Properties class in Java
Properties class is the child class of Hashtable. It works in conjunction with properties file which is a text file. A properties file contains key-value in text format.
Properties class get us access to properties file.
Usage of properties file
Some times we need some properties to be used which we want to provide in such a way that we don’t have to restart our system or service. In those situations, we use properties file.
- First of all, create a file called test.properties in the root of the project
- Write java class
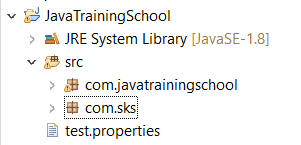
Example
package com.javatrainingschool;
import java.io.FileReader;
import java.util.Properties;
public class PropertiesFileExample {
public static void main(String[] args) throws Exception {
FileReader reader = new FileReader("test.properties");
Properties prop = new Properties();
prop.load(reader);
System.out.println(prop.getProperty("application.name"));
System.out.println(prop.getProperty("year"));
}
}
Output:
My Test Application
2021
Read properties file from resources folder in maven project
In this example, we will see how to read properties file if it resides in the resources folder in a maven project. Below is the directory structure for the same.
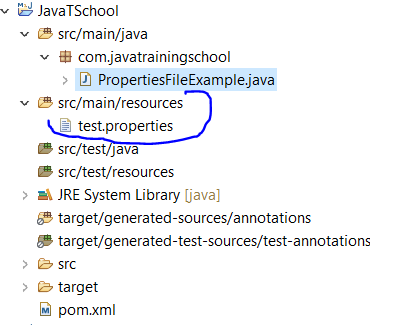
package com.javatrainingschool;
import java.io.File;
import java.io.FileReader;
import java.net.URL;
import java.util.Properties;
public class PropertiesFileExample {
public static void main(String[] args) throws Exception {
URL resource = new PropertiesFileExample().getClass().getClassLoader()
.getResource("test.properties");
File file = new File(resource.toURI());
FileReader reader = new FileReader(file);
Properties prop = new Properties();
prop.load(reader);
System.out.println(prop.getProperty("application.name"));
System.out.println(prop.getProperty("year"));
}
}
Output :
My Test Application
2021
System properties and how to iterate properties file
In this example, we will see how we can iterate over a properties file. For this, we will create a properties file by fetching system properties, and then iterate it.
package com.javatrainingschool;
import java.util.Map.Entry;
import java.util.Properties;
public class PropertiesFileExample {
public static void main(String[] args) throws Exception {
Properties prop = System.getProperties();
for(Entry<Object, Object> entry : prop.entrySet()) {
System.out.println(entry.getKey() + " : " + entry.getValue());
}
}
}
Read properties file from a hard-coded path
package com.javatrainingschool;
import java.io.FileReader;
import java.util.Properties;
public class PropertiesFileExample {
public static void main(String[] args) throws Exception {
FileReader reader = new FileReader("C:\\Users\\saras\\Desktop\\cutom-files\\test.properties");
Properties prop = new Properties();
prop.load(reader);
System.out.println(prop.getProperty("application.name"));
System.out.println(prop.getProperty("year"));
}
}
Create and set values to a properties file
package com.javatrainingschool;
import java.io.FileWriter;
import java.util.Properties;
public class PropertiesFileExample {
public static void main(String[] args) throws Exception {
Properties prop = new Properties();
prop.setProperty("application.name", "Test application");
prop.setProperty("launch.year", "2021");
prop.store(new FileWriter("C:\\Users\\saras\\Desktop\\cutom-files\\test.properties"),
"Java Training School Properties File Example");
System.out.println("File created successfully.");
}
}
After the program is executed successfully, please check at the location specified, you will see a file created with name test.properties. The content of the file should be as shown below
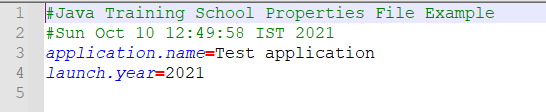