jsp:getProperty and setProperty
jsp:getProperty and jsp:setProperty action tags
jsp:getProperty and jsp:setProperty actions tags are used to get and set properties of a java bean respectively. It uses getter/setter methods for this purpose.
In the below example, let’s first create a java bean called Cricketer. Don’t forget to generate getter/setter methods.
Cricketer.java
package com.javatrainingschool;
public class Cricketer {
private String name;
private String country;
private int noOfRuns;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
public int getNoOfRuns() {
return noOfRuns;
}
public void setNoOfRuns(int noOfRuns) {
this.noOfRuns = noOfRuns;
}
@Override
public String toString() {
return "Cricketer [name=" + name + ", country=" + country + ", noOfRuns=" + noOfRuns + "]";
}
}
index.jsp
<html>
<body>
<form action="process.jsp" method="post">
Cricketer Name :
<input type="text" name="name">
<br>
Country :
<input type="text" name="country">
<br>
No of runs :
<input type="text" name="noOfRuns">
<br>
<input type="submit" value="Submit">
</form>
</body>
</html>
Note that here names of the html elements must be same as the name of the properties in java bean class. For example against noOfRuns property of java bean class, we have html text element with name as ‘noOfRuns’. If it is not same, jsp:setProperty and jsp:getProperty will not work.
process.jsp
<html>
<body>
<jsp:useBean id="c" class="com.javatrainingschool.Cricketer"></jsp:useBean>
<jsp:setProperty property="*" name="c"/>
Cricketer Info:<br>
<hr>
Name :
<jsp:getProperty property="name" name="c"/>
<br>
Country :
<jsp:getProperty property="country" name="c"/>
<br>
No of runs :
<jsp:getProperty property="noOfRuns" name="c" />
<br>
</body>
</html>
Output:
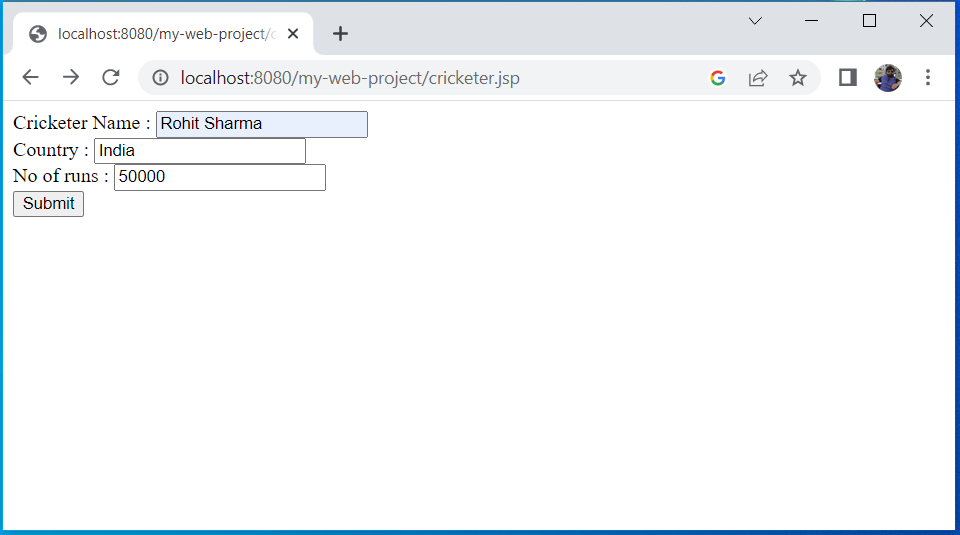
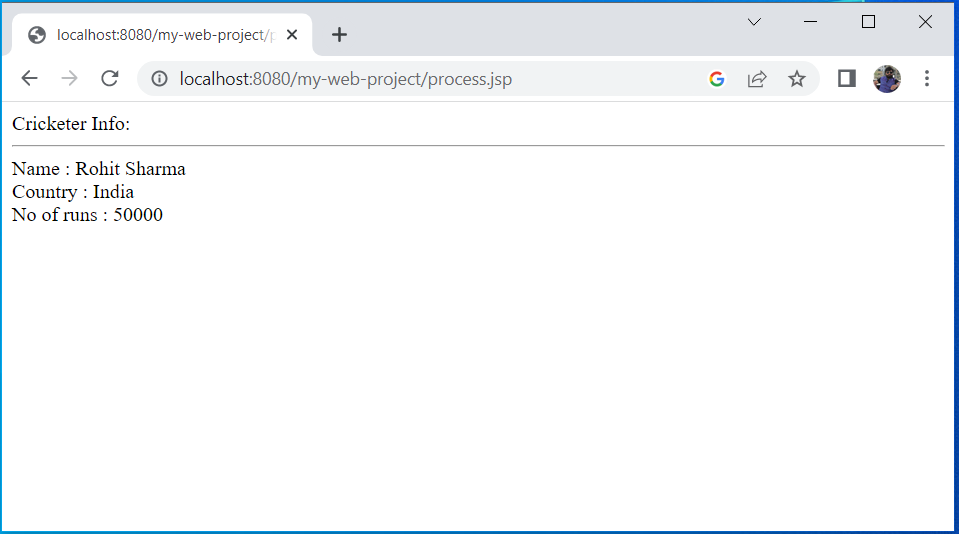