ServletContext Object
- ServletContext object is created by the servlet container
- ServletContext object is contained within ServletConfig object
- ServletContext object is used to get configuration information from web.xml file
- There is one ServletContext object per application
Note -> If some information needs to be shared across all the servlets in the application, then it can be stored in ServletContext object.
ServletContext commonly used methods
Method | Description |
---|---|
public String getInitParameter(String name) | Gives the value of the passed parameter |
public Enumeration getInitParameterNames() | Gives all the initialization parameters |
public void setAttribute(String name,Object object) | Sets the value of an attribute |
public Object getAttribute(String name) | Gets the value of the passed attribute |
public void removeAttribute(String name) | Removes the passed attribute |
How to define servlet context parameters in web.xml
Context parameters are defined in web.xml under the <web-app> element. Refer the below diagram. Here three context parameters are defined
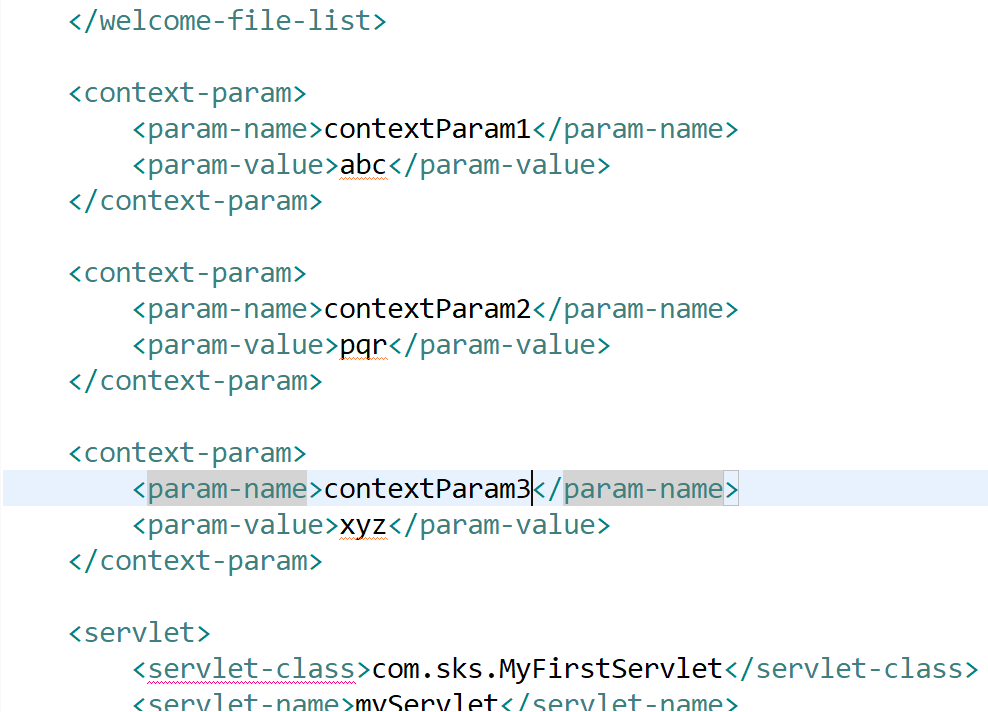
How to get ServletContext object
ServletContext context = getServletContext();
or
ServletContext context = getServletConfig().getServletContext();
ServletContext Example
package com.sks;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ServletContextExample extends HttpServlet {
public void doGet(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException {
res.setContentType("text/html");
PrintWriter writer = res.getWriter();
//Getting ServletContext object
ServletContext context = getServletContext();
// Getting the value of the initialization parameter and printing it
String contextParam1 = context.getInitParameter("contextParam1");
writer.println("Context parameter contextParam1 is = " + contextParam1);
writer.close();
}
}
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
id="WebApp_ID" version="3.0">
<display-name>my-first-servlet-app</display-name>
<context-param>
<param-name>contextParam1</param-name>
<param-value>abc</param-value>
</context-param>
<context-param>
<param-name>contextParam2</param-name>
<param-value>pqr</param-value>
</context-param>
<context-param>
<param-name>contextParam3</param-name>
<param-value>xyz</param-value>
</context-param>
<servlet>
<servlet-class>com.sks.ServletContextExample</servlet-class>
<servlet-name>myServlet</servlet-name>
</servlet>
<servlet-mapping>
<servlet-name>myServlet</servlet-name>
<url-pattern>/my</url-pattern>
</servlet-mapping>
</web-app>
Example to get all the parameters
package com.sks;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Enumeration;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ServletContextExample extends HttpServlet {
public void doGet(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException {
res.setContentType("text/html");
PrintWriter writer = res.getWriter();
//Getting ServletContext object
ServletContext context = getServletContext();
Enumeration<String> contextParams = context.getInitParameterNames();
String str = "";
writer.print("Printing the context initialization parameters : ");
while(contextParams.hasMoreElements()){
str = contextParams.nextElement();
writer.print("<br> " + context.getInitParameter(str));
}
writer.close();
}
}
Run the application and check the output
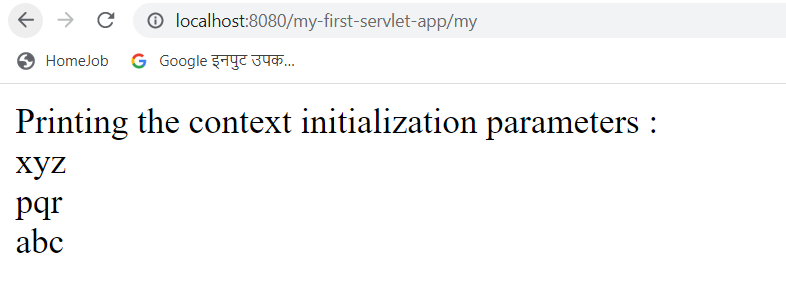