Create SOAP web service with Axis2 and maven in eclipse
Step 1 :
Create a maven project but don’t select “Create a simple project (skip archetype selection)”
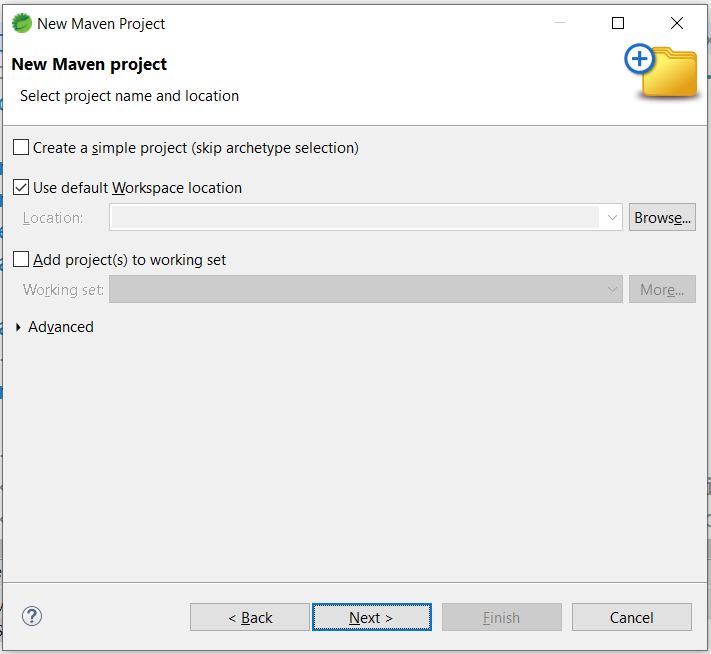
Step 2 :
Type maven-archetype-web in the filter and choose the option as shown in the below diagram
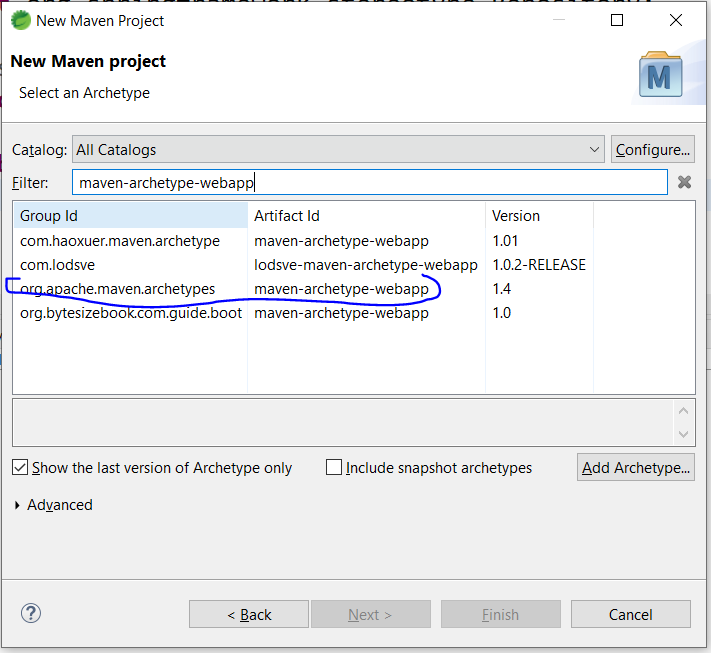
Step 3 :
Enter the values as given in the below screenshot
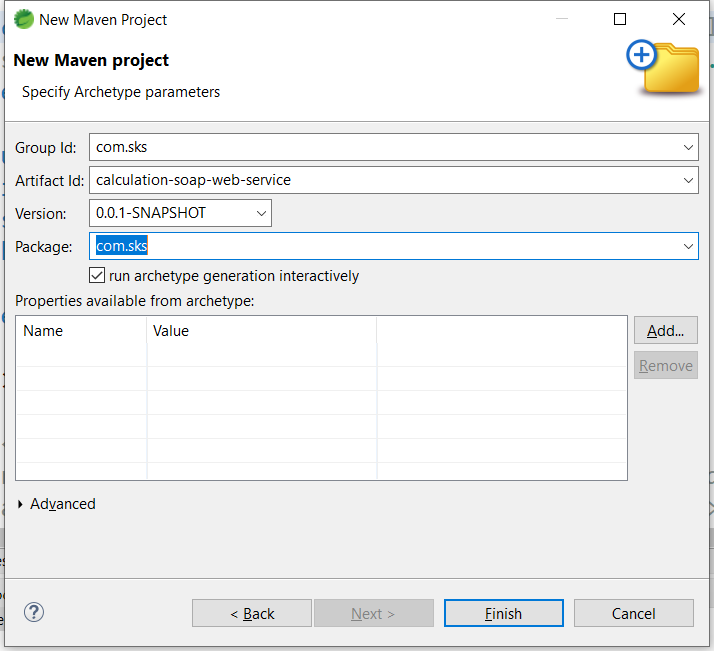
It will create the project interactively. Thus, enter ‘y’ wherever asked.
Once the project is created successfully, you will get build success as below
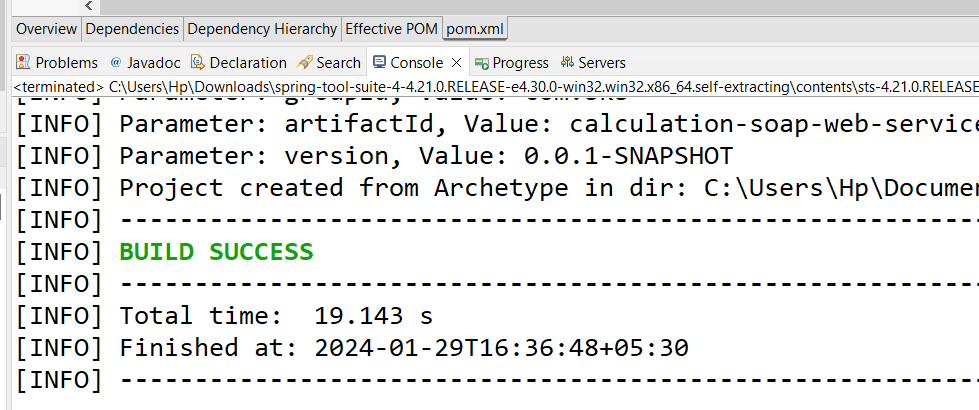
Step 4 : As you expand the project, below should be the structure. Delete the file index.jsp as shown below
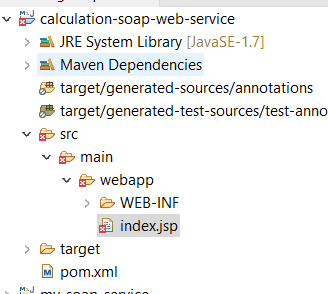
Step 5 : Create below folder structures as shown in the screenshot
following four folder structures need to be created
src/main/java, src/main/resources, src/test/java, src/test/resources
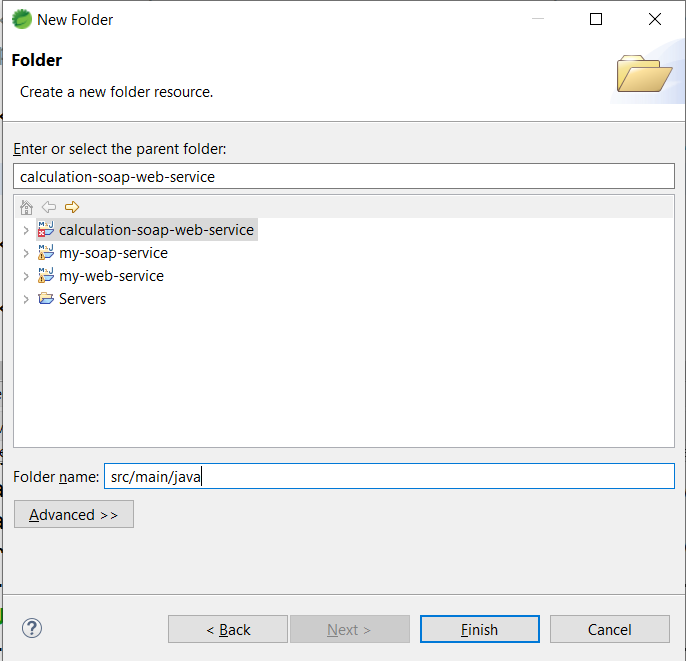
now, the project should look like this
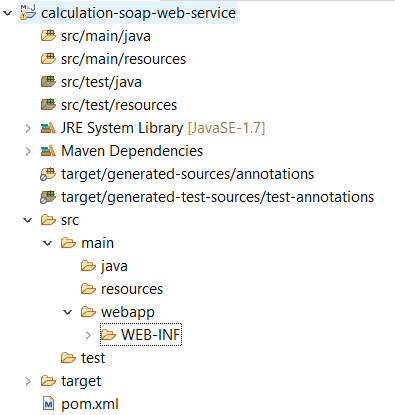
Step 6 : As the project is created. update the pom.xml as follows
- comment the existing <build> </build> tag. And add the new <build></build> tag as shown in the below pom.xml
- Add the below dependencies
- Update the <properties> tag
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.sks</groupId>
<artifactId>calculation-soap-web-service</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<name>calculation-soap-web-service Maven Webapp</name>
<!-- FIXME change it to the project's website -->
<url>http://www.example.com</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<axis2.version>1.7.4</axis2.version>
<log4j.version>1.2.16</log4j.version>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>servlet-api</artifactId>
<version>2.5</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.apache.axis2</groupId>
<artifactId>axis2-kernel</artifactId>
<version>${axis2.version}</version>
</dependency>
<dependency>
<groupId>org.apache.axis2</groupId>
<artifactId>axis2-adb</artifactId>
<version>${axis2.version}</version>
</dependency>
<dependency>
<groupId>org.apache.axis2</groupId>
<artifactId>axis2-transport-http</artifactId>
<version>${axis2.version}</version>
</dependency>
<dependency>
<groupId>org.apache.axis2</groupId>
<artifactId>axis2-transport-local</artifactId>
<version>${axis2.version}</version>
</dependency>
<dependency>
<groupId>org.apache.axis2</groupId>
<artifactId>axis2-xmlbeans</artifactId>
<version>${axis2.version}</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>${log4j.version}</version>
</dependency>
</dependencies>
<!-- <build>
<finalName>my-soap-service</finalName>
<pluginManagement> lock down plugins versions to avoid using Maven defaults
(may be moved to parent pom)
<plugins>
<plugin>
<artifactId>maven-clean-plugin</artifactId>
<version>3.1.0</version>
</plugin>
see
http://maven.apache.org/ref/current/maven-core/default-bindings.html#Plugin_bindings_for_war_packaging
<plugin>
<artifactId>maven-resources-plugin</artifactId>
<version>3.0.2</version>
</plugin>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
</plugin>
<plugin>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.1</version>
</plugin>
<plugin>
<artifactId>maven-war-plugin</artifactId>
<version>3.2.2</version>
</plugin>
<plugin>
<artifactId>maven-install-plugin</artifactId>
<version>2.5.2</version>
</plugin>
<plugin>
<artifactId>maven-deploy-plugin</artifactId>
<version>2.8.2</version>
</plugin>
</plugins>
</pluginManagement>
</build>-->
<build>
<plugins>
<plugin>
<groupId>org.apache.axis2</groupId>
<artifactId>axis2-java2wsdl-maven-plugin</artifactId>
<version>1.7.4</version>
<executions>
<execution>
<phase>process-classes</phase>
<goals>
<goal>java2wsdl</goal>
</goals>
</execution>
</executions>
<configuration>
<className>com.sks.CalculationService</className>
<outputFileName>
${project.build.directory}/CalculationService.wsdl</outputFileName>
</configuration>
</plugin>
</plugins>
</build>
</project>
Step 7 :
Update web.xml like below
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" version="3.0">
<display-name>SampleSoapService</display-name>
<servlet>
<servlet-name>AxisServlet</servlet-name>
<servlet-class>org.apache.axis2.transport.http.AxisServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>AxisServlet</servlet-name>
<url-pattern>/services/*</url-pattern>
</servlet-mapping>
</web-app>
Write a file named services.xml and keep under the below directory
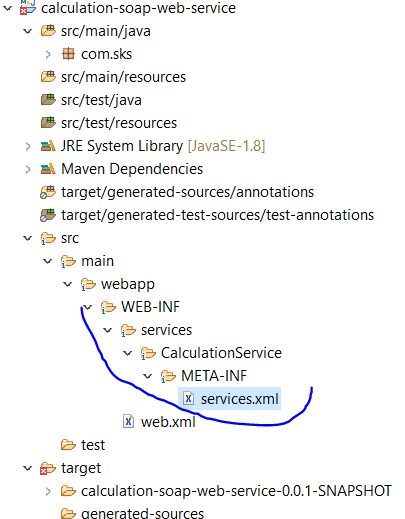
Content of services.xml
<?xml version="1.0" encoding="UTF-8"?>
<serviceGroup>
<service name="CalculationService" targetNamespace="http://webservice.MavenAxis2WebService/">
<description>Calculation service</description>
<schema schemaNamespace="http://webservice.MavenAxis2WebService/" />
<parameter name="ServiceClass" locked="false">com.sks.CalculationServiceImpl</parameter>
</service>
</serviceGroup>
Step 8 :
Write below classes and interface
Operand.java
package com.sks;
public class Operand {
private int num1;
private int num2;
public int getNum1() {
return num1;
}
public void setNum1(int num1) {
this.num1 = num1;
}
public int getNum2() {
return num2;
}
public void setNum2(int num2) {
this.num2 = num2;
}
@Override
public String toString() {
return "Operand [num1=" + num1 + ", num2=" + num2 + "]";
}
}
CalculationService.java interface
package com.sks;
public interface CalculationService {
int add(Operand op);
int multiply(Operand op);
}
CalculationServiceImpl.java
package com.sks;
public class CalculationServiceImpl implements CalculationService {
@Override
public int add(Operand op) {
return op.getNum1() + op.getNum2();
}
@Override
public int multiply(Operand op) {
return op.getNum1() * op.getNum2();
}
}
Step 9 :
Build the application by Run As -> Maven install
After successful build, you should get “Build Success”
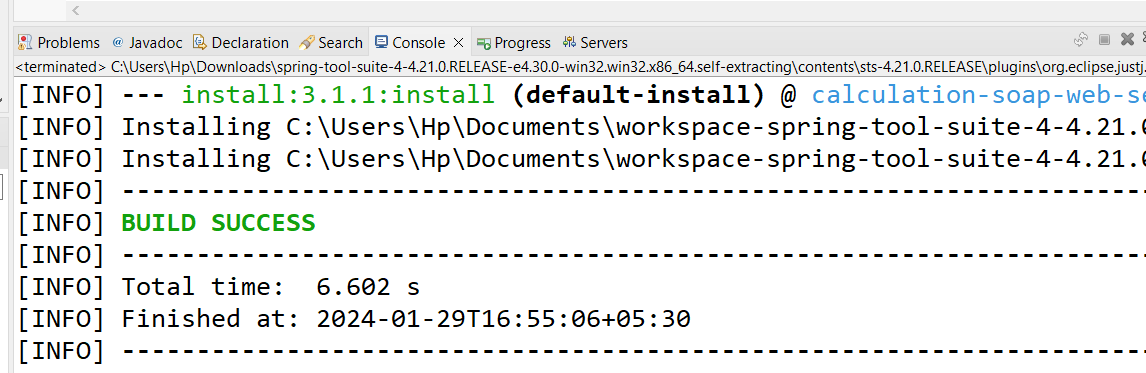
Expand the target folder, war file and a wsdl file should be created
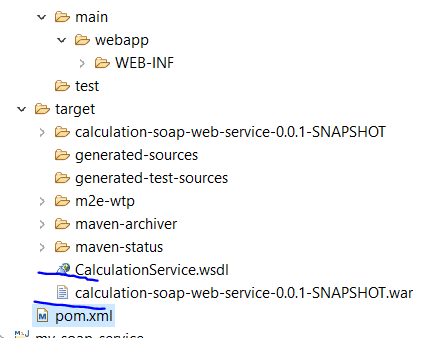
Step 10 :
Now, we need to deploy this war file into tomcat below. And start the server.
If you don’t know how to deploy on tomcat. Click Here
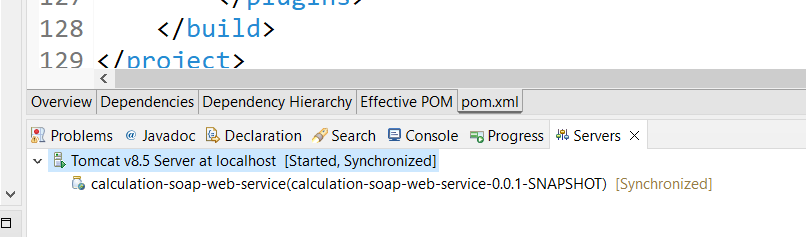
Step 11 :
Access the wsdl file using the below url.
http://localhost:8080/calculation-soap-web-service/services/CalculationService?wsdl
Please note that in the above url, “calculation-soap-web-service” is the context root of the project. If you face any issue in finding the context root of your project, refer the below screenshot
Right click on the project -> properties
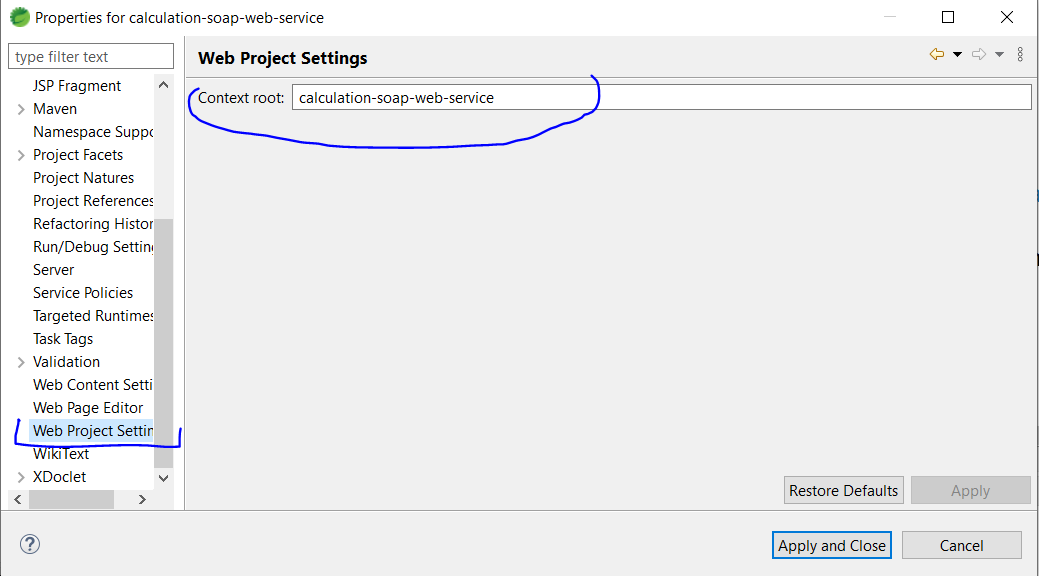
http://localhost:8080/calculation-soap-web-service/services/CalculationService?wsdl
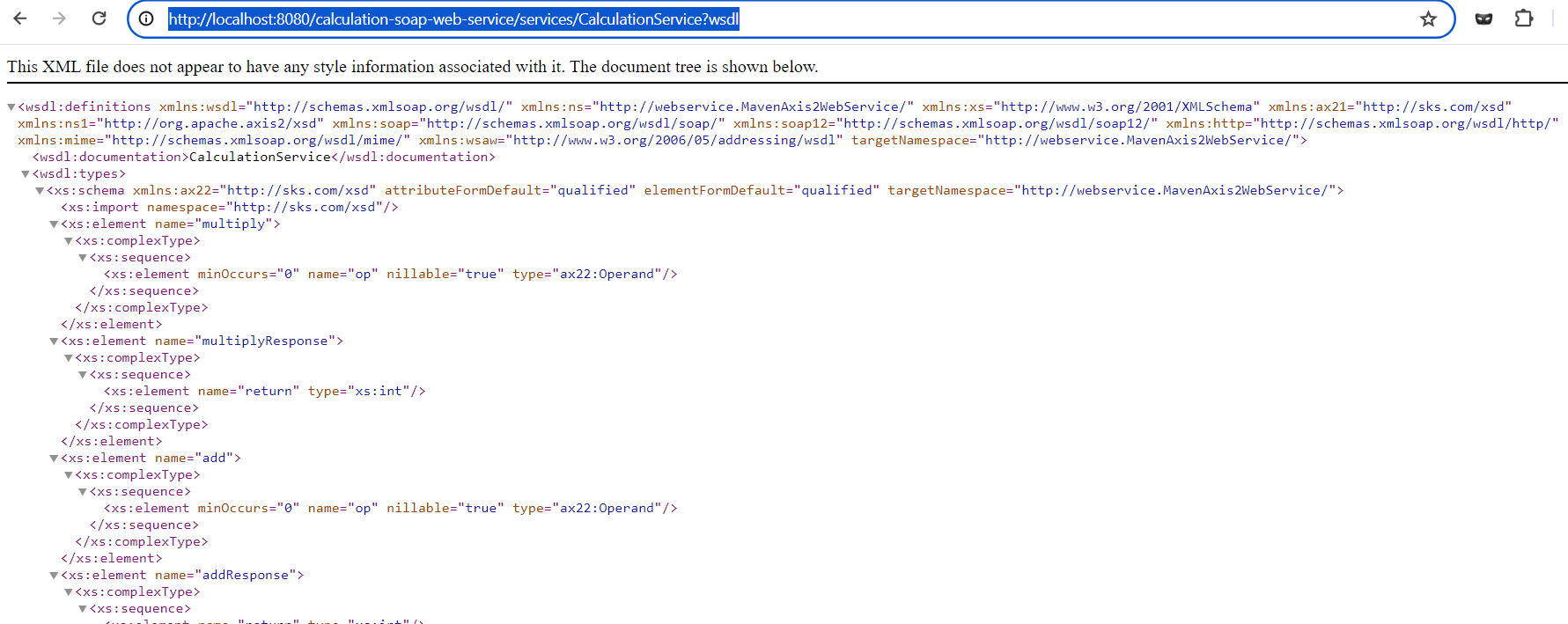
Test the Web Service using SOAP UI
Create a new project in soap. Give any name of your choice. Enter the wsdl url in Initial WSDL as shown below
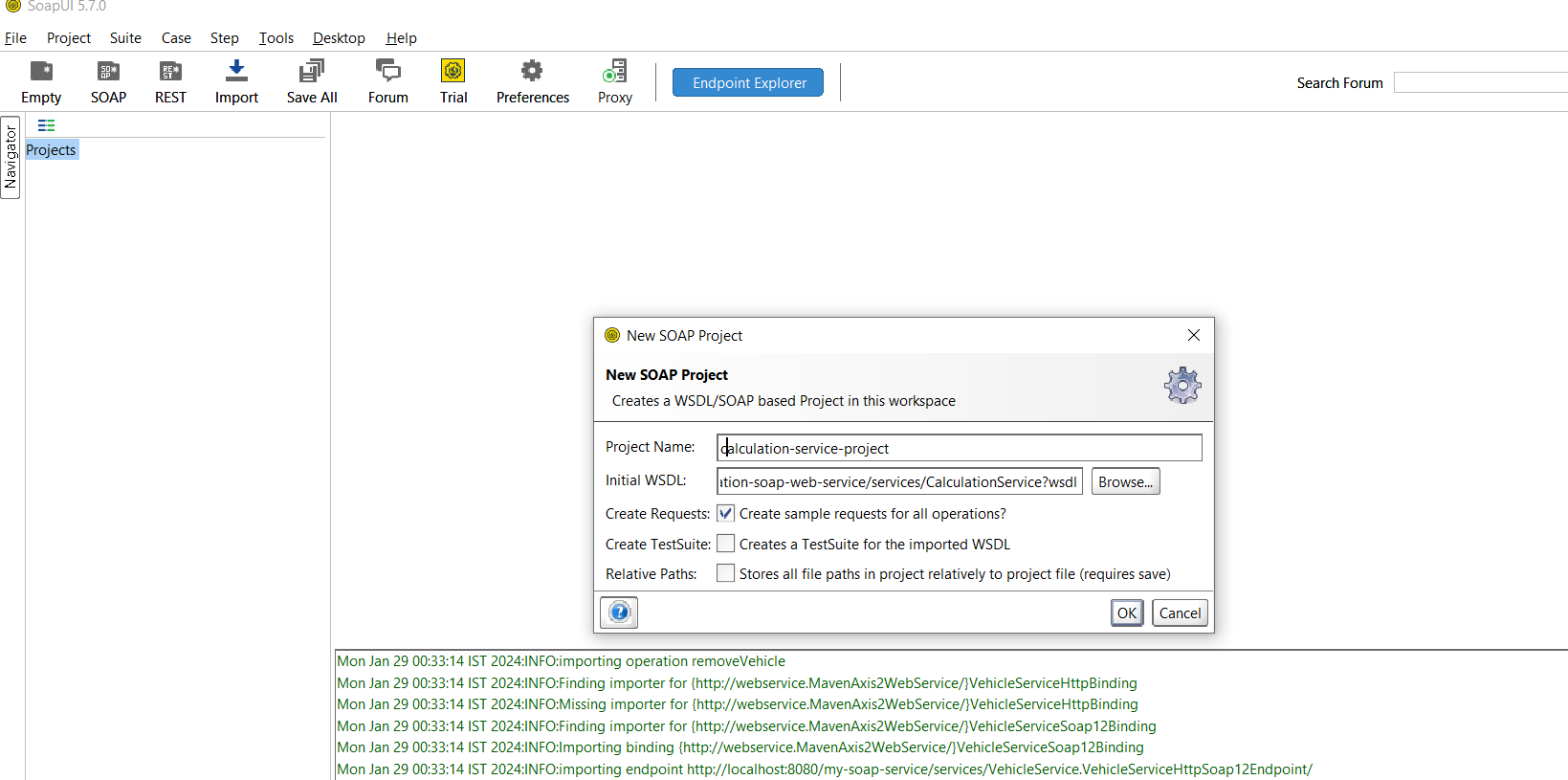
Once the project is created, it will have sample request/response on the left side pane. Give some values and test
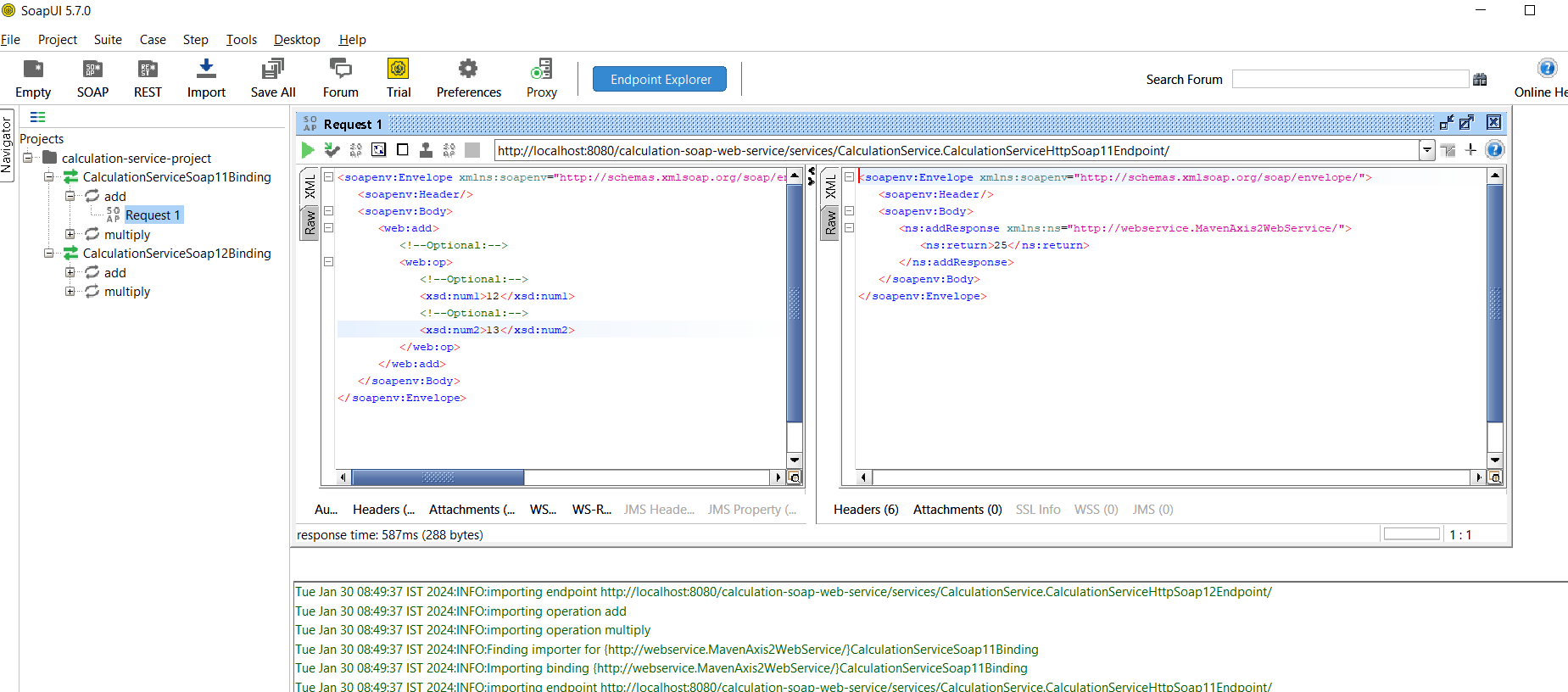
It was quite an effort to create your web service. You deserve a cup of fine coffee. Enjoy
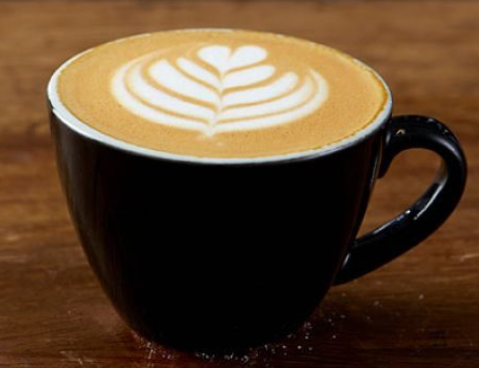