Exception Handling in JSP
Let’s assume we have developed a jsp application. On one of the pages that executes some java code, some exception occurs. What will happen? If it has not been handled, something like this will appear on the browser
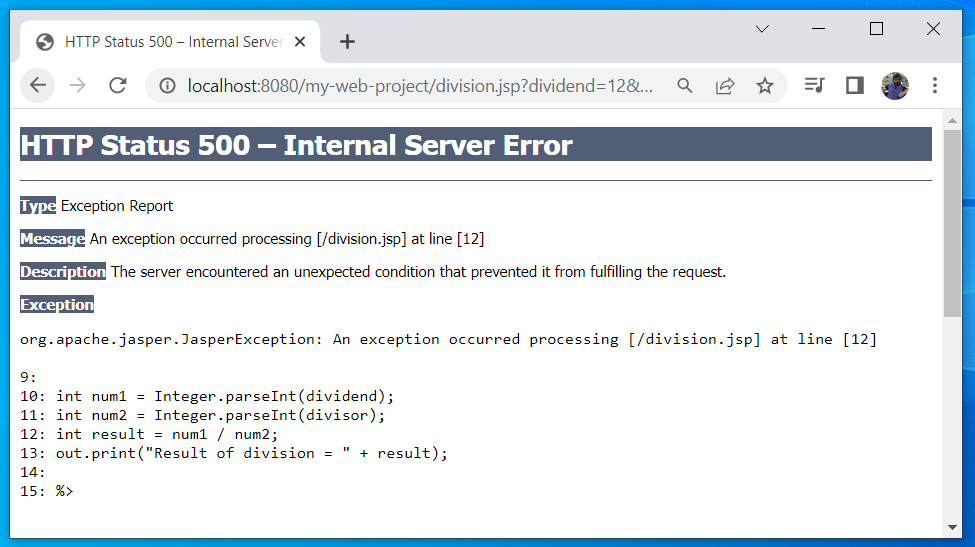
Clearly, it isn’t a good user experience. Also, the user has no way to navigate to other pages on the application. He/she has to open the application again on the browser.
To handle such exception scenarios, jsp provides a way by the use of errorPage and isErrorPage attribute of page directive.
Steps to handle exception in jsp
- Create index.jsp
<html>
<body>
<form action="division.jsp">
Dividend:
<input type="text" name="dividend" /><br /><br />
Divisor:
<input type="text" name="divisor" /><br /><br />
<input type="submit" value="Divide" />
</form>
</body>
</html>
2. Create division.jsp
<%@ page errorPage="error.jsp" %>
<html>
<body>
<%
String dividend = request.getParameter("dividend");
String divisor = request.getParameter("divisor");
int num1 = Integer.parseInt(dividend);
int num2 = Integer.parseInt(divisor);
int result = num1 / num2;
out.print("Result of division = " + result);
%>
</body>
</html>
3. Create error.jsp
<%@ page isErrorPage="true" %>
<html>
<body>
<h2>Divisor cannot be zero. Please try again.</h2>
<a href="index.jsp">Try Again</a>
</body>
</html>
Output:
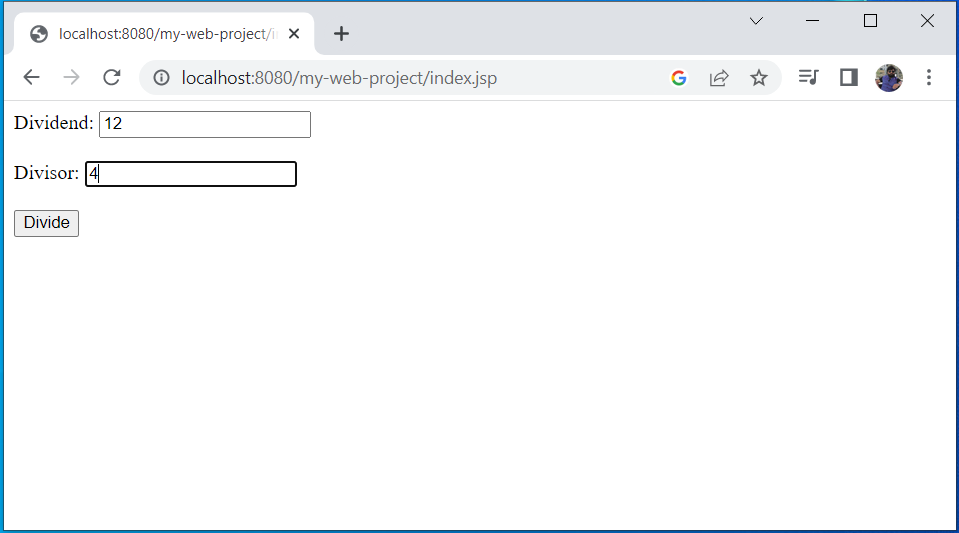
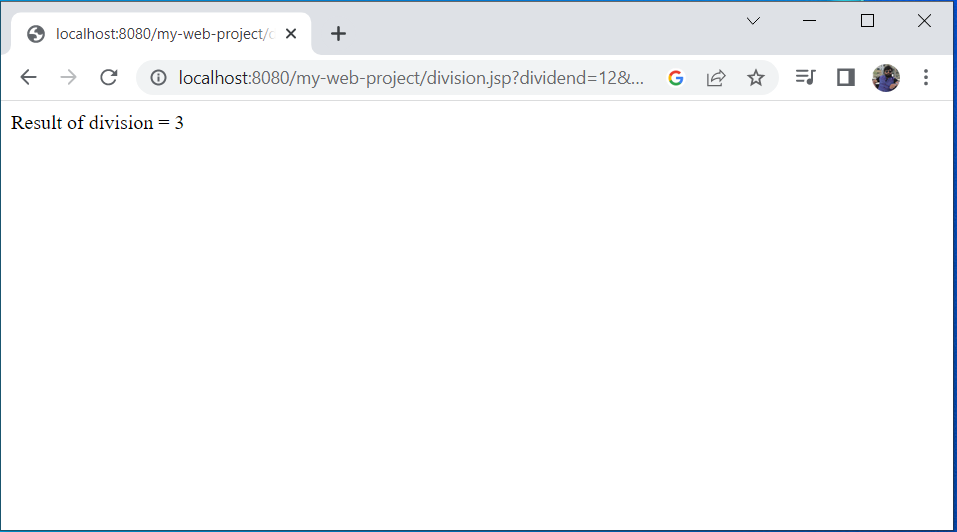
Exception scenario:
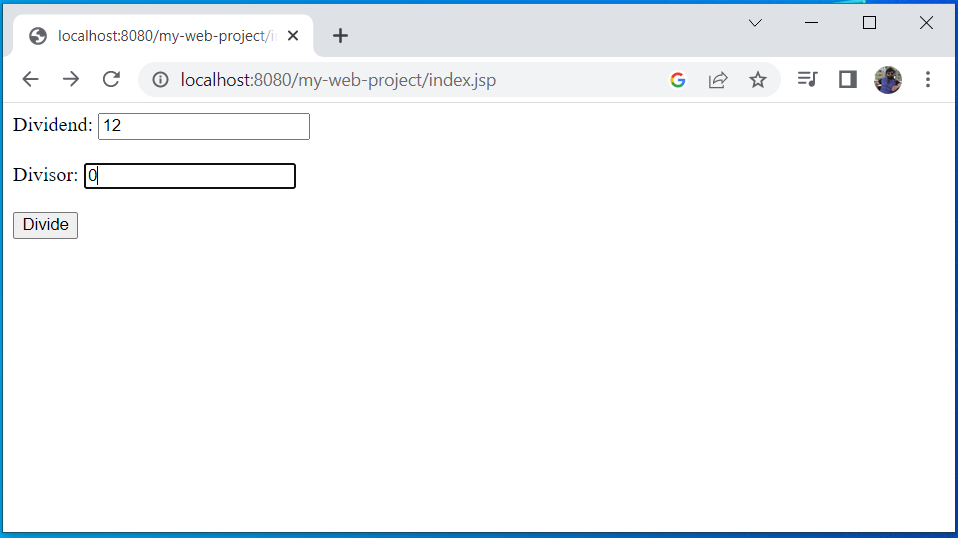
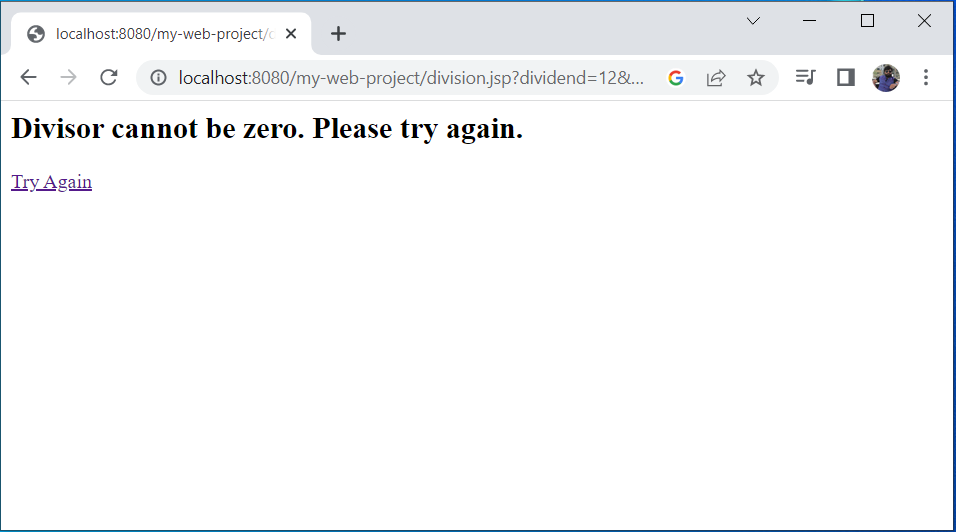
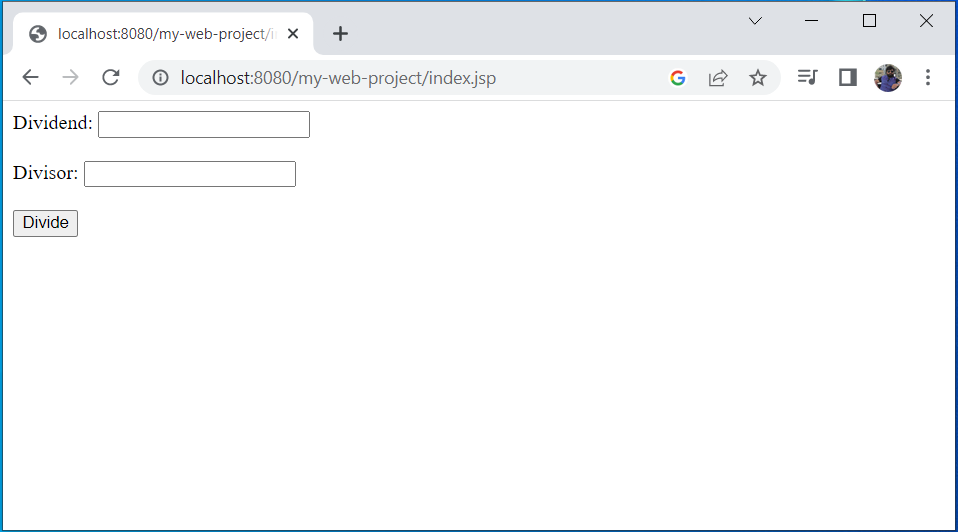