Spring Boot with Swagger3
The biggest challenge with microservices is their maintenance in terms of contract. What type of request/response is needed for a service. Usually this is recorded in document form and is known as service documentation. Earlier it was done manually and therefore was error prone.
The solution to this issue is Swagger framework. Swagger is a tool, a specification and a complete framework implementation for producing the visual representation of RESTful Web Services. Documentation of services with Swagger can be done at a very fast pace. In fact, the moment the service is ready, its documentation is also ready if you implement Swagger. When properly defined via Swagger, a client can understand and interact with the service with minimal amount of implementation logic. Swagger eliminated any kind of guesswork in calling the service.
OpenAPI specification
OpenAPI Specification (formerly known as Swagger Specification) is an API description format for REST APIs. An OpenAPI file allows us to describe our entire API. These includes:
- Available endpoints and operations on each endpoint
- Operation parameters Input and output for each operation
- Authentication methods
- Contact information, license, terms of use and other information.
API specifications can be written in YAML or JSON. The format is easy to learn and readable to both humans and machines.
What is Swagger
Swagger is a JSON-based interface description language for specifying RESTful APIs for microservices with spring boot applications. Swagger is used with a set of oepn-source software tools to design, construct, describe, and consume RESTful web services. Automated documentation, code generation, and test-case generation are all included in Swagger api framework.
Example
In order to apply swagger, we only need one dependency in the classpath and that is it. We don’t need any other configuration.
<dependency>
<groupId>org.springdoc</groupId>
<artifactId>springdoc-openapi-ui</artifactId>
<version>1.5.9</version>
</dependency>
Step 1 : Create one spring starter project in STS and add below dependencies. pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.javatrainingschool</groupId>
<artifactId>spring-boot-swagger3</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>spring-boot-swagger3</name>
<description>Demo application for Spring Boot Swagger3</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.2.4.RELEASE</version>
<relativePath /> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springdoc</groupId>
<artifactId>springdoc-openapi-ui</artifactId>
<version>1.5.9</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Step 2 : Create Sport.java class
package com.javatrainingschool.model;
public class Sport {
private int id;
private String name;
private String noOfPlayers;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getNoOfPlayers() {
return noOfPlayers;
}
public void setNoOfPlayers(String noOfPlayers) {
this.noOfPlayers = noOfPlayers;
}
@Override
public String toString() {
return "Sport [id=" + id + ", name=" + name + ", noOfPlayers=" + noOfPlayers + "]";
}
public Sport(int id, String name, String noOfPlayers) {
super();
this.id = id;
this.name = name;
this.noOfPlayers = noOfPlayers;
}
public Sport() {
super();
// TODO Auto-generated constructor stub
}
}
Step 3 : Create BasicRestController.java class
package com.javatrainingschool.controller;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import com.javatrainingschool.model.Sport;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
@RequestMapping("/api")
@RestController
public class BasicRestController {
List<Sport> sports = new ArrayList<Sport>();
{
sports.add(new Sport(1,"Cricket", "11"));
sports.add(new Sport(2,"Hockey", "11"));
sports.add(new Sport(3,"Tennis", "2"));
sports.add(new Sport(4,"Kabaddi", "7"));
}
@RequestMapping(value = "/getSports", method = RequestMethod.GET, produces = "application/json")
public List<Sport> getSports() {
return sports;
}
@RequestMapping(value = "/getSport/{id}", method = RequestMethod.GET, produces = "application/json")
public Sport getSportById(@PathVariable(value = "id") int id) {
return sports.stream().filter(x -> x.getId()==(id)).collect(Collectors.toList()).get(0);
}
@RequestMapping(value = "/getSport/noOfPlayers/{no}", method = RequestMethod.GET, produces = "application/json")
public List<Sport> getSportByNoOfPlayers(@PathVariable(value = "no") String no) {
return sports.stream().filter(x -> x.getNoOfPlayers().equalsIgnoreCase(no))
.collect(Collectors.toList());
}
}
Step 4 : Run and test the application
Access the below url and you will see the below output. It will have all the endpoints. You can also test thest endpoints from this ui.
http://localhost:8080/swagger-ui.html
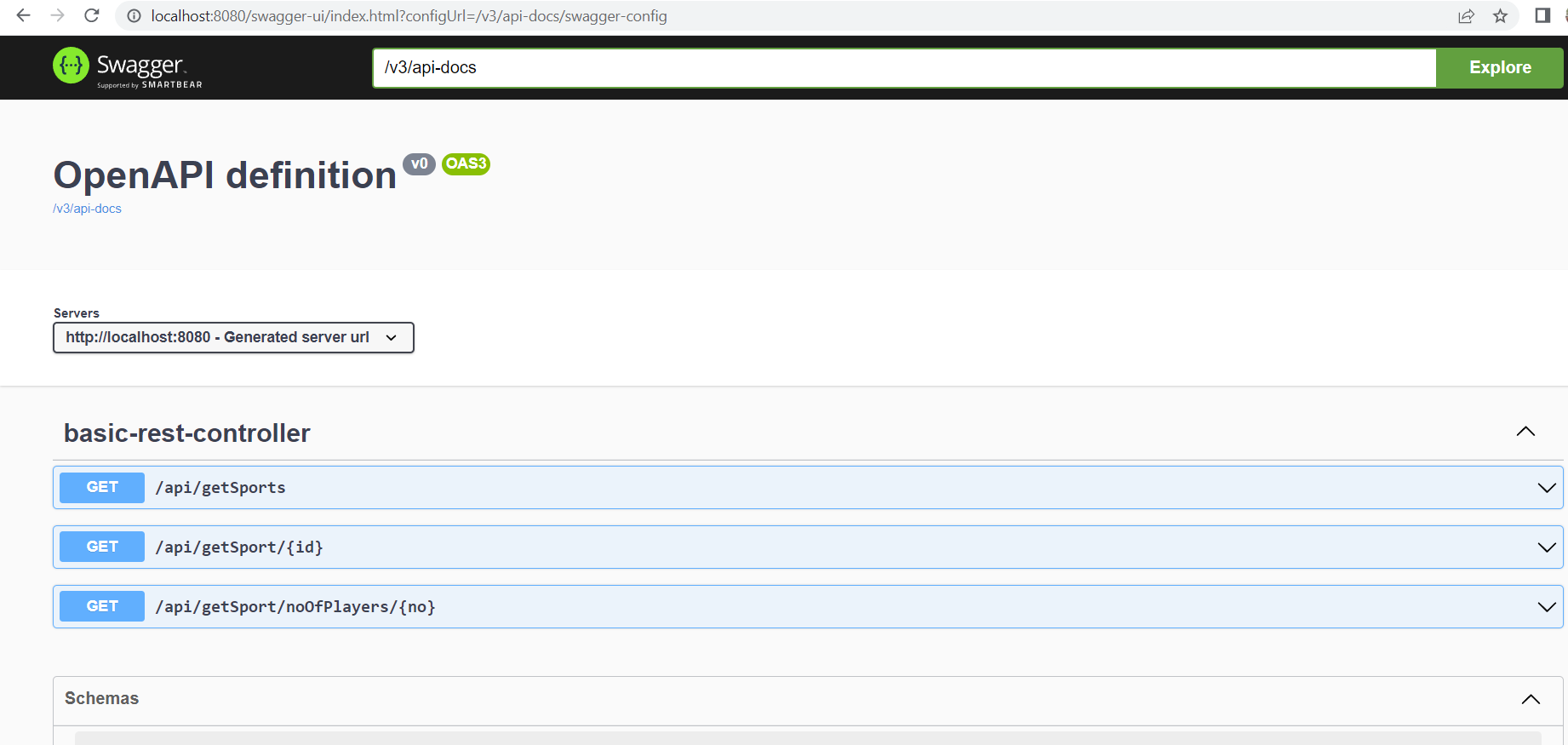

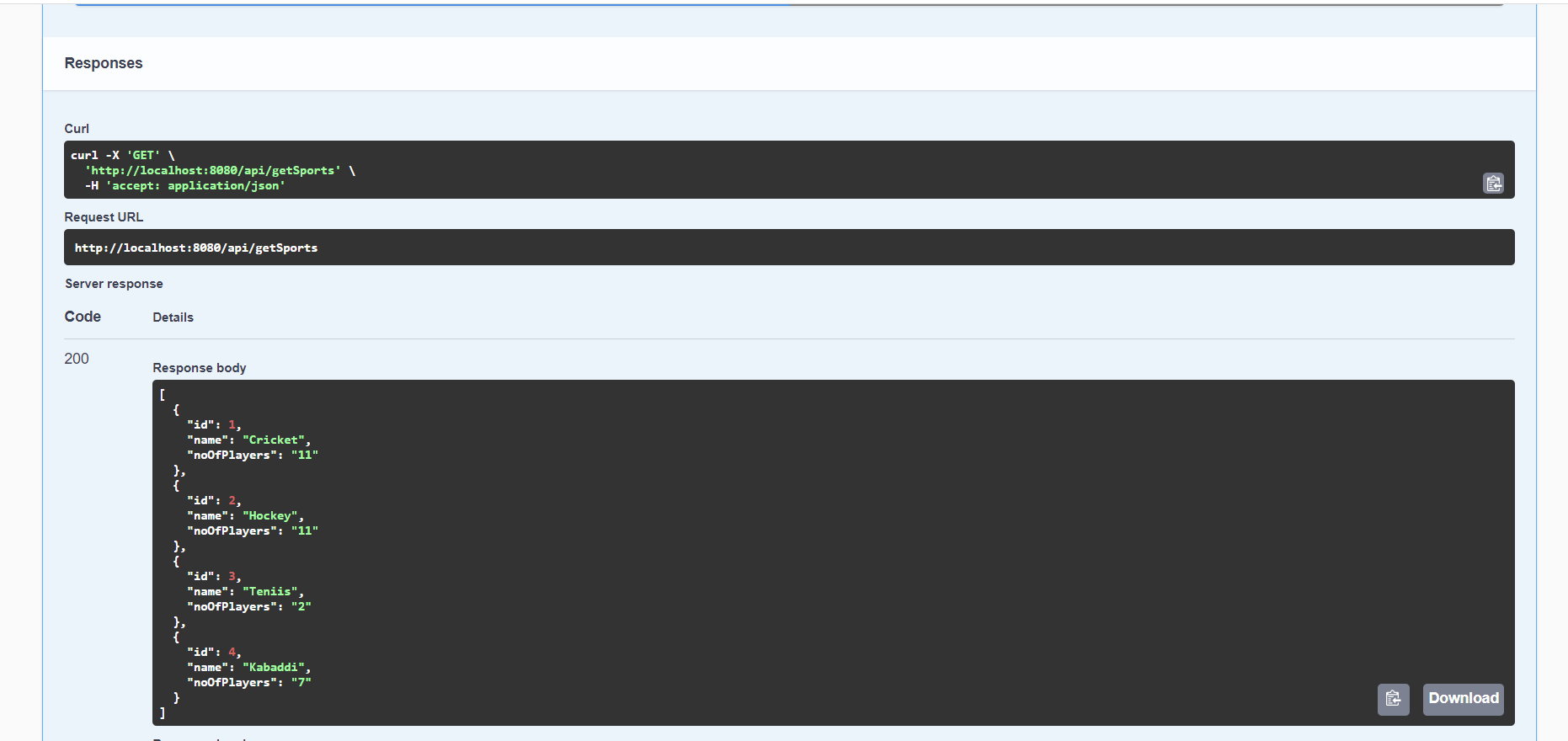