StringBuffer
StringBuffer class is similar to String class except that strings created using StringBuffer objects are mutable( modifiable).
- StringBuffer objects are mutable
- Multiple StringBuffer operations modify the same object
- StringBuffer objects are thread-safe like String objects
How to create a StringBuffer object
- In the first way, it can be created using the new keyword
StringBuffer obj = new StringBuffer("Meera");
Create StringBuffer object from String object
String s = "Meera";
StringBuffer obj = new StringBuffer(s);
Create String object from StringBuffer object
StringBuffer obj = new StringBuffer("Meera");
String s = obj.toString();
StringBuffer Example for append method
package com.javatrainingschool;
public class StringBufferExample {
public static void main(String[] args) {
StringBuffer sb = new StringBuffer("Anjali");
sb.append(" Kumar");
System.out.println(sb);
}
}
Output:
Anjali Kumar
StringBuffer example2
package com.javatrainingschool;
public class StringBufferExample {
public static void main(String[] args) {
StringBuffer sb = new StringBuffer("Anjali");
sb.append(" Kumar");
System.out.println(sb);
//Displays the current capacity of the string buffer object
System.out.println(sb.capacity());
//deletes the character from index 2 to 5(excluding)
sb.delete(2, 5);
System.out.println(sb);
}
}
Output:
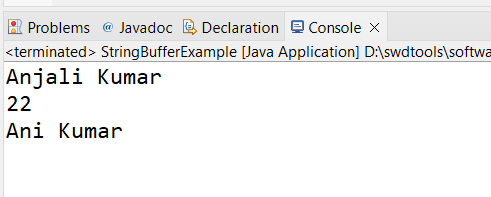
Reverse a String using StringBuffer’s reverse method
package com.javatrainingschool;
public class StringBufferExample {
public static void main(String[] args) {
String s = "Kishor Kumar";
System.out.println("Original String : " + s);
StringBuffer sb = new StringBuffer(s);
sb.reverse();
s = sb.toString();
System.out.println("Reversed String : " + s);
}
}
Output:
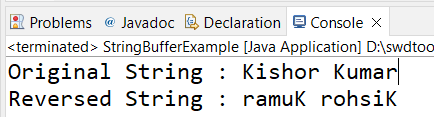
Conlusion:
From the above examples, it is clear that which operations we do on StringBuffer objects affect the original string. It is not true in case of String objects. Each operation on String object creates a new String object altogether.
When to use StringBuffer instead of String
When there are repeated operations like concatenation, replace, delete, insert etc on the string objects, it is better to go with StringBuffer class rather than the String class.