HashSet of Custom Objects in Java
Consider the below example and guess what will be the size of the set (output of the program)
Teacher.java
package com.sks;
public class Teacher {
private String name;
private String subject;
//getters and setters
//constructors
//tostring
}
CustomSet.java
package com.sks;
import java.util.HashSet;
import java.util.Set;
public class CustomSetExample {
public static void main(String[] args) {
Set<Teacher> set = new HashSet<>();
set.add(new Teacher("Anjali", "English"));
set.add(new Teacher("Hari", "Mathematics"));
set.add(new Teacher("Javed", "Hindi"));
set.add(new Teacher("Diana", "English"));
set.add(new Teacher("Diana", "English"));
System.out.println("Size = " + set.size());
}
}
Can you guess the output of the above program
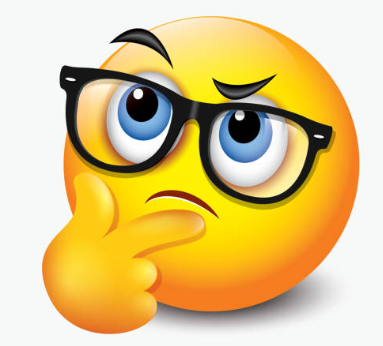
Most of you will answer it as 4, but you’re wrong. The output here would be 5. Let’s find out the reason.
We have learnt that set doesn’t allow duplicates. So, how come it is doing so in this example? The reason is, it is not considering two objects with same values ‘Diana’ and ‘English’ as same. Because the condition of equality for two objects may vary from person to person. Some people may say only if the name is same, the two objects are same. Some people may say if name and subject both are same, then only the two objects are same.
Thus, the condition for equality of two objects is to be given by the developer. For that, he or she needs to override two methods from Object class namely equals() and hashCode().
In this particular example, we’re saying that if values of all the properties of the two objects i.e. name, subject are same, then the objects will be treated as equal objects.
Good news is that you don’t have to write implementations equals() and hashCode(). Your IDE can do that for you. Refer the screenshots
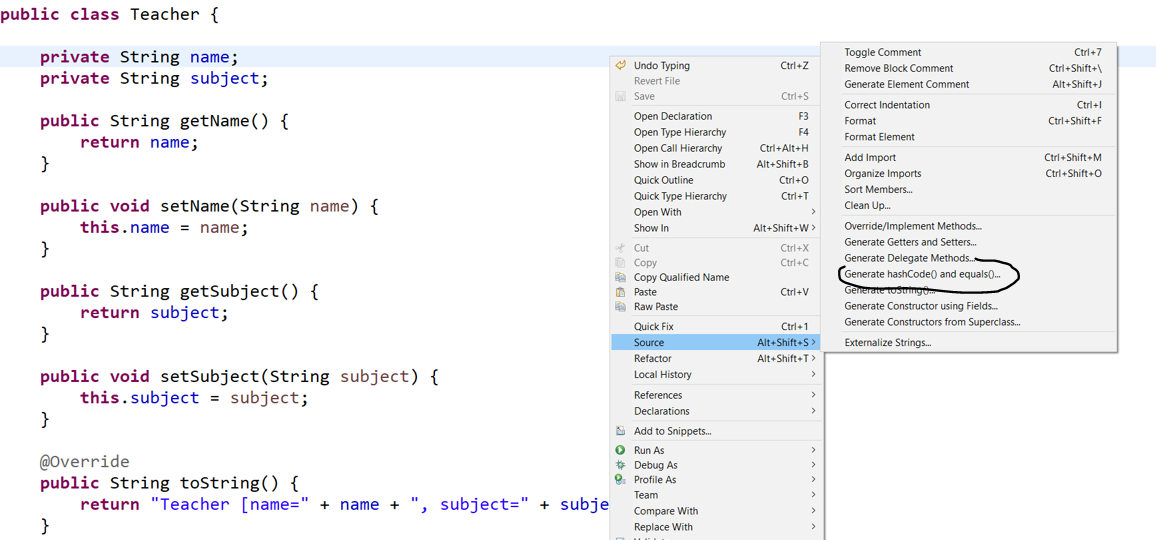
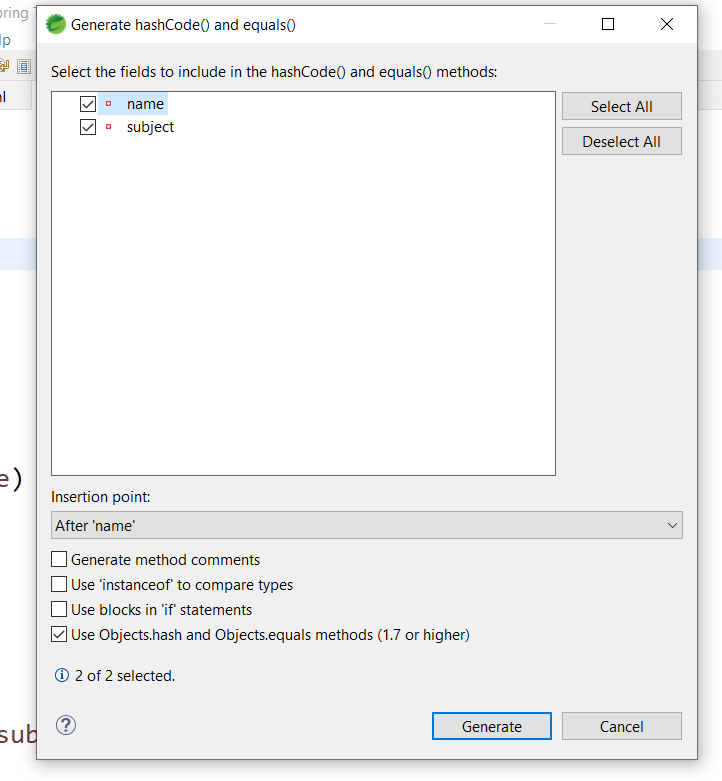
Refer the below code after equals and hashcode methods are generated
package com.sks;
import java.util.Objects;
public class Teacher {
private String name;
private String subject;
//getters and setters
//constructors
//toString
@Override
public int hashCode() {
return Objects.hash(name, subject);
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Teacher other = (Teacher) obj;
return Objects.equals(name, other.name) && Objects.equals(subject, other.subject);
}
}
Now, if you again run the below code, you will get the desired output. Where Diana, English will be stored only once in the hashset
package com.sks;
import java.util.HashSet;
import java.util.Set;
public class CustomSetExample {
public static void main(String[] args) {
Set<Teacher> set = new HashSet<>();
set.add(new Teacher("Anjali", "English"));
set.add(new Teacher("Hari", "Mathematics"));
set.add(new Teacher("Javed", "Hindi"));
set.add(new Teacher("Diana", "English"));
set.add(new Teacher("Diana", "English"));
System.out.println("Size = " + set.size());
}
}
Output:
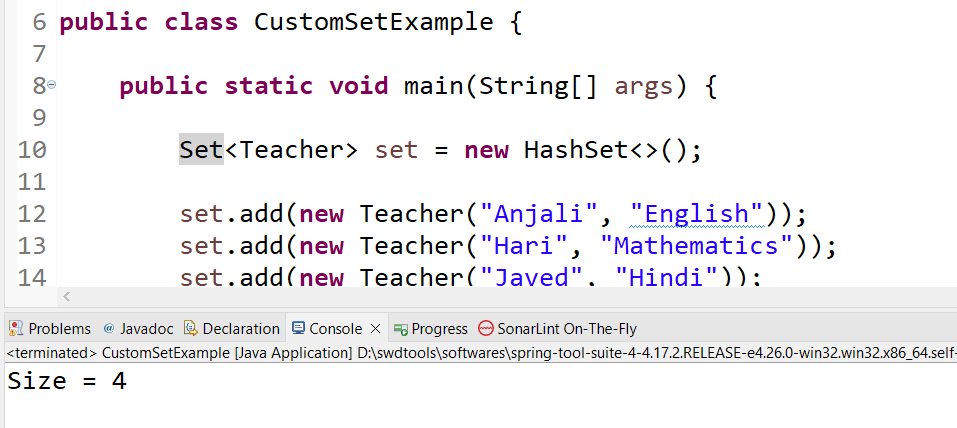
Thus learning from this lesson is that if you’re using custom objects in a set, then make sure that you override equals() and hashCode() methods.