Creating File in Java
We can create files by using createNewFile() method. This method returns a boolean type, true or false.
If the file is successfully created, it will return true otherwise, it will return false.
Before creating file we need to make File class object. Because createNewFile() method is defined inside File class.
File fileObj = new File("javatrainingschool.txt");
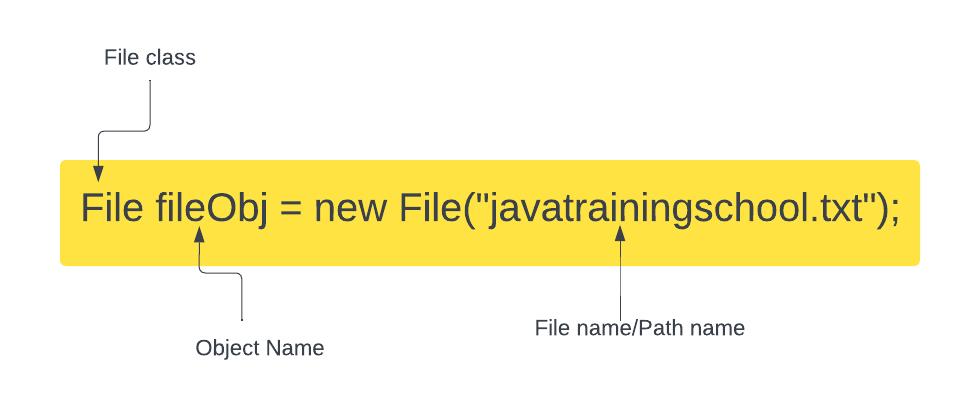
Imp*
1. Above line would not create any physical file, first it will check is ther any physical File already
available with javatrainingschool.txt name or not.
2. If it is already available then fileObj pointing to that file.
3. If it is not already available this line would not crate any physicalfile and just it creates a java file object to represent the name javatrainingschool.txt.
CreateNewFile.Java
import java.io.File;
import java.io.IOException;
public class CreateNewFile {
public static void main(String[] args) {
File fileObj = new File("javatrainingschool.txt");
System.out.println(fileObj.exists());
//If file does not exists output will be false.
try {
fileObj.createNewFile(); //calling createNewFile() methods
} catch (IOException e){
e.printStackTrace();
}
//File has been created successfully
System.out.println(fileObj.exists());
//Now file exists so output will be true.
}
}
Output
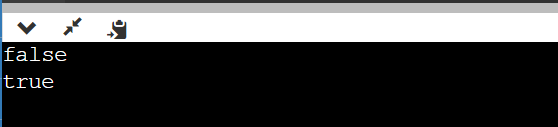
To create a file in a certain directory (which requires permission),provide the file’s path and enclose the ” “ character in double backslashes (for Windows). You can just type the path on Mac and Linux, such as: /Users/name/filename.txt.
File fileObj = new File("C:\\Users\\MyName\\javatrainingschool.txt");
Now go and checkout in specified directory whether a file named javatrainingschool is created successfully.
Get File Information
After creating a file, we can get some information about the file. To get information about a file like its name, absolute path, whether it is readable and writeable, and length, we use File class methods-(click here).
Following is a demonstration of getting file information using several methods.
getInfo.java
import java.io.File; //Importing the File class
public class getInfo {
public static void main(String[] args) {
// Creating file object
File fileObj = new File("javatrainingschool.txt");
// Creating a file if it is not already exists.
try {
fileObj.createNewFile();
}
catch(Exception e) {
System.out.print("File not Created.");
}
System.out.println("The name of the file is: " + fileObj.getName());
// Getting absolute path of the file
System.out.println("The absolute path of the file is: " + fileObj.getAbsolutePath());
// Checking whether the file is writeable or not
System.out.println("Is file writeable?: " + fileObj.canWrite());
// Checking whether the file is readable or not
System.out.println("Is file readable " + fileObj.canRead());
// Getting the length of the file in bytes
System.out.println("The size of the file in bytes is: " + fileObj.length());
}
}
Output
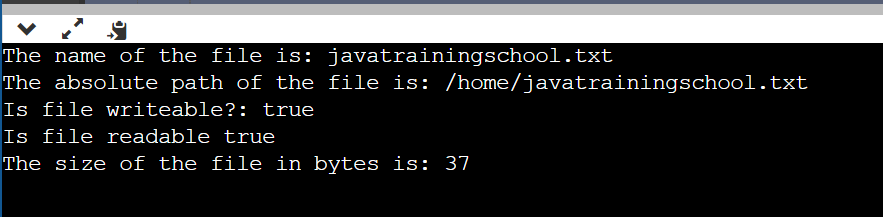
javatrainingschool.txt
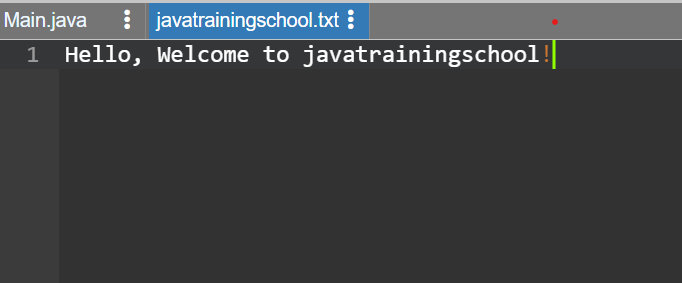
Read the comments very carefully; you will understand the code properly.
Working with Directory in Java
Java provides facility to make directory in our system by writing code. File class having mkdir() methods which is used to create a directory.
createDirectory.java
//importing File class
import java.io.File;
public class createDirectory
{
public static void main(String[] args) {
File dirObj = new File("newDir");
dirObj.mkdir();
//creating new directory with name 'newDir'
File fileObj = new File("newDir","jts.txt");
//File fileObj = new File(dirObj,"jts.txt");
try{
fileObj.createNewFile();
//creating jts.txt in specified directory 'newDir'
System.out.print("File has created in specified Directory!");
}
catch(Exception e){
System.out.print("file not created!");
}
}
}
Output :
File has created in specified Directory!