Exceptions and overriding
Method overriding has certain rules for exceptions. Let’s see what those are.
For quick look, you may refer the below table
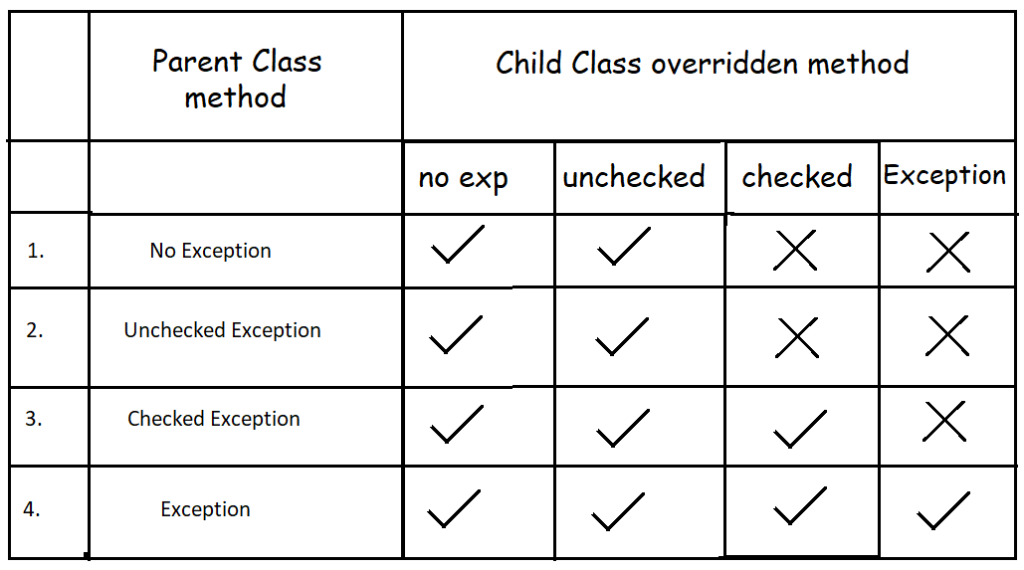
Note -> Child class’ overridden method can narrow down the exception from parent class’ method. But it cannot widen the same.
Scenario | Parent Class | Child Class |
---|---|---|
1. | Parent’s method doesn’t throw any exception | -> Overridden method can throw no exception -> May throw only unchecked exceptions -> Cannot throw checked exceptions |
2. | Parent’s method throws unchecked exception | -> Overridden method can throw no exception -> May throw the same unchecked exception -> May throw any other unchecked exception -> Cannot throw checked exceptions |
3. | Parent’s method throws checked exception | -> Overridden method can throw no exception -> May throw the same checked exception ->May throw any subclass of the checked exception thrown by the parent class -> May throw any unchecked exception |
4. | Parent’s method throws Exception class | -> Overridden method can throw no exception -> May throw any exception class (checked or unchecked) including Exception class |
Case 1 – When parent class method doesn’t throw an exception (Compiler error)
public class Parent {
public void display() {
System.out.println("Parent's display");
}
}
import java.io.IOException;
public class Child extends Parent {
@Override
public void display() throws IOException { //x compilation error
System.out.println("Child's display");
}
}
Case 2 – when parent class throws an exception and child also throws same exception (success scenario)
import java.io.IOException;
public class Parent {
public void display() throws IOException {
System.out.println("Parent's display");
}
}
import java.io.IOException;
public class Child extends Parent {
@Override
public void display() throws IOException {
System.out.println("Child's display");
}
}
Case 3 – when parent class throws an exception and child throws subclass exception (success scenario)
public class Parent {
public void display() throws Exception {
System.out.println("Parent's display");
}
}
import java.io.IOException;
public class Child extends Parent {
@Override
public void display() throws IOException {
System.out.println("Child's display");
}
}
Case 4 – when parent class throws an exception and child throws superclass exception (failed scenario)
import java.io.IOException;
public class Parent {
public void display() throws IOException {
System.out.println("Parent's display");
}
}
public class Child extends Parent {
@Override
public void display() throws Exception { //X compilation error
System.out.println("Child's display");
}
}