Serialization in Java
Serialization is the process of writing an object’s state into byte stream. The object’s whole information is contained in the byte-stream. The concept of serialization is frequently utilized in areas like Hibernate, JMS, JPA, and EJB.
In Java, the term “Serialization” refers to the idea of encoding an object’s state as a byte-stream. The object’s whole information is contained in the byte-stream. The concept of serialization is frequently utilized in areas like Hibernate, JMS, JPA, and EJB.
Serialization concept helps transport the code from one JVM to another and then de-serialize it there.
The reverse operation of serialization is called deserialization where object in the memory is formed from its byte-stream. Both the operations of serialization and deserialization are platform-independent, it means you can serialize an object on one platform and deserialize it on a different platform.
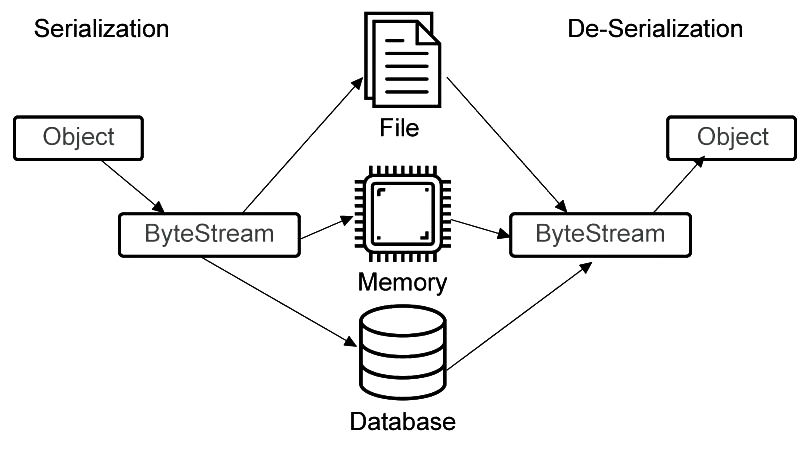
Need For Serialization
The following justifies our necessity for serialization:
- Communication: The processes of object serialization and transmission are involved in serialization. This makes it possible for several computers to design, share, and use objects at once.
- Deep Copy: By employing Serialization, cloning is simplified. By serializing an object to a byte array and then de-serializing it, one can create an exact copy of the object.
- Caching: When compared to the time needed to de-serialize an object, creating one takes longer. By caching the massive objects, serialization reduces time consumption.
- Persistence: By using serialization on it, every object’s state can be directly saved and afterwards retrieved by storing it in a database.
Important Points to be noted for Serialization
A few requirements must be satisfied in order to serialize an object. Before reading the rest of the article, there are a few additional important aspects that should be emphasized. These are the prerequisites and things to keep in mind when utilizing Java serialization.
- Only by implementing the serializable interface can you serialize an object.
- Serializable is a marker interface with no data member or method.
- If a field is not to be serialized, then mark it transient by using transient keyword before it.
- If the parent class implements the Serializable interface, the child class is not required to do so.
- Only non-static data members are saved during the serialization process; neither static nor transitory data members are saved.
- All wrapper classes and the String by default implement the Serializable interface.
Serialization Code Example
MyObject.java
import java.io.*;
class MyObject implements Serializable {
private String message;
private int value;
public MyObject(String message, int value) {
this.message = message;
this.value = value;
}
public String getMessage() {
return message;
}
public int getValue() {
return value;
}
}
ObjectSerializationExample.java
public class ObjectSerializationExample {
public static void main(String[] args) {
// Create an object to serialize
MyObject obj = new MyObject("Hello, world!", 42);
// Serialize the object
try {
FileOutputStream fileOut = new FileOutputStream("object.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(obj);
out.close();
fileOut.close();
System.out.println("Object has been serialized!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Object has been serialized!
In this example, the MyObject class implements the Serializable interface, and an instance of this class is serialized to a file named “object.ser”. The FileOutputStream and ObjectOutputStream classes are used to write the serialized data to the file.