Collections Framework In Java
Collections framework in java is a set of interfaces, abstract classes, and classes which helps us store group of data in various data structures like list, queue, stack, set, map etc.
What is the need of Collections Framework?
Storing data and retrieving the same is a very common phenomenon in computing world. It is also important in which way we store and retrieve it. To overcome the hassle of doing the same things again and again, java has provided collections framework to the developers so that they can focus more on their business logic. It’s time to have a look at the structure of this framework.
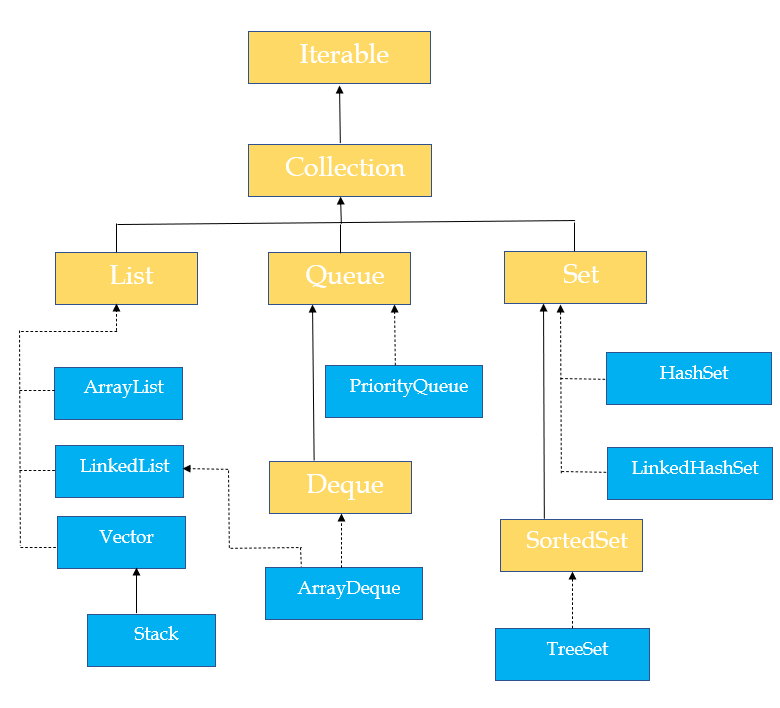
There is another data structure called Map, which is, although, not part of the above hierarchy, but it is part of collections framework. Below is its hierarchy.
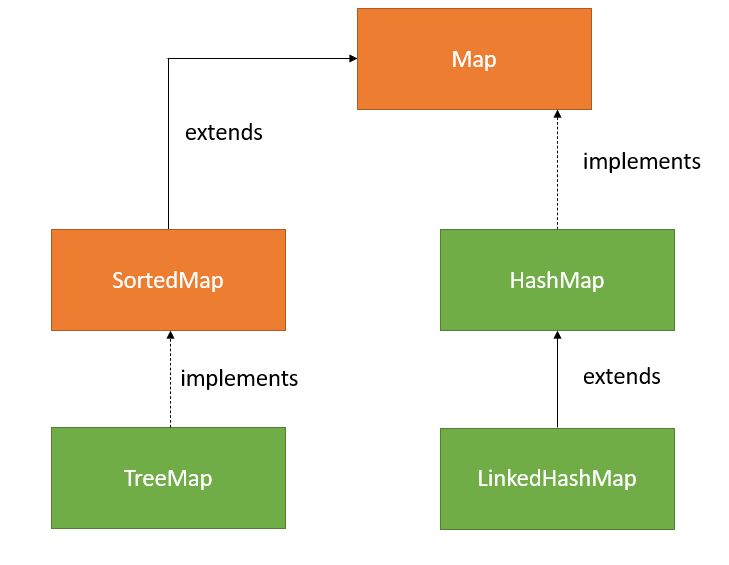
Iterable Interface
This interface is the top level interface of collections framework. This define a type for all the collection classes. All Iterable classes can be iterated. It has only one method in it.
public interface Iterable {
Iterator<T> iterator();
}
Collection Interface
This is second interface in the hierarchy which extends Iterable. This interface declares methods which are application for all kinds of Collection type classes like ArrayList, HashSet, LinkedHashSet etc. Some of those common methods are:
boolean add(E e);
boolean remove(Object o);
boolean isEmpty();
List Interface
This interface defines a type that all the classes implementing this will store objects in an ordered manner. This also allows duplicate elements.
Concrete classes of this interface are ArrayList, LinkedList, Vector, Stack. We shall learn about all these classes in next chapter
Queue Interface
Queue represents a data structure which follows First-In-First-Out (FIFO) ordering of elements. So, the first element that is added to the queue is the one that will be the first element to be out of the queue.
Concrete classes of this interface are PriorityQueue and ArrayDeque. We shall learn about them in next chapter
Set Interface
Set is a data structure which doesn’t allow duplicate elements and has all elements unique. That is the specific use of Set interface. Concrete classes of this interface are HashSet, LinkedHashSet, and TreeSet.
Map Interface
Map is a data structure which stores key and values pair as one entry in it. The only condition is that all the keys should be unique. Its concrete classes are HashMap, LinkedHashMap, and TreeMap. We shall talk about them in detail in next chapters.