Control Statements – If else
Control statements can change the flow of a program based on certain conditions. We can divide such statements in 3 categories:
- Decision making (based on condition(s)) statements such as
If/else, if-else if-else, switch, and ternary operator
2. Loops such as
while, do while, for, for-each
3. Branching or jump statements such as
break, continue
If-else statement
It is a combination of two statements i.e. ‘if’ and ‘else’. If the condition is true, then if block is executed otherwise else block. It is written in following way:
if(<some-condition>) {
//lines of code
//lines of code
}
else {
//lines of code
//lines of code
}
package com.javatrainingschool;
public class IfElseExample {
public static void main(String[] args) {
int num = 24;
if (num >= 20) {
System.out.println("The number greater than 20.");
System.out.println("If block executed.");
} else {
System.out.println("The number is less than 20");
System.out.println("Else block executed.");
}
}
}
Output :
The number greater than 20.
If block executed.
If, else if, else if…, else
This has multiple conditions. One associated with ‘If’ block and others associated with ‘else if’ blocks. All these blocks are mutually exclusive, meaning only one of them will get executed. It is written in following way:
if(<some-condition>) {
//lines of code
}
else if(<some-condition>) {
//lines of code
}
else if(<some-condition>) {
//lines of code
}
else {
//lines of code
}
In the above example, the control will only go to else if block if all the previous conditions are failed.
package com.javatrainingschool;
public class IfElseIfExample {
public static void main(String[] args) {
int num = 0;
if (num > 20) {
System.out.println("If block executed.");
System.out.println("The number is greater than 20.");
} else if (num == 20) {
System.out.println("First Else if block executed.");
System.out.println("The number is equal to 20.");
} else if (num != 0) {
System.out.println("Second else if block executed.");
System.out.println("The number is not equal to 0.");
} else {
System.out.println("Else block executed.");
System.out.println("The number is = " + num);
}
}
}
Output :
Else block executed.
The number is = 0
Switch Statement
Switch statement also control the flow of program. It is written in following way.
Please note that we have to use break statement in every case. If we don’t do so, all the cases after the matching case will be executed, including the default case.
switch (expression) {
case value1 :
//lines of code
//lines of code
break;
case value2 :
//lines of code
//lines of code
break;
case value3 :
//lines of code
//lines of code
break;
......
......
......
default :
//lines of code
//lines of code
}
package com.javatrainingschool;
public class SwitchExample {
public static void main(String[] args) {
String name = "Raidas";
switch(name) {
case "Meera" :
System.out.println("name is Meera.");
break;
case "Kabir" :
System.out.println("Name is Kabir");
break;
case "Raidas" :
System.out.println("Name is Raidas");
break;
default :
System.out.println("The default name is Ram");
}
}
}
Output:
Name is Raidas
Switch example without any break statement :
package com.javatrainingschool;
public class SwitchExample {
public static void main(String[] args) {
String name = "Kabir";
switch(name) {
case "Meera" :
System.out.println("name is Meera.");
case "Kabir" :
System.out.println("Name is Kabir");
case "Raidas" :
System.out.println("Name is Raidas");
default :
System.out.println("The default name is Ram");
}
}
}
Output :
Name is Kabir
Name is Raidas
The default name is Ram
Ternary Operator
It is symbolic representation of if-else block. ? : collectively known as ternary operator. It is written in the following way :
condition ? statement : statement
If the condition is true, the statement after ? will be executed, othewise, the condition after : will be executed.
package com.javatrainingschool;
public class TernaryOperatorExample {
public static void main(String[] args) {
int a = 10;
int b = 20;
int greaterNum = (a > b) ? a : b;
System.out.println("Greater number between " + a + " and " + b + " is : " + greaterNum);
}
}
Here, we have covered first set of control statements. Now, let’s not control our urge to have a cup of coffee. Let’s enjoy!
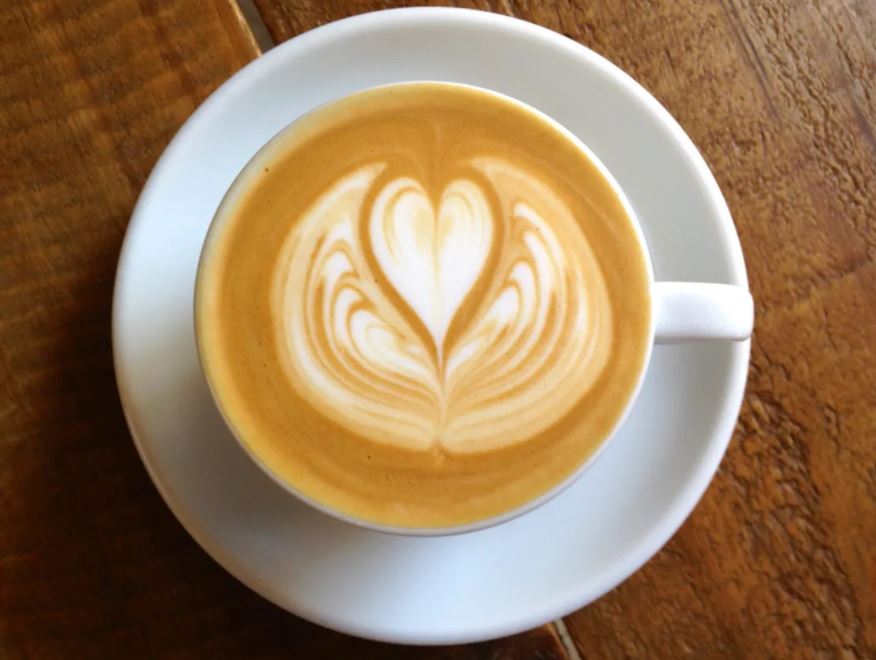