Spring Boot with Mongo DB
In this tutorial, we will be learning how to integrate a spring boot application to Mongo DB cloud using your Mongo DB Atlas account.
Prerequisite
You must have a Mongo DB Atlas account. If not, create one using the below link.
https://www.mongodb.com/cloud/atlas/register
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.7</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.jts</groupId>
<artifactId>boot-with-mongo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>boot-with-mongo</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
application.properties file
spring.data.mongodb.uri=mongodb+srv://<user-name>:<password>@xyzcluster.jwvsbo8.mongodb.net/?retryWrites=true&w=majority
spring.data.mongodb.database=my_db
The above property can be found on your Mongo DB Atlas account as shown below
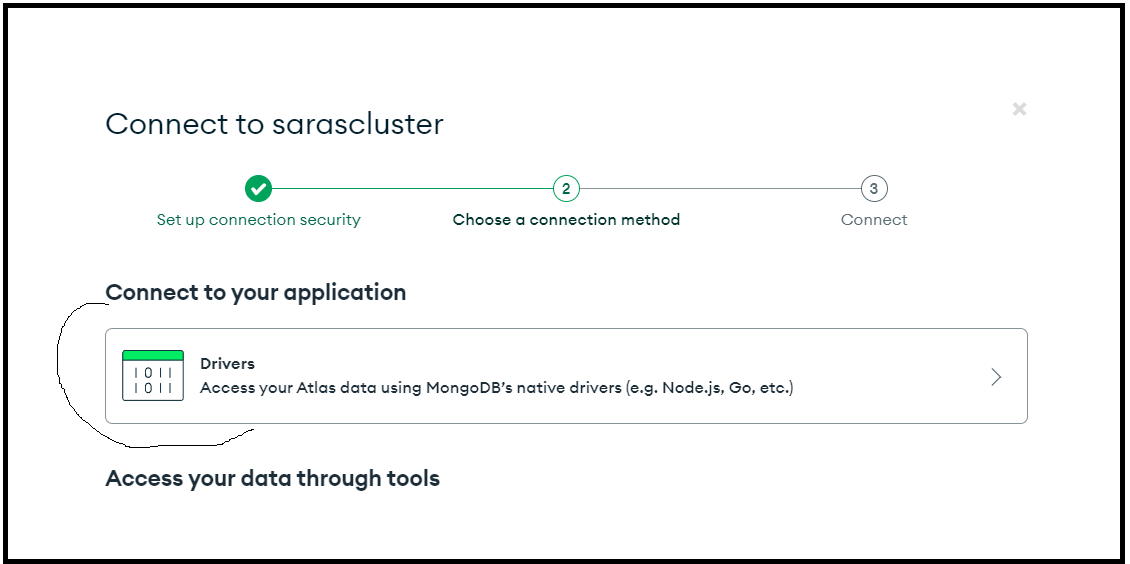
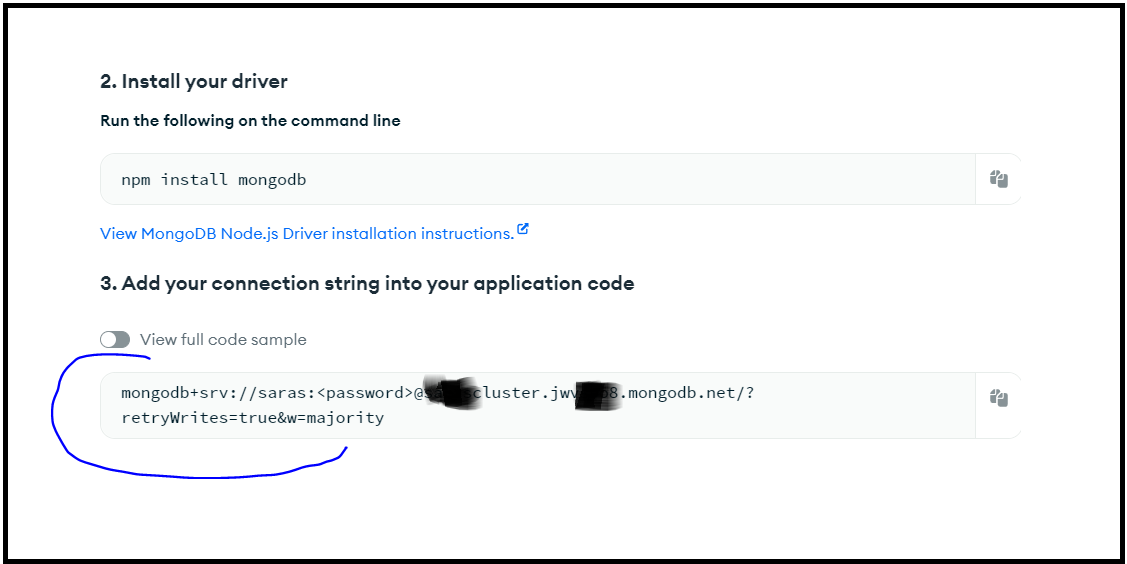
application.properties file for local mongo db
If you have mongo database running on your local machine, you can use below properties.
#Local MongoDB
spring.data.mongodb.authentication-database=admin
spring.data.mongodb.username=root
spring.data.mongodb.password=pwd
spring.data.mongodb.database=my_db
spring.data.mongodb.port=27017
spring.data.mongodb.host=localhost
Student.java
package com.example.demo;
import java.util.Date;
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;
@Document
public class Student {
@Id
private String id;
private String name;
private Date creationDate = new Date();
//getter and setter methods
//constructors
//toString method
}
StudentRepository.java
package com.example.demo;
import org.springframework.data.mongodb.repository.MongoRepository;
public interface StudentRepository extends MongoRepository<Student, String>{
}
StudentController.java
package com.example.demo;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping(value = "/student")
public class StudentController {
@Autowired
private StudentRepository studentRepository;
@RequestMapping(value = "/all", method = RequestMethod.GET)
public List<Student> getAllStudent() {
System.out.println("Fetching students data");
return studentRepository.findAll();
}
@RequestMapping(value = "/create", method = RequestMethod.POST)
public Student addNewStudent(@RequestBody Student student) {
return studentRepository.save(student);
}
}
Retrieve all the students information
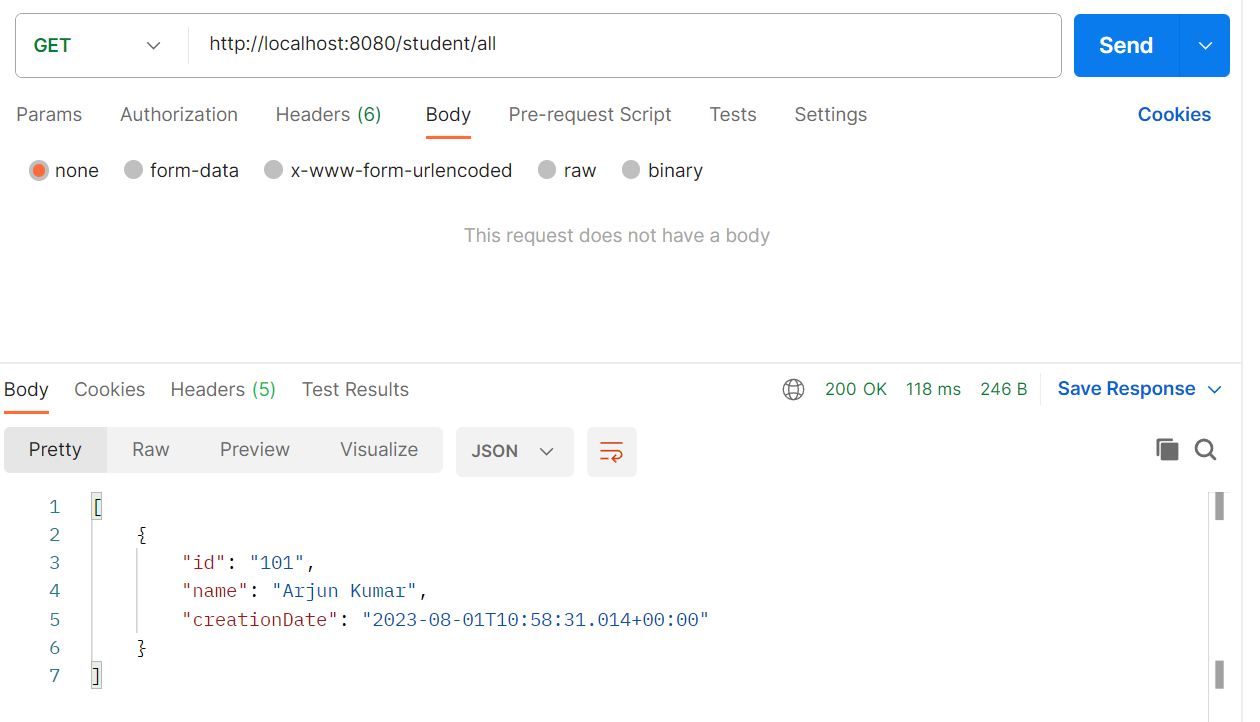
Create a student
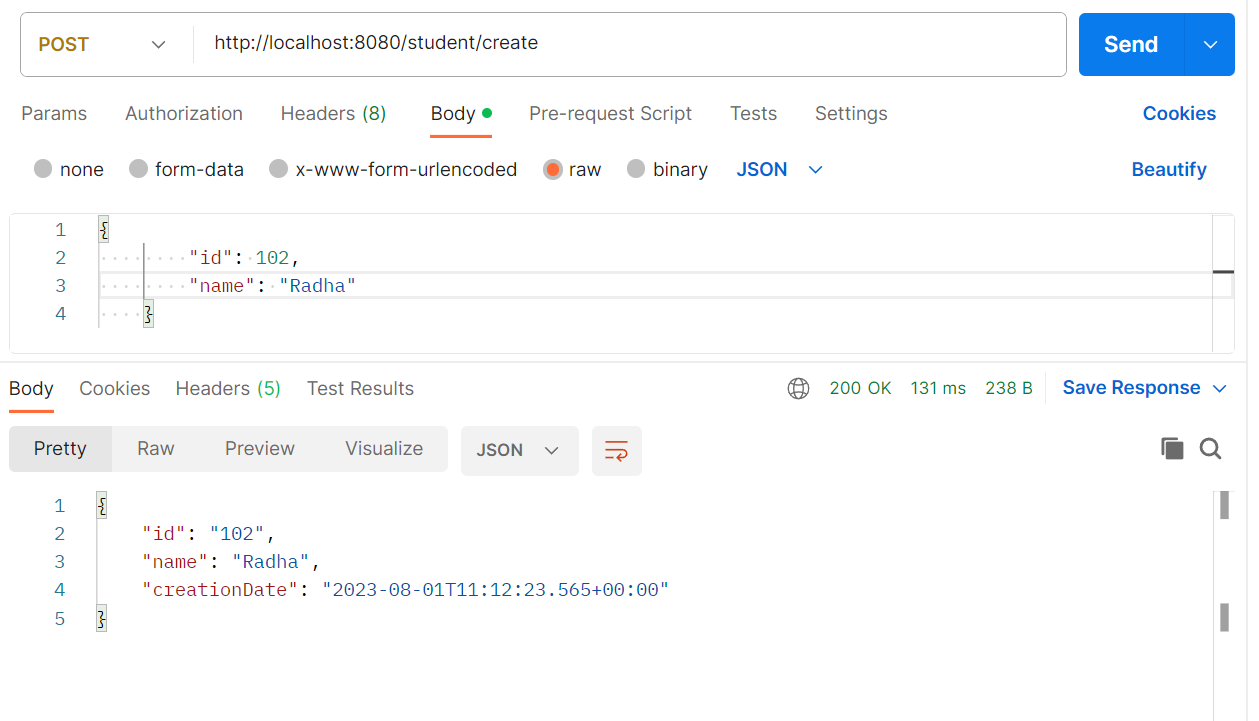