Strings are immutable
In java, Strings are immutable. Meaning, once created, their state/content cannot be changed.
String s1 = "Meera";
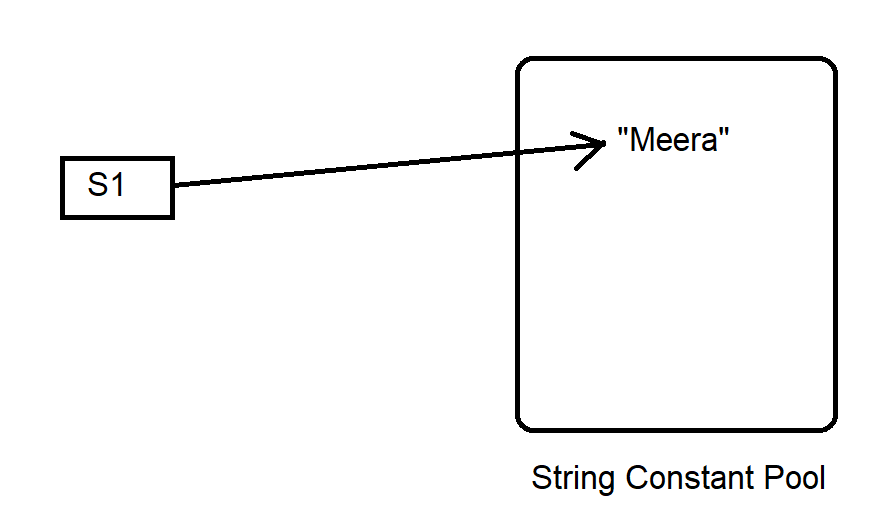
In the above statement, there are two things created: reference variable s1 and object with value as “Meera”. Since, String objects are immutable, it means the object with value “Meera” cannot be changed. However, s1 can point to another object. So, if we say
s1 = "Arjun";
Another object with value “Arjun” will be created, and s1 will start pointing to it. However, “Meera” will still be existing in the memory, but will be unreferenced. Let’s understand this using the below diagram
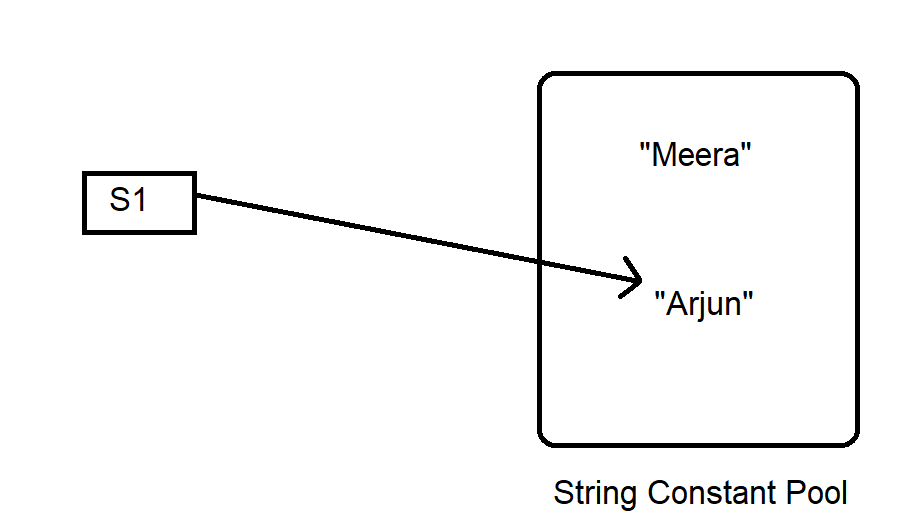
Thus, any operation done on a String object, actually creates a new object. And the reference variable starts pointing to the newly created object. Consider below example,
package com.javatrainingschool;
public class StringImmutabilityTest {
public static void main(String[] args) {
String s1 = "Meera";
System.out.println(s1);
s1 = s1.concat(" Kumar");
System.out.println(s1);
}
}
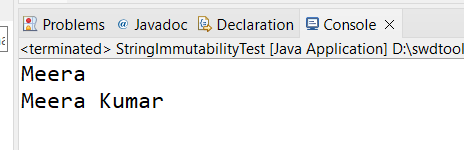
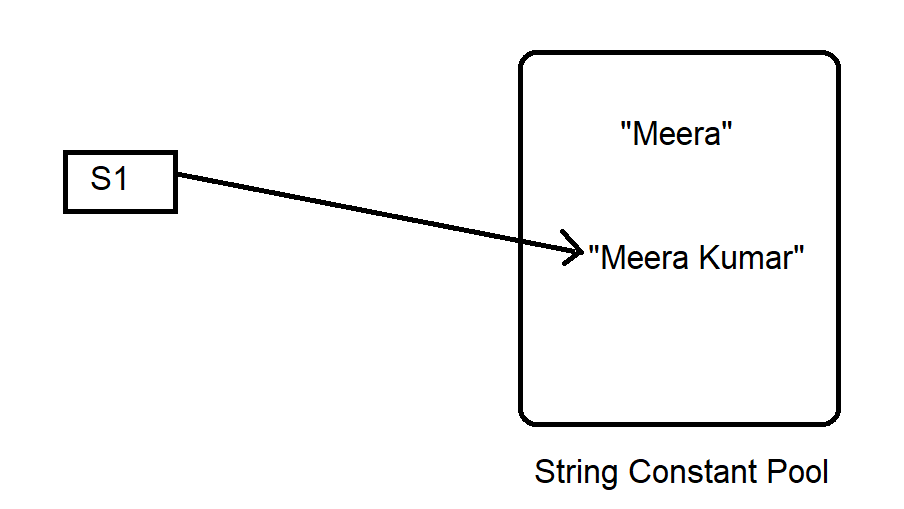
Why are Strings immutable in Java?
There are certain reasons because of which Strings are made immutable in java. Let’s understand those
1. Security
The Strings are used everywhere in java programming language. They are also used widely in Java applications to store sensitive information like usernames, passwords, connection URLs, network connections, etc. It’s also used extensively by JVM class loaders while loading classes.
Therefore it becomes important to secure String class regarding the security of the whole application in general. If Strings were mutable, it would have been difficult to safeguard them against unauthorized changes.
2. Synchronization
Strings immutability feature make them thread-safe and they can be used between multiple threads without need of any external synchronization.
3. HashCode Caching
Since String objects are used in data structures, they are also used in hash implementations like HashMap, HashTable, HashSet, etc. While working on these hash implementations, hashCode() method is called several times for finding bucket locations.
The immutability feature of String guarantees that their value won’t change.
4. Performance
String Constant Pool where String literals are stored keeps only unique copies of String objects. It saves a lot of HEAP space and thus enhances their performance. If Strings were mutable, this wouldn’t have been achieved.