Constructors in Java
Constructors are parts of a class that are responsible for initializing attributes of an object upon construction.
In other words, the constructors are used to initialize the state of an object upon construction.
But wait, if the preceding statement is true why didn’t we see a constructor actually in a class so far. We have written quite a few classes but in none of them we saw or defined a constructor. Why so?
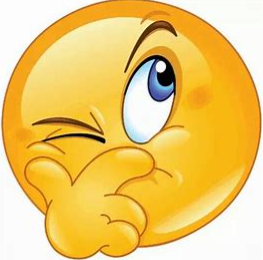
The reason for the above doubt is that, so far, we have been using the default constructor of the classes unknowingly. A default constructor is implicitly provided to the class which means we don’t have to write it explicitly, it is already there with the class. When JVM creates the objects of that class it uses the default constructor.
How does a default constructor look like?
Let’s have a look at the below statement which we have been using to create an object of a class.
Coffee coffee = new Coffee();
Here we are creating an object of Coffee class using the new operator. Let’s understand the meaning of each and every word in this statement.
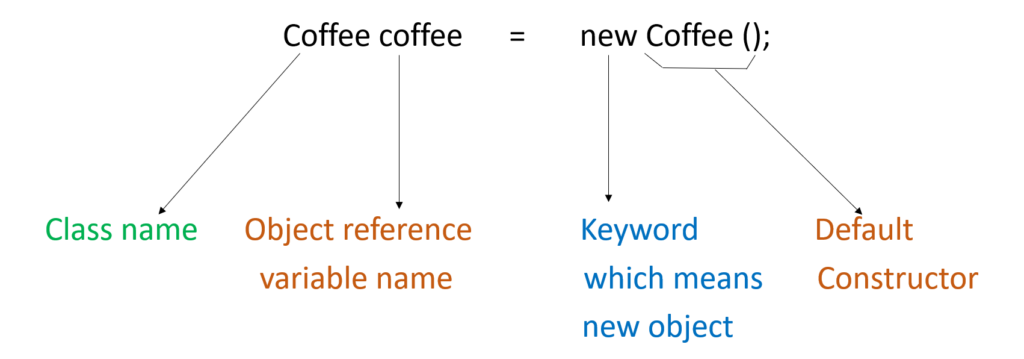
“Coffee ()” – this is called the default constructor of the class. As said before, we don’t have to explicitly define this, because it is already available. But if you still want to write it in your class, then also there is no problem. Let’s see how we can write a default constructor in a class.
public class Coffee {
String name;
String colour;
String type;
//Default constructor
public Coffee() {
}
}
Note -> that default constructor is also known as no-argument constructor.
We can also write some code in the default constructor. Like below
public Coffee() {
System.out.println ("Coffee object constructed successfully");
}
Some important points about constructors :
- Constructors are used by the JVM in constructing the objects of that class.
- Even if we don’t define a constructor, there is always a default/no-args constructor associated with that class.
- Constructors look like methods. the only difference is that they don’t have a return type, not even void. But constructors are not methods.
- Constructors can have only one name and that is the name of the class.
- Apart from few exceptional cases, constructors are always public.
- A class can have multiple constructors with different type/number of parameters.
- Constructors with parameters are known as ‘parameterized constructors’.
- Constructors can be definitely overloaded.
- Constructors cannot be overridden.
Parameterized Constructor
Constructors which take one or more parameters are called parameterized constructors. Let’s have a look at the below example.
public class Coffee {
String name;
String colour;
String type;
//Default or no-args constructor
public Coffee() {
}
//constructor with just one parameter
public Coffee(String name) {
this.name = name;
}
public Coffee(String name, String colour) {
this.name = name;
this.colour = colour;
}
//constructor with 3 parameters
public Coffee(String name, String colour, String type) {
this.name = name;
this.colour = colour;
this.type = type;
}
public static void main(String[] args) {
Coffee coffee1 = new Coffee();
System.out.println("coffee name : " + coffee1.name + ", coffee colour : " + coffee1.colour + ", coffee type : " + coffee1.type);
Coffee coffee2 = new Coffee("Cappucino");
System.out.println("coffee name : " + coffee2.name + ", coffee colour : " + coffee2.colour + ", coffee type : " + coffee2.type);
Coffee coffee3 = new Coffee("Cafe Frape", "Light Brown");
System.out.println("coffee name : " + coffee3.name + ", coffee colour : " + coffee3.colour + ", coffee type : " + coffee3.type);
Coffee coffee4 = new Coffee("Cappi Nirvana", "Dark Brown", "Cold Coffee");
System.out.println("coffee name : " + coffee4.name + ", coffee colour : " + coffee4.colour + ", coffee type : " + coffee4.type);
Coffee coffee5 = new Coffee();
System.out.println("coffee name : " + coffee5.name + ", coffee colour : " + coffee5.colour + ", coffee type : " + coffee5.type);
}
}
Constructor Overloading
Having more than one constructor in a class itself is an example of constructor overloading. As we know all the constructors have the same name as class, they also have different parameters, and therefore they implement constructor overloading. The above example shows constructor overloading.
Difference Between Constructors and Methods
Constructors | Methods |
---|---|
Constructors have same name as the name of the class | Methods may have same name as class and different names as well |
Constructors don’t have a return type | Methods are bound to have a return type |
If no constructors are defined, then there is a default constructor provided implicitly | No method is provided implicitly. All the methods need to be defined explicitly |
Constructors are used to initialize the state of an object | Methods expose the behaviour of objects |
Quiz time -> Constructors don’t have a return type, do they still return anything?
Answer -> Yes. Constructor return the object of the class in which they are defined.