SendRedirect method
HttpServletResponse iterface has a method sendRedirect(). This method can be used to redirect response from one servlet to another resource. The resource can be a servlet, jsp or html file.
We can pass a url which is relative to the existing application. Or we can pass an absolute url as well, such as www.google.com, www.javatrainingschool.com etc.
The redirection of response using sendRedirect method happens at client side. It means the new url is called from the browser again. This is why it can redirect the response inside as well as outside the server.
response.sendRedirect("https://www.javatrainingschool.com");
Create a html file customSearch.html
<!DOCTYPE html>
<html>
<head>
<title>Custom Search Using sendRedirect</title>
</head>
<body>
<form action="customSearch">
<input type="text" name="textToSearch">
<input type="submit" value="Search it on Bing">
</form>
</body>
</html>
CustomSearchServlet.java
package com.sks;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class CustomSearchServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String textToSearch = request.getParameter("textToSearch");
response.sendRedirect("https://www.bing.com/search?q=" + textToSearch);
}
}
Servlet mappings in web.xml
<servlet>
<servlet-class>com.sks.CustomSearchServlet</servlet-class>
<servlet-name>customServlet</servlet-name>
</servlet>
<servlet-mapping>
<servlet-name>customServlet</servlet-name>
<url-pattern>/customSearch</url-pattern>
</servlet-mapping>
Run the server and access the application
url – http://localhost:8080/my-first-servlet-app/customSearch.html
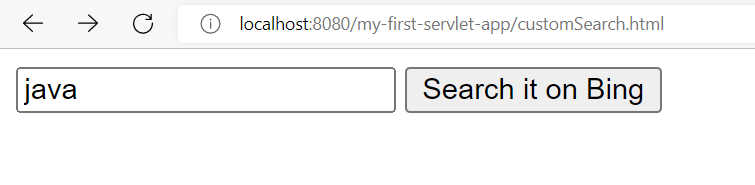
After clicking on the ‘Search it on Bing’
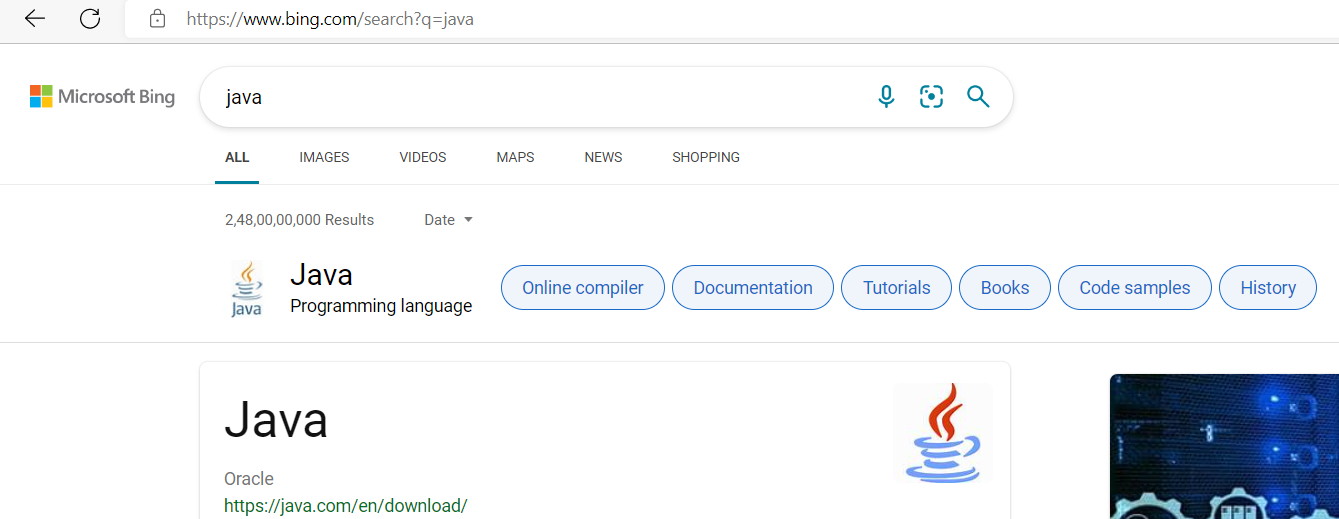