FilterConfig in Servlet
If we want to pass some initial parameter to a filter then it can be done using web.xml. And to get these parameters in the filter, FilterConfig object can be used.
Note -> Just like to get initial params information in the servlets, we use ServletConfig object, similarly, we have FilterConfig object to get initial params information in filters.
Note -> FilterConfig object is analogous to ServletConfig object
FilterConfig methods
Method | Description |
---|---|
public void init(FilterConfig config) | Called once to initialize the filter |
public String getInitParameter(String parameterName) | Gets the value of the parameter against the passed parameter name |
public java.util.Enumeration getInitParameterNames() | Gets all the init parameters |
public ServletContext getServletContext() | Returns ServletContext object |
FilterConfig Example
In this example, we will pass one init parameter to the fiter named ‘environment‘ in the web.xml. In the filter code, we will check if the value of environment is test, we will give message – This app is a test app. Don’t deploy it on PROD. If the value of environment is Prod, we will take the request to the servlet and print the message from the servlet – Welcome to my servlet.
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
</head>
<body>
<a href="configTestServlet">Click here to test filter config</a>
</body>
</html>
ConfigTestFilter.java
package com.sks.filter;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
public class ConfigTestFilter implements Filter {
private FilterConfig config;
public void init(FilterConfig config) throws ServletException {
this.config = config;
}
public void doFilter(ServletRequest req, ServletResponse resp, FilterChain chain)
throws IOException, ServletException {
PrintWriter out = resp.getWriter();
String env = config.getInitParameter("environment");
if (env.equalsIgnoreCase("test")) {
out.print("This app is a test app. Don't deploy it on PROD");
} else if (env.equalsIgnoreCase("prod")){
chain.doFilter(req, resp);// forward the request to next resource
}
}
public void destroy() {
}
}
ConfigTestServlet.java
package com.sks;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ConfigTestServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.print("<br>Welcome to my servlet<br>");
}
}
web.xml
<web-app>
<servlet>
<servlet-class>com.sks.ConfigTestServlet</servlet-class>
<servlet-name>configTestServlet</servlet-name>
</servlet>
<servlet-mapping>
<servlet-name>configTestServlet</servlet-name>
<url-pattern>/configTestServlet</url-pattern>
</servlet-mapping>
<filter>
<filter-class>com.sks.filter.ConfigTestFilter</filter-class>
<filter-name>configTestFilter</filter-name>
<init-param>
<param-name>environment</param-name>
<param-value>prod</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>configTestFilter</filter-name>
<url-pattern>/configTestServlet</url-pattern>
</filter-mapping>
</web-app>
Deploy and test the application
When the value of environment is PROD in web.xml
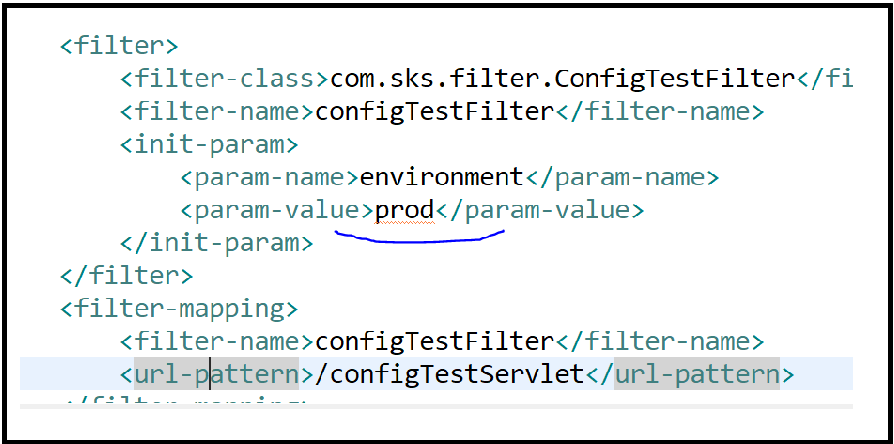
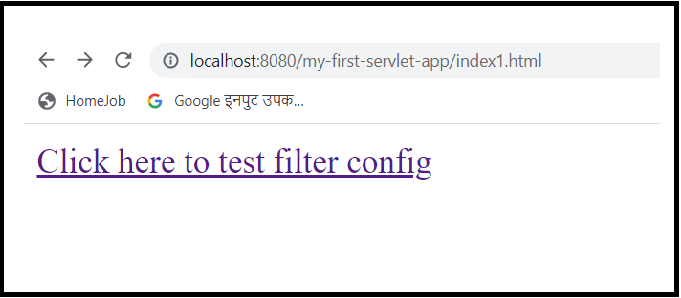
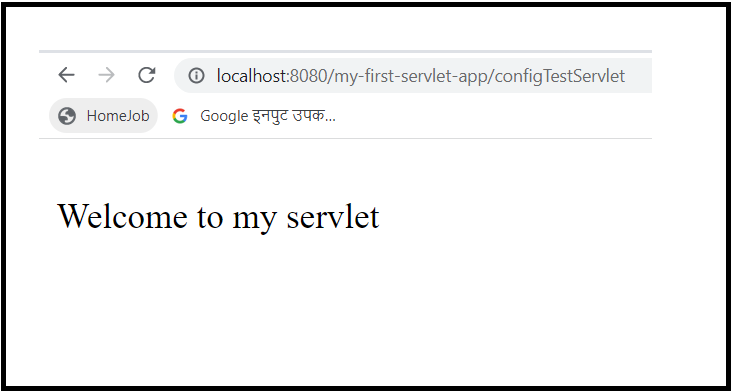
When the value of environment is Test, the output will be like below
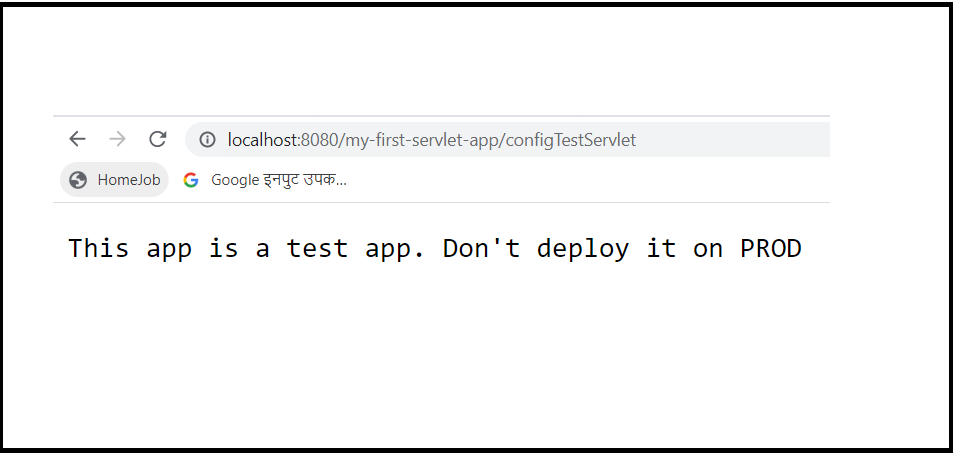