Start and Run method
In the previous examples, we have seen two methods in use.
public void run();
t.start(); //where t is a thread
Let’s understand these methods in detail.
Run method
public void run() { };
This abstract method is declared in Runnable interface. Since Thread class implements Runnable interface, this method is available in Thread class also.
When we create threads, either by extending Thread class, or by implementing Runnable interface, we have to override this method.
This method’s business logic is run as a separate thread. If we create n threads, each thread will execute run() method’s logic in a separate call stack.
Please note that, we only have to override this method. This is not called directly in our class. But indirectly it is called, when we call start() method on a thread. But if someone calls this method explicitly, its logic is executed normally. It is not done in a separate thread.
Start method
Thread t = new UserThread(); //UserThread is a custom class that extends Thread class
t.start();
When we call start method on a thread, JVM starts a new call-stack for that thread and stores thread data in that. Now, the thread executes run method logic in newly created stack. This is why we call start method on threads rather than the run method.
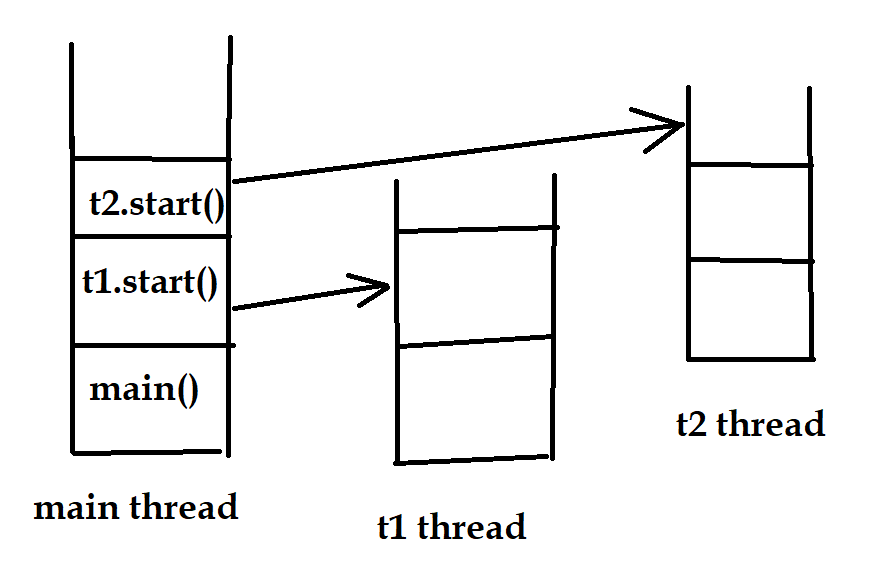
What if run method is called instead of start
The below diagram explains the situation that we have already talked about
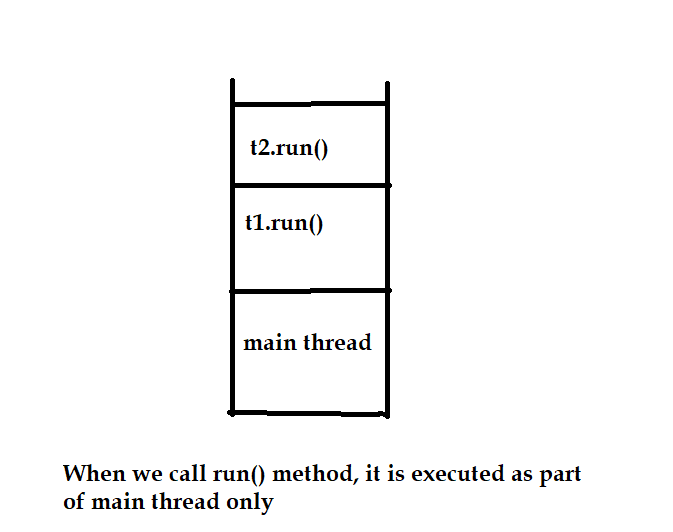
If run() method is called on a thread object rather than start() method, it will still execute run() method’s logic, but not in a separate thread. It will be executed as part of main thread only.
What happens if you call start() method twice on the same thread
We cannot do so. Because once a thread finishes its run method execution, it goes to dead state. And once in dead state, it cannot be brought back to life. So, if someone tries to call start() method twice on the same thread, java.lang.IllegalThreadStateException is thrown.