JDBC Connectivity Example with MySQL
In the below tutorial, we will connect to mysql database. So, we will need my sql dependency.
Step 1 : You need to have a dependency in your java project – mysql-connector-java-8.0.30.jar
maven dependency
<!-- https://mvnrepository.com/artifact/mysql/mysql-connector-java -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.30</version>
</dependency>
Step 2 : Create a table ‘Cars’ in the database and insert some records as below
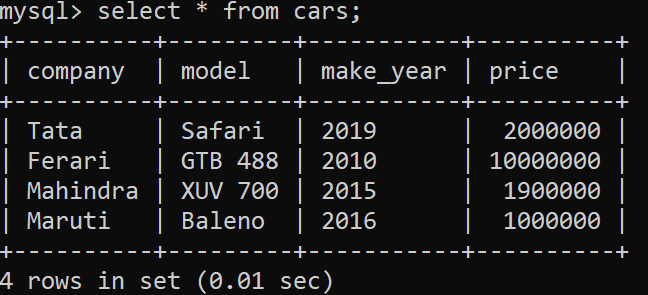
Step 3 : Main class
package com.sks;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class MainClass {
public static void main(String[] args) throws SQLException, ClassNotFoundException {
// Step 1 : Load the mysql jdbc driver
Class.forName("com.mysql.cj.jdbc.Driver");
Connection con = null;
// Step 2 : Getting Connection Object
con = DriverManager.getConnection("jdbc:mysql://localhost:3306/my_test_db", "root", "abcd");
Statement stmt = null;
// Step 3: Getting statement object
stmt = con.createStatement();
// Step 4 : Executing the query
String query = "select * from cars";
ResultSet rs = null;
rs = stmt.executeQuery(query);
while (rs.next()) {
System.out.println("Company : " + rs.getString("company"));
System.out.println("Model : " + rs.getString("model"));
System.out.println("Make Year : " + rs.getString("make_year"));
System.out.println("Price : " + rs.getDouble("price"));
System.out.println("_____________________________________");
}
rs.close();
stmt.close();
con.close();
}
}
Output :
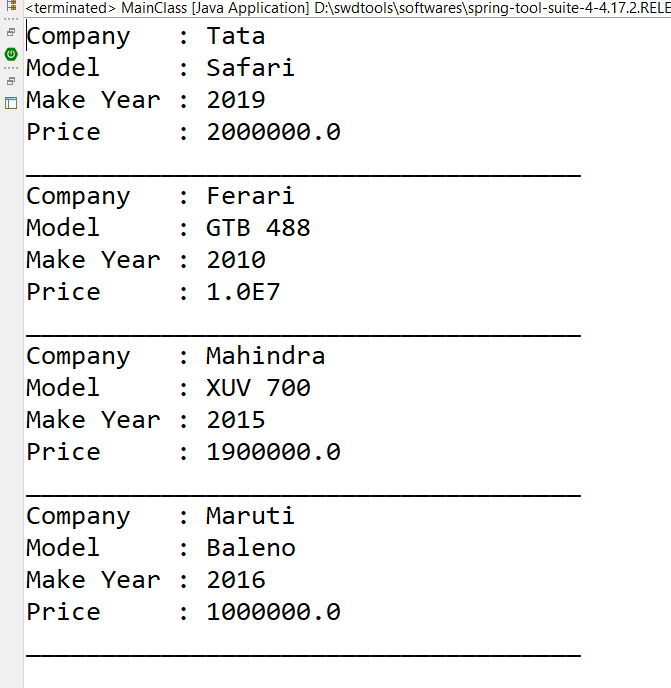