Handling Request Parameters
It is important to handle request parameters in right way. There are different ways in which this can be done. Below are the ways in which we can handle request parameters.
- @RequestParam annotation: It is used to bind the method parameters to web request parameters
- @RequestBody annotation: It is used to bind the method parameter to the body of the web request
- @PathVariable annotation: It is used to bind the method parameter to the URI template variable
1. @RequestParam
Let’s consider below form
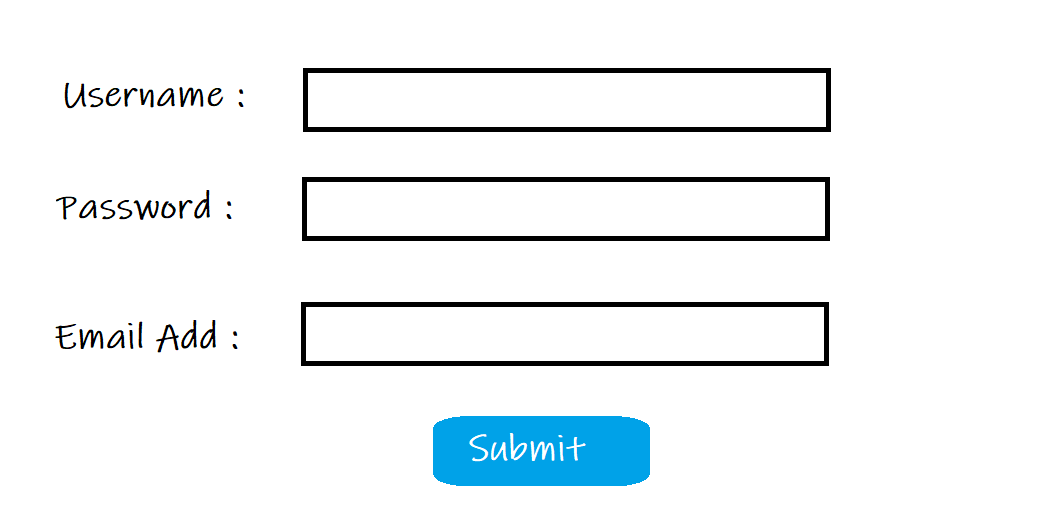
When we submit the above form with user details, the user request parameters will be handled using the @RequestParam annotation. The RequestParam annotation is used to bind a method parameter to a web request parameter. Let’s have a look at the below code to understand. userName, password, and emailAdd parameters are the names in the html file.
@Controller
@RequestMapping("/user/*")
public class UserController {
@GetMapping("/signup")
public String signup() {
return "signup";
}
@PostMapping("/signup/process")
public String processSignup(ModelMap model, @RequestParam("userName") String userName,
@RequestParam("password") String password, @RequestParam("emailAdd") String emailAdd) {
model.addAttribute("login", true);
model.addAttribute("name", userName);
return "index";
}
}
Query parameters from the url can also be mapped using @RequestParam annotation. such as
http://localhost:8080/user/signup/process?userName=Mark&password=dummy&[email protected]
2. @RequestBody
In this section, we will learn when and how to use the RequestBody annotation (@RequestBody) for handling web requests.
When the request parameters are sent as part of body of the request, RequestBody annotation is used to bind the method parameter with the body of the incoming request.
The RequestBody annotation is most commonly used in scenarios dealing with REST APIs where request is sent mostly as a json request.
The following example explains the usage of the @RequestBody annotation:
{
"name" : "Ashok Kumar",
"profession" : "actor",
"industry" : "Bollywood"
}
The above json request will be mapped to User object using @RequestBody annotation as shown below.
public class User {
private String name;
private String profession;
private String industry;
}
@RestController
public class DemoRestController {
@PostMapping("/hello")
public String hello(@RequestBody User user) {
String name = user.getName();
return "Hello " + name;
}
}
3. @PathVariable
The PathVariable annotation is used to bind a method parameter to a URI template variable. When request parameter is sent as path variable, it can be bound with method parameter using @PathVariable annotation. A URI template is a URI-like string containing one or more variables. For example, in the following code, /{name} is a path variable. A method can have multiple @Pathvariable annotations to bind multiple variables present in the URI template.
In the below example, userName will be mapped from the url path. http://localhost:8080/Mohan
Value of userName would be ‘Mohan’ in this case.
@RestController
@SpringBootApplication
public class UserApplication {
@RequestMapping("/")
public String home() {
return "index";
}
@GetMapping("/{userName}")
public String home(ModelMap model, @PathVariable String userName) {
model.addAttribute("name", userName);
return "index";
}
public static void main(String[] args) {
SpringApplication.run(HealthAppApplication.class, args);
}
}