How to compare Strings In Java
String comparison is one of the most frequently used operation in java programming language. But if not understood correctly, developers make mistakes while comparing two String objects.
There can be two things which can be compared for String objects: The reference and the content. Look at the below diagram to understand it better.
String s1 = “Shankar”;
When we create an object of String, there are two things created: the reference variable and the object itself
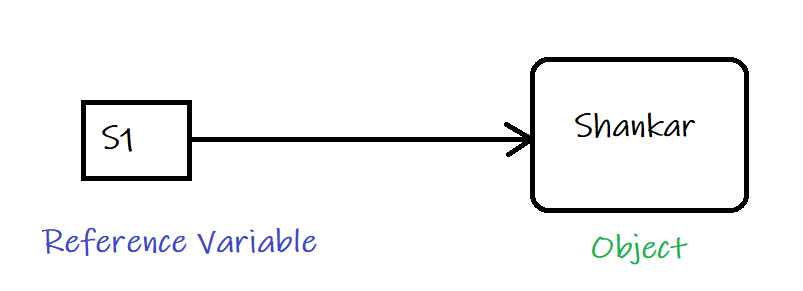
Let’s create another object
String s2 = “Mark”;
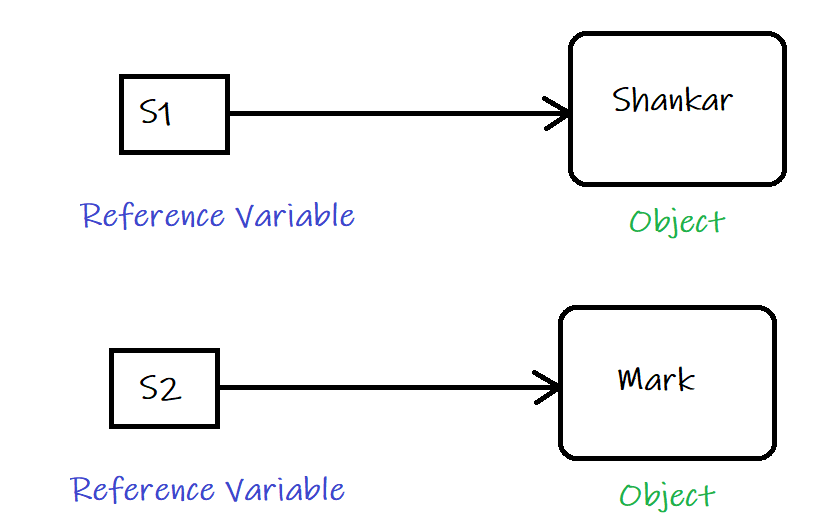
It is clear from the diagram that there are two things that can be compared: The value of reference(s1 and s2) and the content of the object (Shankar and Mark).
In order to compare the values of two reference variables, we need to use == operator; whereas to compare the contents of two objects, we need to use .equals() method.
Comparing String objects using == operator
When we use == operator between two String objects, it compares the reference variables of String objects. It returns true if the value of two reference variables are equal.
String s1 = "Shankar";
String s2 = "Mark";
boolean result;
result = (s1 == s2);
In the above example we are comparing the two references s1 and s2. So, what will be the output? To understand it, we need to understand what are the values held by s1 and s2.
We have understood when we create String objects as literals, they are created in String Constant Pool.
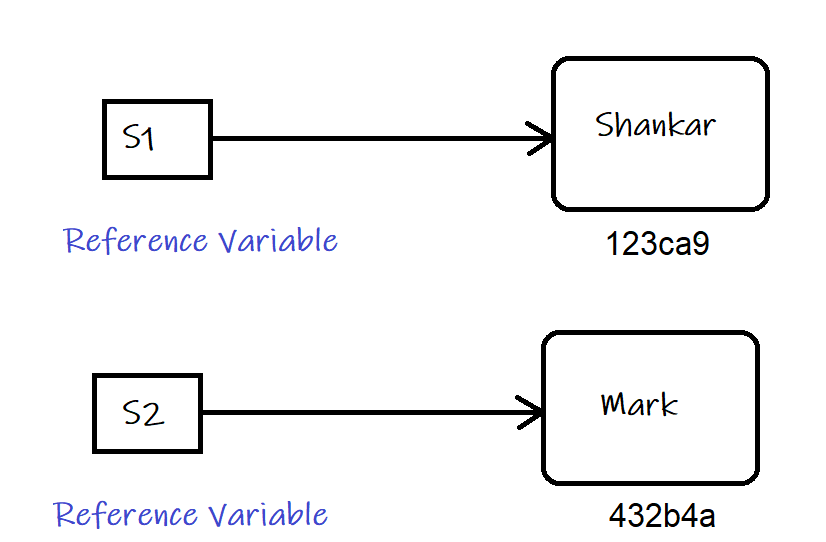
It is clear from the above diagram that s1 and s2 have different memory address values 123ca9 and 432b4a respectively. So, the comparison s1 == s2 will return false.
Now, consider below example
String s1 = "Shankar";
String s2 = "Shankar";
boolean result;
result = (s1 == s2);
Here, since Shankar is already created in String Constant Pool, s2 will not create a new object, rather it will start pointing to the existing object as explained in the below diagram.
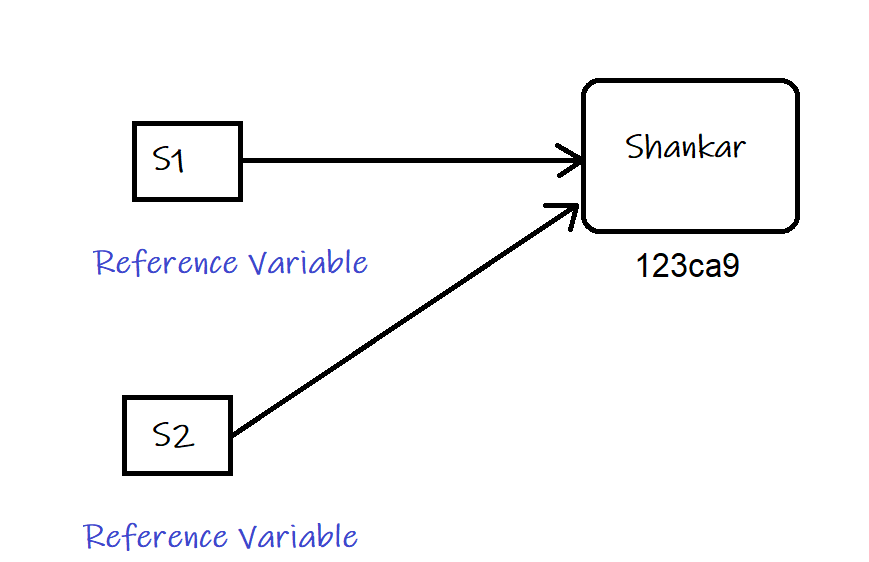
Now, s1 and s2 both have the memory address value as 123ca9. Thus the comparison, s1 == s2 will return true.
Note -> == operator returns true only when two reference variables point to the same object, otherwise it returns false.
String comparison with == example
package com.javatrainingschool;
public class DoubleEqualToComparison {
public static void main(String[] args) {
String s1 = "Shankar";
String s2 = "Mark";
System.out.println(s1 == s2); //false
String s3 = "Mark";
System.out.println(s2 == s3); //true
}
}
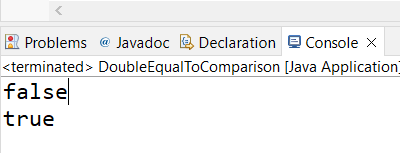
== comparision for String literal and New keyword
In the below example, the comparison returns false. Even though the content is same. Because s1 object is created in String constant pool, whereas s2 object is created in HEAP. Thus memory addresses are different
package com.javatrainingschool;
public class DoubleEqualToComparison {
public static void main(String[] args) {
String s1 = "Shankar";
String s2 = new String("Shankar");
System.out.println(s1 == s2);
/*
* The above comparison returns false. Even though the content is same. Because
* s1 object created in String constant pool, whereas s2 object is created in HEAP.
* Thus memory addresses are different
*/
}
}
Comparing String content using equals() method
When we want to compare the contents of two String objects, we can use equals() method.
String s1 = "Shankar";
String s2 = "Shankar";
s1.equals(s2); //return true as the content is same for s1 and s2
package com.javatrainingschool;
public class EqualsMethodComparison {
public static void main(String[] args) {
String s1 = "Shankar";
String s2 = "Shankar";
System.out.println(s1.equals(s2));
}
}
Output:
true
Another Example:
package com.javatrainingschool;
public class EqualsMethodComparison {
public static void main(String[] args) {
String s1 = "Shankar";
String s2 = new String("Shankar");
System.out.println(s1.equals(s2));
}
}
Output:
true
equalsIgnoreCase() method
There is another method equalsIgnoreCase() which doesn’t consider case of two Strings being compared.
package com.javatrainingschool;
public class EqualsMethodComparison {
public static void main(String[] args) {
String s1 = "Shankar";
String s2 = new String("shAnkar");
System.out.println(s1.equalsIgnoreCase(s2));
}
}
Output:
true