JDBC Connectivity Example with SQL Server
In this tutorial, we will connect to SQL Server
Create a database table books with columns like below
bookid varchar(10), bookName varchar(255), authorName(255)
Step 1 : Load the driver
Class.forName(“com.microsoft.sqlserver.jdbc.SQLServerDriver”);
Step 2 : Get the connection object
String dbURL = “jdbc:sqlserver://localhost:1433;databaseName=saras_test_db;
integratedSecurity=true;authenticationScheme=NTLM;encrypt=true;trustServerCertificate=true;”;
package com.sks;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class JDBCConnectivityExample {
public static void main(String[] args) throws ClassNotFoundException, SQLException {
// Step 1 : Load the driver
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
String dbURL = "jdbc:sqlserver://localhost:1433;databaseName=saras_test_db;integratedSecurity=true;authenticationScheme=NTLM;encrypt=true;trustServerCertificate=true;";
String userName = "abcd";
String pass = "1234";
// Step 2 : Getting the connection
Connection con = DriverManager.getConnection(dbURL, userName, pass);
// Step 3: Getting statement object
Statement stmt = null;
stmt = con.createStatement();
// Step 4 : Executing the query
String query = "select * from books";
ResultSet rs = stmt.executeQuery(query);
while (rs.next()) {
String id = rs.getString("bookid");
String name = rs.getString("bookname");
String author = rs.getString("authorname");
System.out.println("Book id : " + id);
System.out.println("Book name : " + name);
System.out.println("Author Name : " + author);
System.out.println("__________________________________________");
}
}
}
Output:
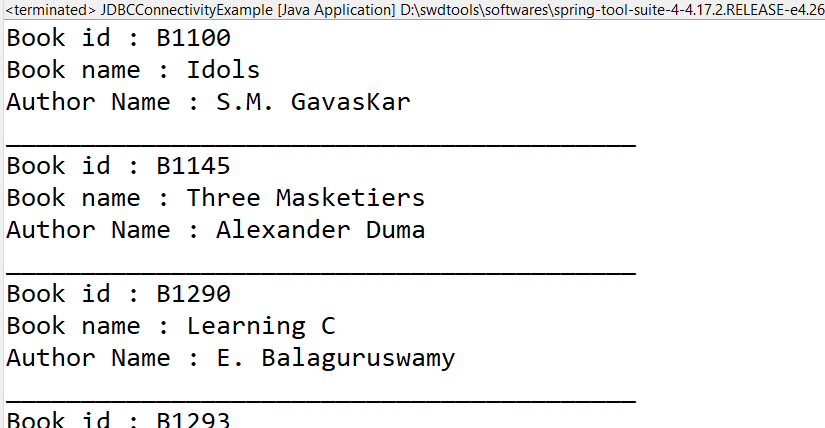