HashMap in Java
A map contains key and value pair. These keys are unique in nature and they identify values.
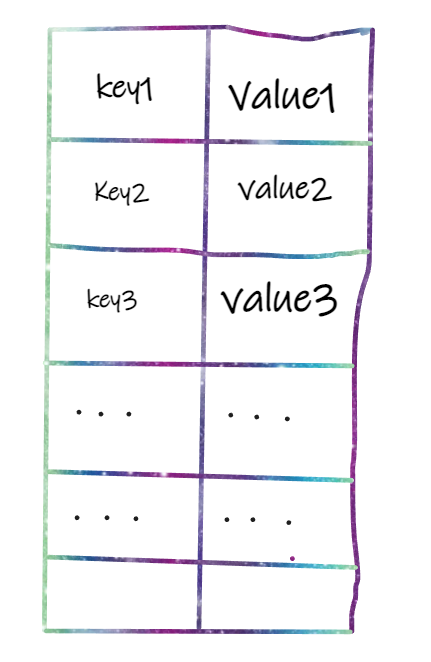
Hierarchy of Map
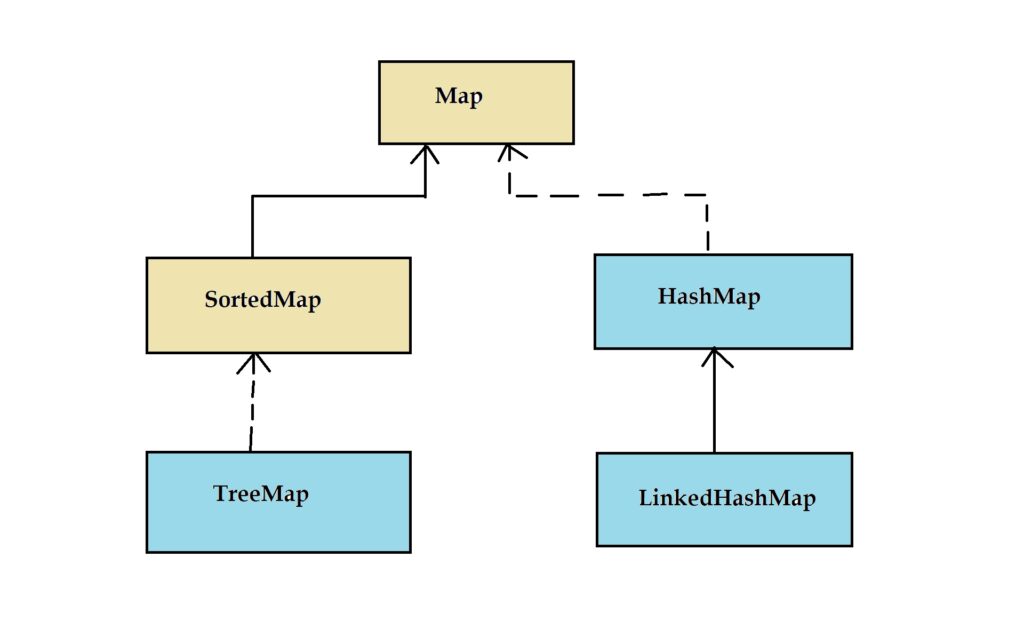
Some interesting features of Map
- Map stores key value pair as one entry in it.
- All keys are unique in nature. Therefore, duplicate keys are not allowed.
- However, duplicate values are allowed.
- HashMap and LinkedHashMap allows one null key but TreeMap doesn’t allow null key.
Map classes
- HashMap – Stores key-value pair. Doesn’t gaurantee any order of keys.
- LinkedHashmap – Stores key-value pair. It follows insertion order of keys.
- TreeMap – Stores key-value pair, It follows natural sorting order of keys.
Creating a map in Java
Syntax :
Map<K, V> map = new HashMap<K, V>();
where K represents type for a key, and V represents type for a value.
Examples :
Map<Integer, Integer> map = new HashMap<Integer, Integer>();
Map<Integer, String> map = new LinkedHashMap<Integer, String>();
Map<String, String> map = new TreeMap<String, String>();
How to add entries in a map in Java
There is a method called put which is used to add entries in a map
map.put(key, value);
HashMap
HashMap example
In the below example, we are going to create a hashMap of keys as id and name as value.
package com.javatrainingschool;
import java.util.HashMap;
import java.util.Map;
public class HashMapExample {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<Integer, String>();
map.put(1, "Einstein");
map.put(2, "Newton");
map.put(3, "Darwin");
System.out.println(map);
}
}
Output :
{1=Einstein, 2=Newton, 3=Darwin}
How to iterate over a HashMap in Java
Iteration over a hash map is not as simple as it is for list or set for the simple reason that it doesn’t have only elements. It has a pair of elements known as keys and values. So, how do we iterate over a HashMap or a map for that matter. We need to do following things to iterate over a map
- As a first step, get the set view of Map. Meaning, breaking down map into a set which contains Entry objects. These Entry objects have two attributes i.e. key and value
- Now, since we have a set representating the map, we know how to iterate over a set. Either use an iterator or for-each loop.
Let’s see how to break down map into a set.
Map<Integer, String> map = new HashMap<Integer, String>();
Set<Entry<Integer, String>> setViewOfMap = map.entrySet();
Now, since we have a set representing our map, we can traverse over it.
Iterator<Entry<Integer, String>> it = setViewOfMap.iterator();
Let’s see one full example
package com.javatrainingschool;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
public class HashMapExample {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<Integer, String>();
map.put(1, "Einstein");
map.put(2, "Newton");
map.put(3, "Darwin");
Set<Entry<Integer, String>> setViewOfMap = map.entrySet();
Iterator<Entry<Integer, String>> it = setViewOfMap.iterator();
while(it.hasNext()) {
Entry<Integer, String> entry = it.next();
Integer key = entry.getKey();
String value = entry.getValue();
System.out.println("Key = " + key + " | Value = " + value);
}
}
}
Output :
Key = 1 | Value = Einstein
Key = 2 | Value = Newton
Key = 3 | Value = Darwin
Iterate over a HashMap using for-each loop
package com.javatrainingschool;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
public class HashMapExample {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<Integer, String>();
map.put(1, "Einstein");
map.put(2, "Newton");
map.put(3, "Darwin");
Set<Entry<Integer, String>> setViewOfMap = map.entrySet();
for(Entry<Integer, String> entry : setViewOfMap) {
Integer key = entry.getKey();
String value = entry.getValue();
System.out.println("Key = " + key + " | Value = " + value);
}
}
}
Map.Entry Interface
Entry is the subinterface (inner interface/ nested interface) of Map interface. That’s why it is accessed using Map.Entry. It returns a collection-view of the map, whose elements are of this class. It provides methods to get key and value of the map. We have already seen its examples.
What happens if we try to add duplicate keys in HashMap
Let’s consider above example and try to add a duplicate key. What will happen?
We know that map doesn’t allow duplicate keys. So, if we try to add a duplicate key, then it will simply replace the new value with old one against this key. Below is an example
map.put(1, "Einstein");
map.put(1, "Newton");
map.put(2, "Darwin");
//in this case, there will be only two entries in the map. Against, key 1, value will be Newton.
HashMap with custom values example
In the below example, we will create one custom class Scientist and add its objects to HashMap as values. We will keep id as keys.
package com.javatrainingschool;
public class Scientist {
private int id;
private String name;
private String speciality;
//getters and setters methods
public Scientist(int id, String name, String speciality) {
super();
this.id = id;
this.name = name;
this.speciality = speciality;
}
@Override
public String toString() {
return "Scientist [id=" + id + ", name=" + name + ", speciality=" + speciality + "]";
}
}
Testing class with main method
package com.javatrainingschool;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
public class HashMapExample {
public static void main(String[] args) {
Scientist s1 = new Scientist(1, "Einstein", "Physicist");
Scientist s2 = new Scientist(2, "Newton", "Physicist");
Scientist s3 = new Scientist(3, "Darwin", "Biologist");
Map<Integer, Scientist> map = new HashMap<Integer, Scientist>();
map.put(1, s1);
map.put(2, s2);
map.put(3, s3);
Set<Entry<Integer, Scientist>> setViewOfMap = map.entrySet();
for(Entry<Integer, Scientist> entry : setViewOfMap) {
Integer key = entry.getKey();
Scientist value = entry.getValue();
System.out.println("Key : " + key + " | Value : " + value);
}
}
}
Output :
Key : 1 | Value : Scientist [id=1, name=Einstein, nationality=Physicist]
Key : 2 | Value : Scientist [id=2, name=Newton, nationality=Physicist]
Key : 3 | Value : Scientist [id=3, name=Darwin, nationality=Biologist]
How to remove, replace entries in Map
There are two methods which can be used to remove entries from map. Also, there is a method to replace entires in map.
map.remove(2); //this will remove entry whose key is 2
map.remove(1, "Einstein"); //this will only remove an entry if key and value are matching
map.replace(1, "Newton"); //this will replace the existing value with new one
putIfAbsent() method in Map
putIfAbsent() method only adds an entry of key value pair, if there is no value associated with the key already in the map. This method is used to make sure that an already existing value is not replaced with the new value against a key.
map.putIfAbsent(1, "Darwin");
//This will only add Darwin against key 1, if there is not an entry already in the map against key 1