Multithreading
Multithreading is a mechanism in which a task or a set of tasks are performed using multiple threads. This results in enhanced throughput of a system.
Java also supports multithreading. By default also java programs are run in a thread. That default thread is called ‘main’ thread. Before proceeding let’s see how we can see the default thread and verify its name.
package com.javatrainingschool;
public class MainThreadExample {
public static void main(String[] args) {
String defaultThreadName = Thread.currentThread().getName();
System.out.println("Default thread name : " + defaultThreadName);
}
}
Output :
Default thread name : main
What is the need of threads
This is a valid question what the need of multiple threads is. Let’s understand this with a practical example.
Suppose, a house is being built. And the owner of the house has appointed just one person. The same person is mixing the raw materials, the same person is carrying the bricks, and the same person is constructing the walls also. Don’t you think it is the wrong way of building the house. Months or maybe years make take for completion of such a house. The best way to solve this problem is to employ several people to do specific jobs. Time will also reduce significantly.
Same example can be applied to programming world. We can make use of multiple threads to perfrom a business logic to increase the response time. This is why multithreading is needed.
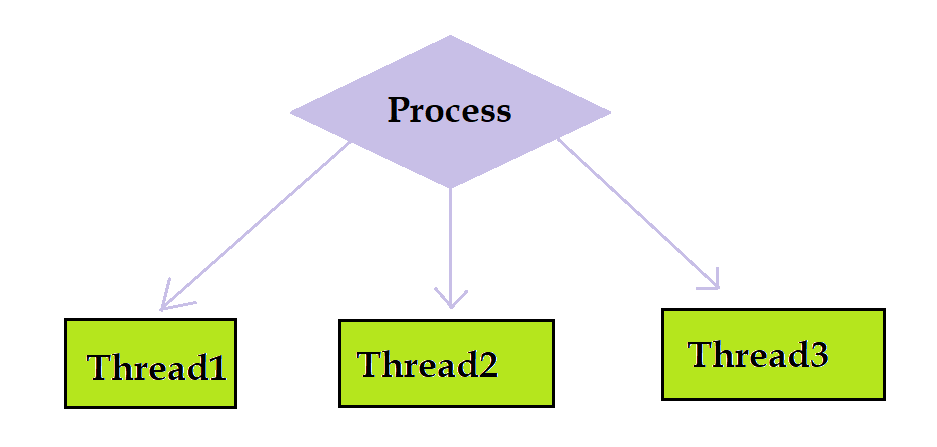
Multiprocessing
Multiprocessing is another technique to do multitasking. But it is carried out by multiple processes rather than threads. Process is a costly affair. Threads reside under a process and thus use same memory address. That’ why communication between threads is also faster. Let’s see the differences between multithreading and multiprocessing.
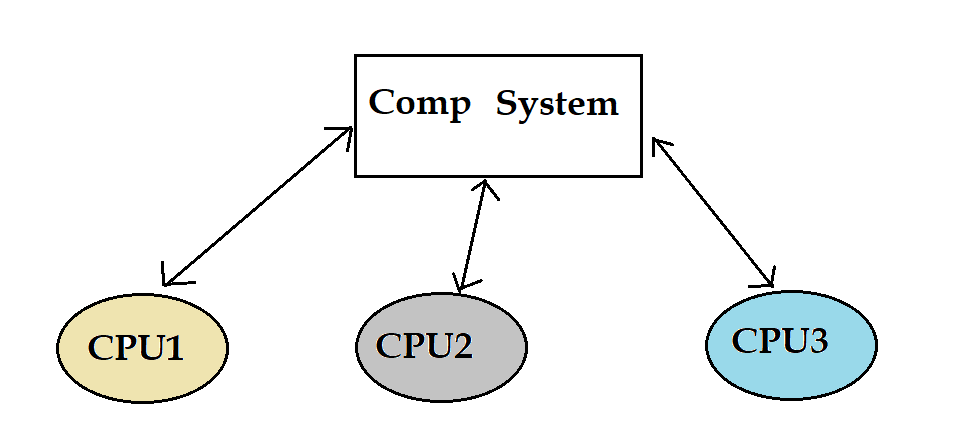
Multiprocessing vs Multithreading
Multiprocessing | Multithreading |
---|---|
Multiprocessing, as name suggests, is based on processes | Multithreading is based on threads |
Many CPUS work together to achieve multiprocessing | Multiple threads are created in a single process within the same CPU in case of multithreading |
Is categorized as symmetric and asymmetric | No such category in threads |
Process creation is a costly task | Threads creation is relatively much cheaper |
Every process has its own memory and address space | Threads share same memory space |
Inter process communication is time consuming and costly affair | Inter thread communication is much faster and less costly |
How is Thread represented in Java
java.lang.Thread class represents a thread in java. Any class that extends java.lang.Thread class is also a thread.
public class MyOwnThread extends Thread
//here objects of MyOwnThread class are java threads