Hashtable in Java
Hashtable is a data structure similar to HashMap. It contains key and value pair. Hashtable is a legacy class, meaning it was introduced before Collections framework in Java 1.2.
Important points about Hashtable
- Hashtable contains key-value pair.
- It contains unique keys.
- It doesn’t allow null keys or null values.
- Hashtable is synchronized and thus can be used in a multithreaded context very well.
Class declaration
public class Hashtable<K,V> extends Dictionary<K,V> implements Map<K,V>, Cloneable, Serializable
Class hierarchy
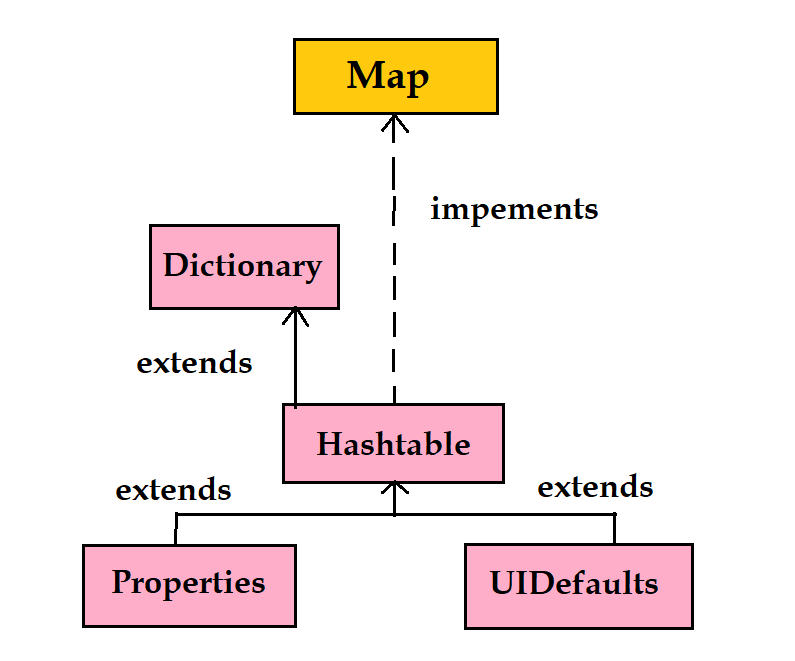
Example
package com.javatrainingschool;
import java.util.Enumeration;
import java.util.Hashtable;
public class HashTableExample {
public static void main(String[] args) {
Hashtable<Integer, String> ht = new Hashtable<Integer, String>();
ht.put(1, "The god of small things");
ht.put(2, "A fine Balance");
ht.put(3, "The white tiger");
ht.put(4, "A suitable boy");
Enumeration<Integer> en = ht.keys();
while(en.hasMoreElements()) {
int key = en.nextElement();
System.out.println("Key = " + key + " : value = " + ht.get(key));
}
}
}
Output :
Key = 4 : value = A suitable boy
Key = 3 : value = The white tiger
Key = 2 : value = A fine Balance
Key = 1 : value = The god of small things
Another way of iterating Hashtable using keySet() method
package com.javatrainingschool;
import java.util.Hashtable;
import java.util.Set;
public class HashTableExample {
public static void main(String[] args) {
Hashtable<Integer, String> ht = new Hashtable<Integer, String>();
ht.put(1, "The god of small things");
ht.put(2, "A fine Balance");
ht.put(3, "The white tiger");
ht.put(4, "A suitable boy");
Set<Integer> en = ht.keySet();
for(int key : en) {
String value = ht.get(key);
System.out.println("Key = " + key + " : value = " + value);
}
}
}
Another way of iterating Hashtable using entrySet() method
package com.javatrainingschool;
import java.util.Hashtable;
import java.util.Map.Entry;
import java.util.Set;
public class HashTableExample {
public static void main(String[] args) {
Hashtable<Integer, String> ht = new Hashtable<Integer, String>();
ht.put(1, "The god of small things");
ht.put(2, "A fine Balance");
ht.put(3, "The white tiger");
ht.put(4, "A suitable boy");
Set<Entry<Integer, String>> entrySet = ht.entrySet();
for(Entry<Integer, String> entry : entrySet) {
System.out.println("Key = " + entry.getKey() + " : value = " + entry.getValue());
}
}
}
remove() and putIfAbsent() methods
remove() method is used to remove a key and its value from Hashtalble. putIfAbsent() method is used to add an entry in the Hashtable only if the key is absent in it. If key is present, then the entry will not be added. Let’s see an example for both the methods.
package com.javatrainingschool;
import java.util.Hashtable;
import java.util.Map.Entry;
import java.util.Set;
public class HashTableExample {
public static void main(String[] args) {
Hashtable<Integer, String> ht = new Hashtable<Integer, String>();
ht.put(1, "The god of small things");
ht.put(2, "A fine Balance");
ht.put(3, "The white tiger");
ht.put(4, "A suitable boy");
//the below entry will not be added since key 3 is already present
ht.putIfAbsent(3, "The kite runner");
//this will remove key 1 and its value 'The god of small things'
ht.remove(1);
Set<Entry<Integer, String>> entrySet = ht.entrySet();
for(Entry<Integer, String> entry : entrySet) {
System.out.println("Key = " + entry.getKey() + " : value = " + entry.getValue());
}
}
}
Output :
Key = 4 : value = A suitable boy
Key = 3 : value = The white tiger
Key = 2 : value = A fine Balance