. |
Modifications made to the cloned object will affect the reference variable of the source object. | Modifications made to the cloned object will not affect the reference variable of the source object. |
By default, the clone method in Java performs a shallow copy. | To create a deep copy, it is necessary to override the clone() method of the Object class and implement custom cloning logic that traverses and clones all object references within the object being cloned. |
Shallow copy is a good option if the class variables of the object are only primitive types as fields. | Deep copy is a preferable option when the class variables of the object have references to other objects as fields. |
It is relatively fast to perform shallow copying. | Deep copying is relatively slow compared to shallow copying, especially when the object being cloned contains a large number of object references or complex data structures. |