String Introduction
String class belongs to java.lang package. The objects of String class are most frequently used in java programming language. In fact, it is almost impossible to write an executable java program without a String object.
The main method which is the entry point of any java program also takes an array of String objects as a parameter.
public static void main (String [] args){ }
Strings are sequence of characters.
“Arjun”, “Meera”, “ABC123”, “DEF$” are examples of String objects.
Ways to create String objects
There are two ways of creating String objects
- Using String literals
- Using new keyword
Create String objects using String literals
Below are the examples of String objects of string literals. In this way, simply assign a text within the double quotes to a String variable.
String s1 = "Arjun";
String s2 = "Meera";
String s3 = "Newton";
String s4 = "Darwin";
In the below diagram, String “ARJUN” can be seen as array of characters.
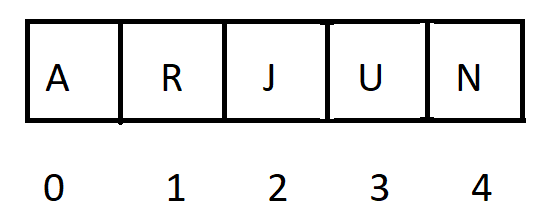
package com.sks;
public class StringExample {
public static void main(String[] args) {
String s1 = "Arjun";
String s2 = "Meera";
System.out.println("String s1 : " + s1);
System.out.println("String s2 : " + s2);
}
}
Output:
String s1 = "Arjun";
String s2 = "Meera";
Create String objects using new keyword
In this way, String objects are created using the new keyword like other java objects. Below are some examples
String s1 = new String("Kabir");
String s2 = new String("Shakuntala");
package com.sks;
public class StringExample {
public static void main(String[] args) {
String s1 = new String("Kabir");
String s2 = new String("Shakuntala");
System.out.println("String s1 : " + s1);
System.out.println("String s2 : " + s2);
}
}
Output:
String s1 : Kabir
String s2 : Shakuntala
Difference between String literal and object created using new keyword
We have seen the two ways of creating String objects. Now, the question arises what is the difference between the two.
The difference is the memory allocation for the objects created in the two ways.
The objects created as String literals (String s1 = “Arjun Kumar”) are created in a memory area called String Constant Pool. The interesting thing about String Constant Pool is that it doesn’t keep duplicate String objects.
Whereas the objects created using ‘new’ keyword are created in heap area of memory. And also, if there is no same object in the String Constant Pool, it also creates one in the String Constant Pool. So, there is a possibility of two objects creation in case of new keyword. Unlike String Constant Pool, heap area allows duplicate String objects.
A detailed analysis of String Constant Pool is done on the next page