Deque and ArrayDeque in Java
Deque is double ended queue. It means insertion and removal of elements can happen at both ends of the queue.
Declaration
public interface Deque<E> extends Queue<E>
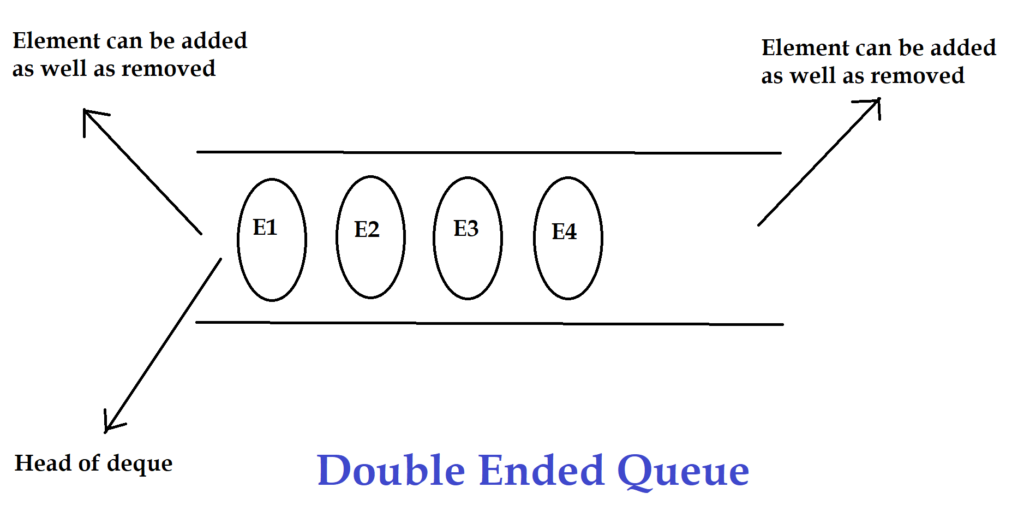
ArrayDeque
ArrayDeque is a class that implements Deque interface. Let’s see some imortant points about ArrayDeque.
- Using ArrayDeque, we can add and remove elements from both ends of it.
- It does not have any capacity restrictions. They can grow as required.
- Null elements are prohibited in ArrayDeque
- It is not thread safe.
- They are faster than linked list and stack.
Class Declaration
public class ArrayDeque extends AbstractCollection implements Deque, Cloneable, Serializable
ArrayDeque Example
package com.javatrainingschool;
import java.util.ArrayDeque;
import java.util.Deque;
public class ArrayDequeExample {
public static void main(String[] args) {
Deque<String> dq = new ArrayDeque<String>();
dq.add("Harry");
dq.add("Gurpreet");
dq.add("Ajay");
dq.add("Abrar");
dq.add("Neha");
dq.offerFirst("Manish");
dq.offerLast("Shahid");
while (dq.size() > 0) {
System.out.println(dq.pop());
}
}
}
Output :
Manish
Harry
Gurpreet
Ajay
Abrar
Neha
Shahid
offerFirst() and offerLast() methods
Using offerFirst() method, we can add an element at the beginning of the deque. whereas, Using offerLast() method, we can add an element at the end of the deque.
pop() method
pop() method is used to retrieve and remove the head element of the deque. It is method of Deque class.
pollFirst() and pollLast() methods
pollFirst and pollLast() methods are methods of Deque interface. These methods are used to retrieve and remove first and last elements in the deque.
package com.javatrainingschool;
import java.util.ArrayDeque;
import java.util.Deque;
public class ArrayDequeExample {
public static void main(String[] args) {
Deque<String> dq = new ArrayDeque<String>();
dq.add("Harry");
dq.add("Gurpreet");
dq.add("Ajay");
dq.add("Abrar");
dq.add("Neha");
dq.offerFirst("Manish");
dq.offerLast("Shahid");
System.out.println(dq.pollFirst());
System.out.println(dq.pollLast());
}
}
Output :
Manish
Shahid
Thus, we have seen that Deque supports insertion and removal of elements at the beginning and end of deque.