Checked Exception Example
In the below example, we are trying to read from a file. The below program may throw two checked exceptions, FileNotFoundException and IOException. Therefore, it is mandatory to handle these.
If the program finds the file, it prints the content of the file as in the below output.
package com.sks.exception;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
public class CheckedExceptionExample {
public static void main(String[] args) {
readFile();
}
public static void readFile() {
File file = new File("D:\\practice\\test1.txt");
FileReader reader = null;
// compiler makes it mandatory to handle FileNotFoundException
// since it is a compile time/checked exception
try {
reader = new FileReader(file);
} catch (FileNotFoundException e) {
e.printStackTrace();
}
BufferedReader bf = new BufferedReader(reader);
String str = null;
try {
while ((str = bf.readLine()) != null) {
System.out.println(str);
}
reader.close();
bf.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output :
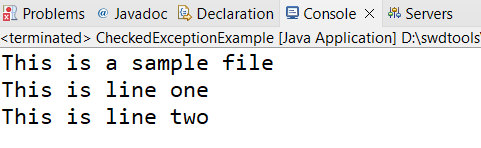
If the program doesn’t find the file, it gives exception as shown below
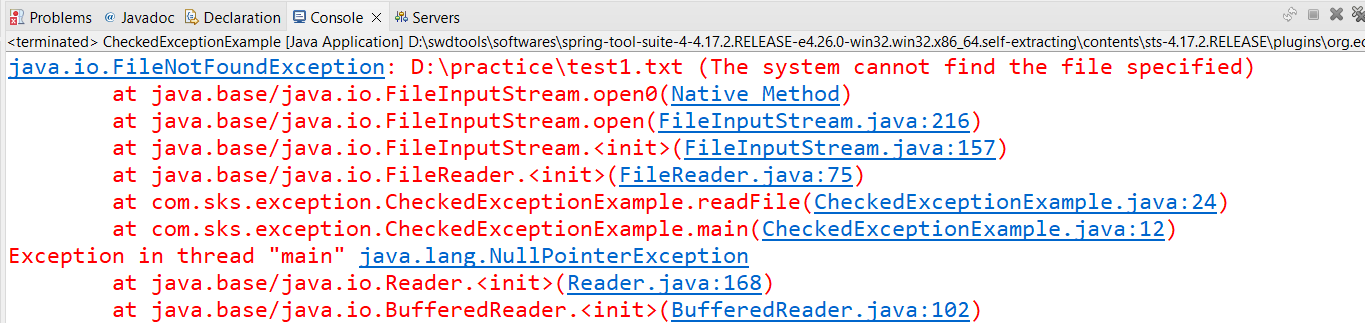