Spring Boot + MVC + JSP
Library Management System
In this tutorial, we are going to develop a small Library Management System.
Step 1 : Create a spring boot project and add following dependencies in your pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.3</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.javatrainingschool</groupId>
<artifactId>LibraryManagementApp</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>LibraryManagementApp</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
</dependency>
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Step 2 : application.properties file
spring.mvc.view.prefix=/WEB-INF/jsp/
spring.mvc.view.suffix=.jsp
server.port = 8081
spring.application.name= Library Management System
spring.datasource.driver-class-name=org.h2.Driver
spring.datasource.url=jdbc:h2:mem:bootapp;DB_CLOSE_DELAY=-1
spring.datasource.username=sa
spring.datasource.password=
spring.jpa.defer-datasource-initialization=true
spring.jpa.show-sql=true
spring.h2.console.enabled=true
Step 3 : Entity class Book.java
package com.javatrainingschool.entity;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Table;
@Entity
@Table(name = "book_111")
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private int id;
private String name;
private String author;
private int price;
private String status;
private String forDays;
//getters and setters
//constructors
//toString
}
Step 4 : Repository interface
package com.javatrainingschool.repo;
import java.util.List;
import org.springframework.data.repository.CrudRepository;
import com.javatrainingschool.entity.Book;
public interface BookRepository extends CrudRepository<Book, Integer> {
public List<Book> findByName(String name);
}
Step 5 : Controller class
package com.javatrainingschool.controller;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import com.javatrainingschool.entity.Book;
import com.javatrainingschool.repo.BookRepository;
@Controller
@RequestMapping("/library")
public class BookController {
@Autowired
private BookRepository bookRepo;
@RequestMapping("/home")
public String home(Model model) {
model.addAttribute("appName", "Java Training School Library");
return "home";
}
@RequestMapping("/display")
public String displayBookInfo(Model model) {
List<Book> allBooks = (List<Book>) bookRepo.findAll();
model.addAttribute("books", allBooks);
if(allBooks.isEmpty())
model.addAttribute("msg", "Unfortunately, the library is empty now. Let's"
+ "contribute by adding some books");
else {
if(model.getAttribute("msg") == null)
model.addAttribute("msg", "Welcome to Java Traning School Library");
}
return "displayBook";
}
@GetMapping("/borrowForm/{id}")
public String borrowForm(@PathVariable String id, Model model) {
Book book = bookRepo.findById(Integer.parseInt(id)).get();
model.addAttribute("book", book);
return "borrowBook";
}
@PostMapping("/borrow")
public String borrow(@RequestParam int id, Model model) {
Book book = bookRepo.findById(id).get();
book.setStatus("Borrowed");
bookRepo.save(book);
model.addAttribute("msg", "Book borrowed successfuly. Please return within a month's time");
return displayBookInfo(model);
}
@GetMapping("/addBookForm")
public String addBookForm(Model model) {
model.addAttribute("book", new Book());
return "addBook";
}
@PostMapping("/addBook")
public String addBook(@ModelAttribute("book") Book book, Model model) {
book.setStatus("available");
bookRepo.save(book);
model.addAttribute("msg", "Book added successfully. Thanks");
return displayBookInfo(model);
}
}
Step 6 : Create jsp files and keep them under webapp/WEB-INF/jsp folder as shown below
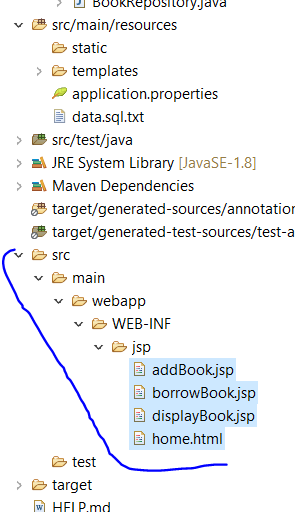
home.jsp
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<title>Home Page</title>
</head>
<body>
<h1>Hey, hope you are doing excellent today !</h1>
<p>
Welcome to spring boot app - <h3>${appName}</h3><br>
Would you like to pay a visit?
<a href="/library/display">Try Now</a>
</p>
</body>
</html>
displayBook.jsp file
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<%@ taglib uri="http://www.springframework.org/tags/form" prefix="form"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<html>
<body>
<h1>Welcome to Java Training School Library</h1><br>
<div> ${msg} </div><br>
<table border="2" width="70%" cellpadding="2">
<tr>
<th>Id</th>
<th>Book Name</th>
<th>Author</th>
<th>Price</th>
<th>Status</th>
<th>Borrow</th>
</tr>
<c:forEach var="book" items="${books}">
<tr>
<td>${book.id}</td>
<td>${book.name}</td>
<td>${book.author}</td>
<td>${book.price}</td>
<td>${book.status}</td>
<c:if test="${book.status == 'available'}">
<td><a href="borrowForm/${book.id}">Borrow</a></td>
</c:if>
</tr>
</c:forEach>
</table>
<br />
<a href="addBookForm">Add a Book</a>
</body>
</html>
addBook.jsp
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<%@ taglib uri="http://www.springframework.org/tags/form" prefix="form"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<html>
<body>
<h1>Add Book</h1>
<form:form method="post" action="addBook" modelAttribute="book">
<table>
<tr>
<td>Book Name :</td>
<td><form:input path="name" /></td>
</tr>
<tr>
<td>Author :</td>
<td><form:input path="author" /></td>
</tr>
<tr>
<td>Price :</td>
<td><form:input path="price" /></td>
</tr>
<tr>
<td></td>
<td><input type="submit" value="Save Book" /></td>
</tr>
</table>
</form:form>
</body>
</html>
borrowBook.jsp
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<%@ taglib uri="http://www.springframework.org/tags/form" prefix="form"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<html>
<body>
<h1>Borrow book</h1>
<form:form method="post" action="/library/borrow">
<table border="2" width="70%" cellpadding="2">
<tr>
<th>Id</th>
<th>Book Name</th>
<th>Author</th>
<th>Price</th>
<th>Status</th>
</tr>
<tr>
<td>${book.id}</td>
<td>${book.name}</td>
<td>${book.author}</td>
<td>${book.price}</td>
<td>${book.status}</td>
</tr>
</table>
<br /> <input type="submit" value="Borrow">
<input type="hidden" name="id" value="${book.id}" />
</form:form>
</body>
</html>
Step 7 : Run and test the application
home url – http://localhost:8081/library/home
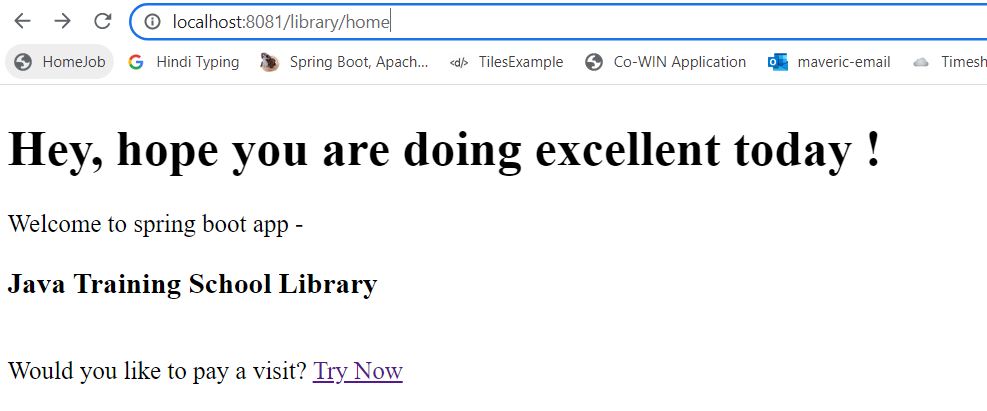
__________________________________________________________________________________________________________
displaybook page
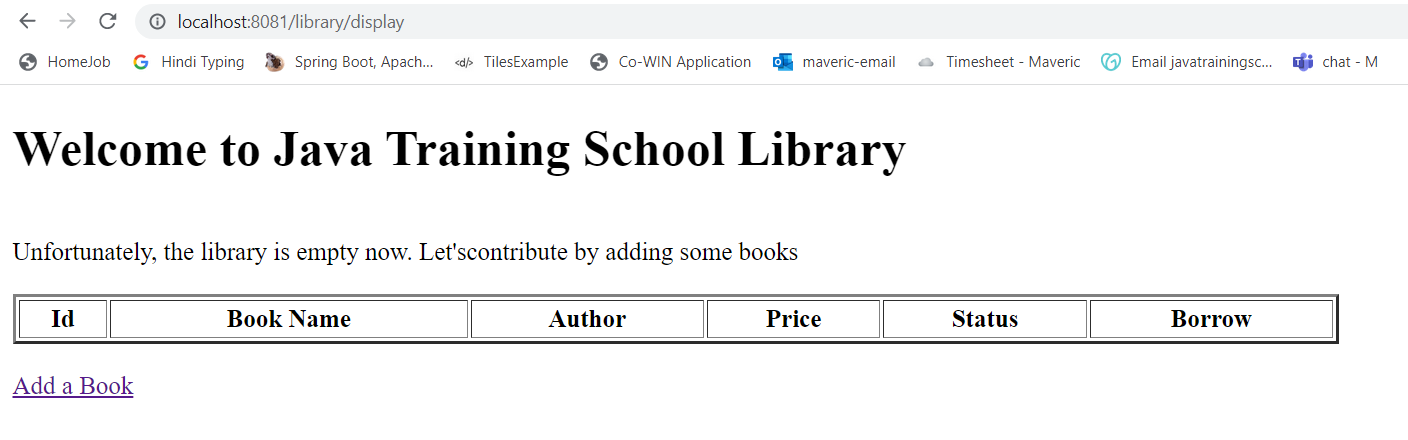
__________________________________________________________________________________________________________
addBook page
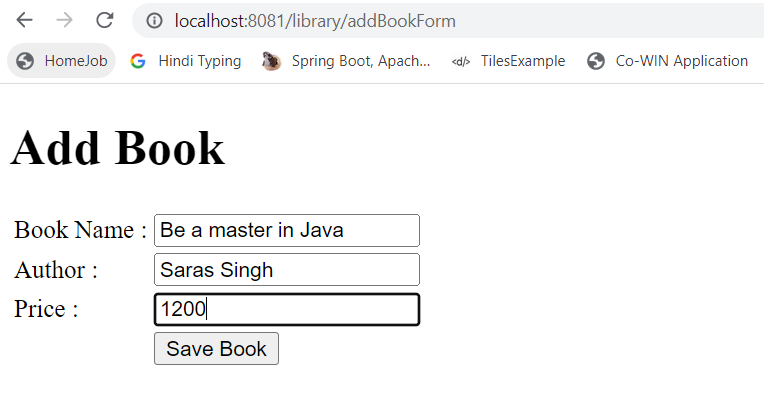
__________________________________________________________________________________________________________
After add book is successful
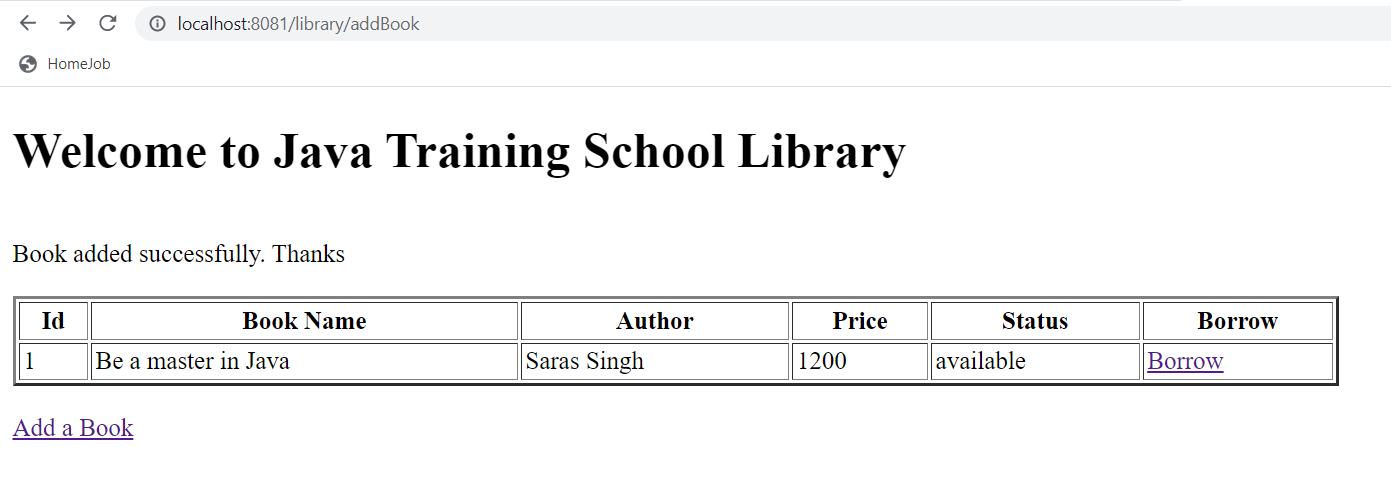
__________________________________________________________________________________________________________
Borrow a book page
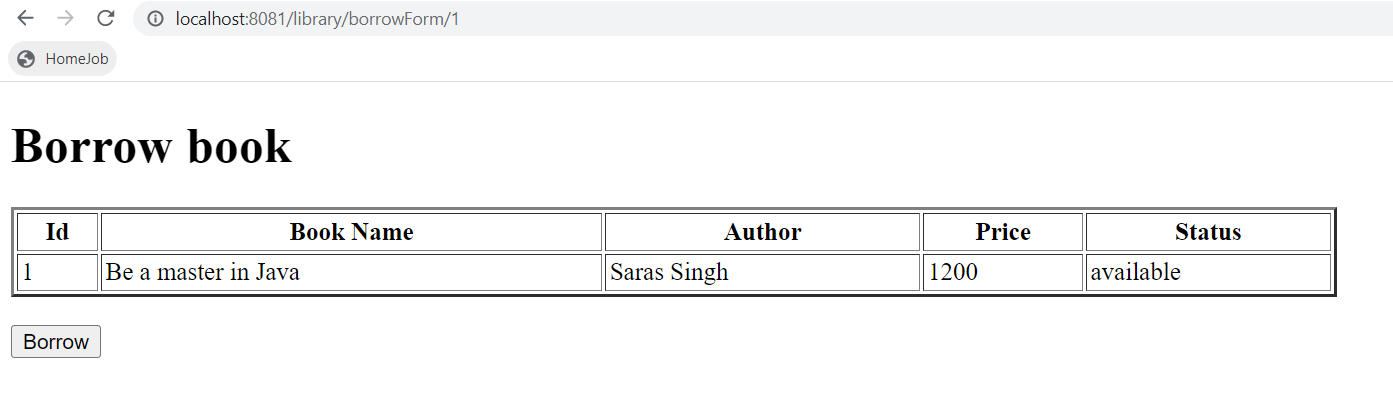
__________________________________________________________________________________________________________
After borrowing is successful
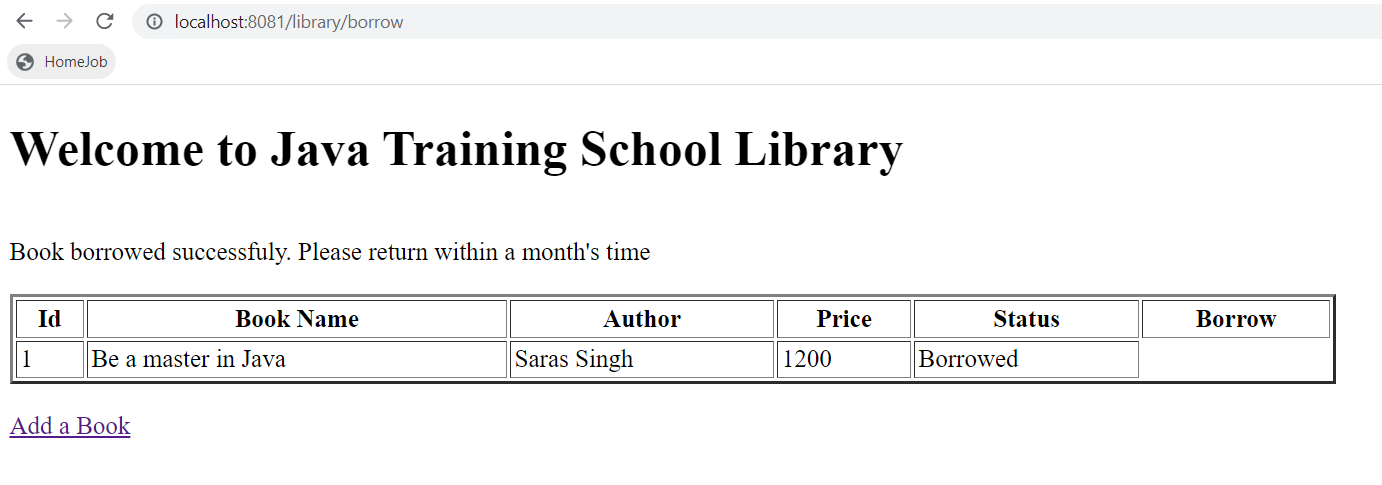
__________________________________________________________________________________________________________
After adding multiple books, display page would look like below
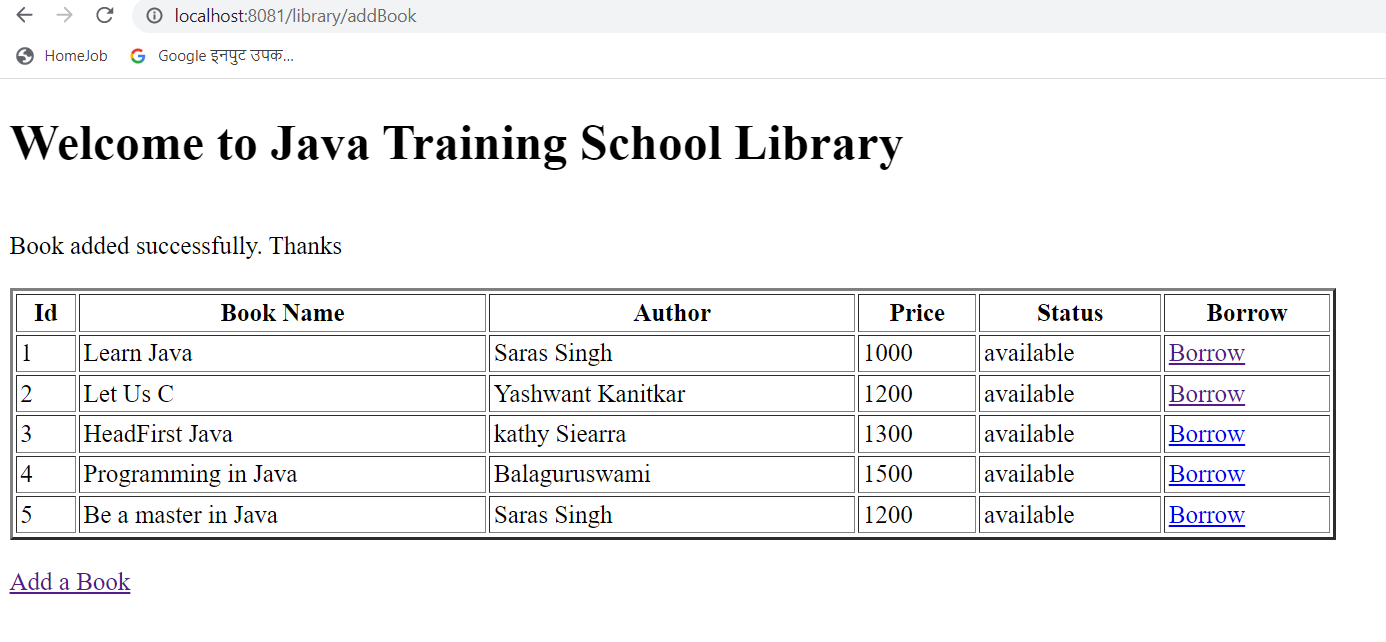