toString() method
toString() method belongs to java.lang.Object class. The purpose of toString() is to give the string representation of an object’s state.
The Default implementation of toString() method inside Object class is as follows
public String toString() {
return getClass().getName() + "@" + Integer.toHexString(hashCode());
}
It means if not overridden, calling toString() method on any object will simply print <Name-of-the-class>@HashCode. See the below example,
package com.javatrainingschool;
public class Scientist {
private String name;
private String country;
public Scientist(String name, String country) {
this.name = name;
this.country = country;
}
public static void main(String[] args) {
Scientist st = new Scientist("APJ Abdul Kalam", "India");
System.out.println(st.toString());
}
}
Output:
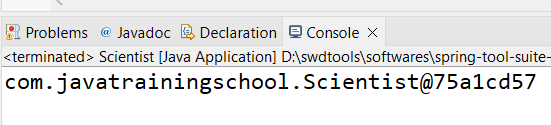
Note -> System.out.println(st.toString()) is similar to System.out.println(st)
Note that this doesn’t give any meaningful information about the object’s state. Therefore, if we want to display the values of an object’s properties, we need to override toString() method.
toString() example
package com.javatrainingschool;
public class Scientist {
private String name;
private String country;
public Scientist(String name, String country) {
this.name = name;
this.country = country;
}
public static void main(String[] args) {
Scientist st = new Scientist("APJ Abdul Kalam", "India");
System.out.println(st.toString());
}
@Override
public String toString() {
return "Scientist [name=" + name + ", country=" + country + "]";
}
}
Output:
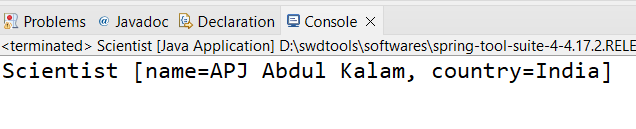
Note -> Every IDE provides a way to generate toString() method. So, it’s not necessary to write this method manually. For Eclispe/STS the short cut key is ctrl + shift + s, Generate toString()…