Variables and Constants in Java
Variables
Variables are used to store different types of values which can be changed in the program. The data type of variable defines what kind of value a variable will store. The syntax to define a variable is:
<access-modifier> <data-type> <name-of-the-variable> = <value>;
Example of variables
private int number = 10;
private String name = “Arjun”;
public boolean isSuccess = false;
protected char = ‘n’;
float decimalNumber = 1231.1f;
Access Modifier
Access modifier decides the scope of variables. There are four access modifier/specifier : private, protected, public, default( or no access modifier).
Private Variables – are accessible only within the class.
Protected Variables – are accessible in all the child classes whether in the same package or different package; and in all the classes in the same package.
Public Variables – are accessible in all the classes. They are accessible from everywhere.
Default Variables – are accessible in all the classes in the same package.
Types of Variables
Variables have been divided into three categories : Instance variables, Static variables, Local variables.
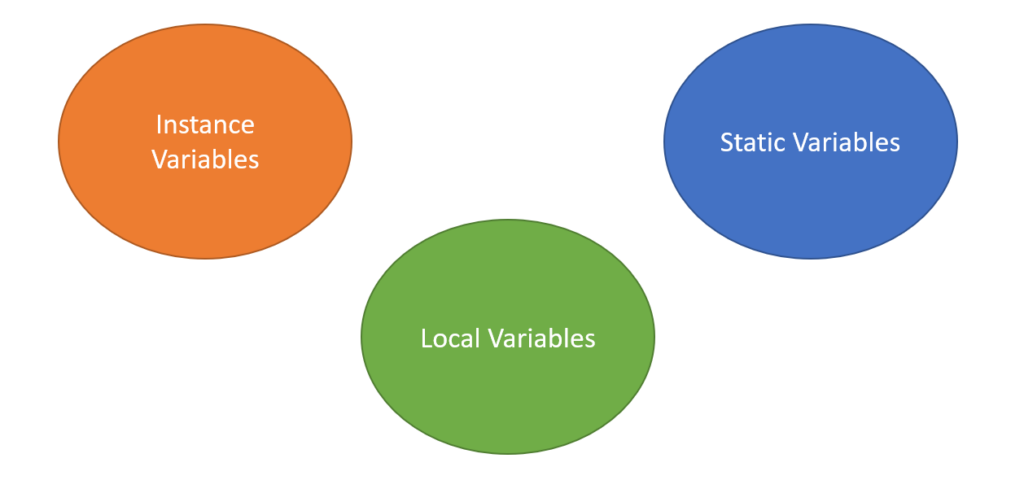
Instance Variables
Instance variables are associated with instances/objects of the class. Every object has its own copy of instance variables. One object’s values will be specific to that object. Let’s take one example
package com.sks;
public class Cricketer {
private String name;
private int age;
private String type;
public static void main(String[] args) {
Cricketer cricketer1 = new Cricketer();
cricketer1.name = "Sachin Tendulkar";
cricketer1.age = 35;
cricketer1.type = "Batsman";
Cricketer cricketer2 = new Cricketer();
cricketer2.name = "Shane Warne";
cricketer2.age = 38;
cricketer2.type = "Bowler";
System.out.println("Cricketer 1 name : " + cricketer1.name + ", age : " + cricketer1.age + ", type : " + cricketer1.type);
System.out.println("Cricketer 2 name : " + cricketer2.name + ", age : " + cricketer2.age + ", type : " + cricketer2.type);
}
}
Output :
Cricketer 1 name : Sachin Tendulkar, age : 35, type : Batsman
Cricketer 2 name : Shane Warne, age : 38, type : Bowler
In the above example, Cricketer class has 3 instance variables : name, age and type. We have created two objects of Cricketer class. Each cricketer object has own values of its instance variables.
Static or Class Variables
Static or Class variables are associated with the class itself, which means their values will be common for all the objects of that class. This is the reason static variables are also called Class variables. Such variables are accessed using name of the class. In order to declare static variables, we have to use static keyword after the access modifier.
Syntax for declaring static variables :
public static String sportName;
package com.sks;
public class Cricketer {
private String name;
private int age;
private String type;
private static String sportName; //This is static or class variable
public static void main(String[] args) {
Cricketer.sportName = "Cricket"; //set only once and it is available to all the objects
Cricketer cricketer1 = new Cricketer();
cricketer1.name = "Sachin Tendulkar";
cricketer1.age = 35;
cricketer1.type = "Batsman";
Cricketer cricketer2 = new Cricketer();
cricketer2.name = "Shane Warne";
cricketer2.age = 38;
cricketer2.type = "Bowler";
System.out.println("Cricketer 1 name : " + cricketer1.name + ", age : " + cricketer1.age + ", type : " + cricketer1.type + ", sportName : " + Cricketer.sportName);
System.out.println("Cricketer 2 name : " + cricketer2.name + ", age : " + cricketer2.age + ", type : " + cricketer2.type + ", sportName : " + Cricketer.sportName);
}
}
Output :
Cricketer 1 name : Sachin Tendulkar, age : 35, type : Batsman, sportName : Cricket
Cricketer 2 name : Shane Warne, age : 38, type : Bowler, sportName : Cricket
Local Variables
Local variables are the ones that we define inside a method or a block. The scope of these variables are limited to the method they are defined in, which means they cannot be accessed outside the method.
Note -> ‘static’ keyword cannot be used with local variables.
package com.sks;
import java.util.Random;
public class Cricketer {
private String name;
private int age;
private String type;
private static String sportName;
public int assignJerseyNumber() {
Random random = new Random(); //random is a local variable to this method
int jerseyNumber = random.nextInt(); //jerseyNumber is a local variable to this method
return jerseyNumber;
}
}
Constants
Constants are those attributes of a class whose value doesn’t change. Real world examples of constants will be – a woman/man has 2 legs. So, if we are creating woman or man class, then we can define an attribute with a constant value of 2. Similarly, if we are defining a Triangle class, we can keep an attribute with names ‘noOfSides’ with value 3. Because that is going to remain constant. So, any value that is going to be common for all the objects of that class, we should make it a constant.
public class Triangle {
private static final int noOfSides = 3;
public static void main (String [] args) {
System.out.println("No of sides of a triangle are always : " + Triangle.noOfSides);
}
}
In the above example, please note that we have made the constant static. The reason for that is since this value is going to remain the same for all the objects of the class, it is wise to make it static property of the class. Below are some important points about constants in Java.
- Constants must be declared static because their value is supposed to be same for all the objects of the class.
- It is mandatory to make them final by the use of keyword ‘final’. Final means once assigned, its value cannot be changed throughout the program.
- Right at the time of declaration of a constant, it must be initialized. e.g. public static final int number = 100;
- If we don’t initialize a constant at the time of declaration, then there is another way to do so. And that is, it must be initialized inside a static block.
public class Triangle {
private static final int noOfSides;
static {
noOfSides = 3;
}
public static void main (String [] args) {
System.out.println("No of sides of a triangle are always : " + Triangle.noOfSides);
}
}
5. Constants can be categorized as per the below diagram.
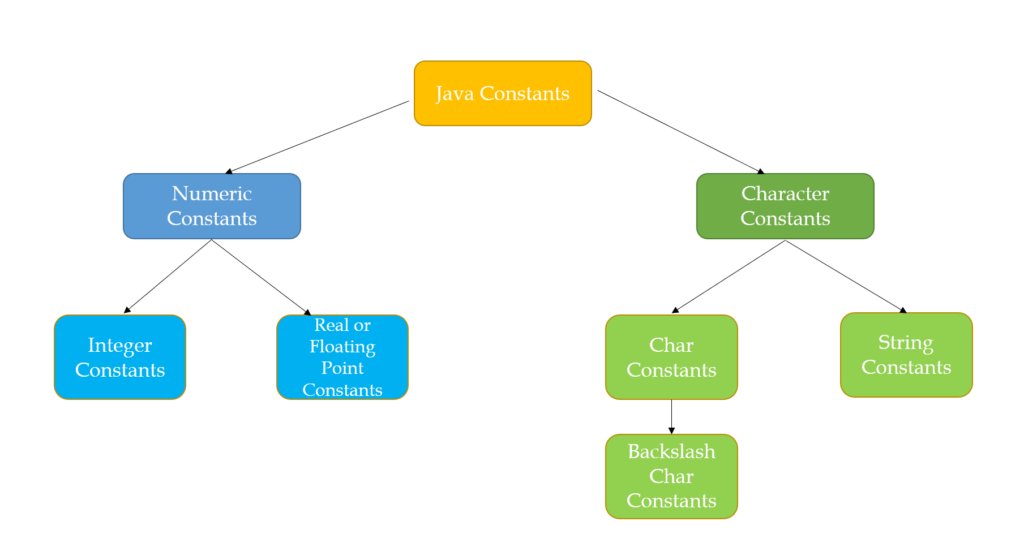
Examples of constants :
public static final String str = “abc”;
public static final float num = 1231.12f;
public static final char c = ‘A’;
Examples of backslash constants :
\r Carriage return
\b Backspace
\n New line
\f Form feed
\\ Backslash
\t Horizontal tab
\” Double quote
\’ Single quote
\v Vertical tab
\? Question mark
\a Alert
\xN Hexadecimal constant
\N Octal constant