Dockerize Spring Boot App
In this tutorial, we will dockerize a spring boot application.
Build a simple microservice
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.1.2</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.jts</groupId>
<artifactId>boot-with-docker</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>boot-with-docker</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Controller class
package com.jts;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class SimpleController {
@RequestMapping("/hello")
public String hello() {
return "Welcome to spring boot. Let's dockerize this application";
}
}
Package the application
run as -> maven package or maven install
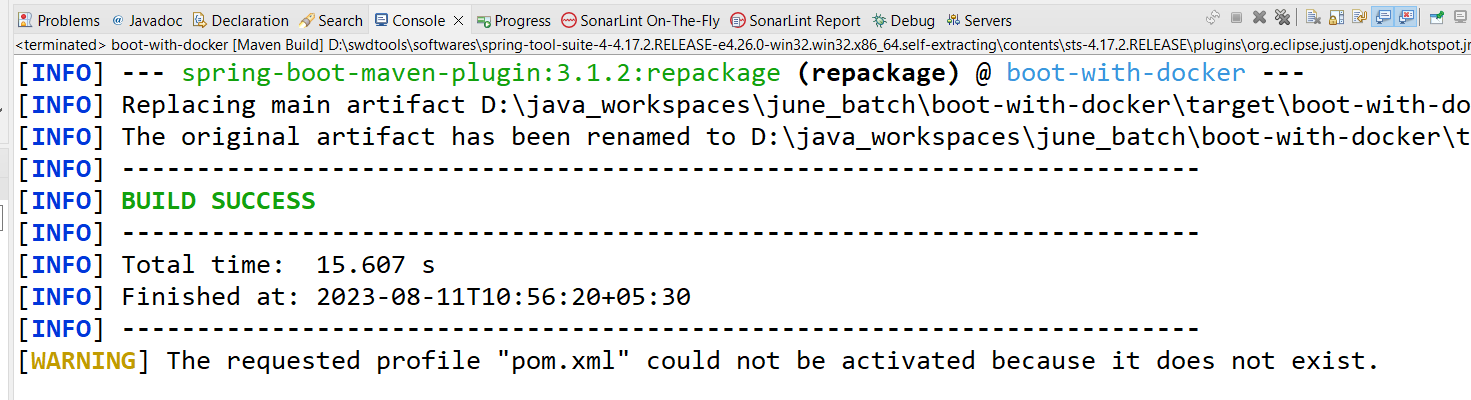
Now, go the project root folder and create a file Dockerfile there
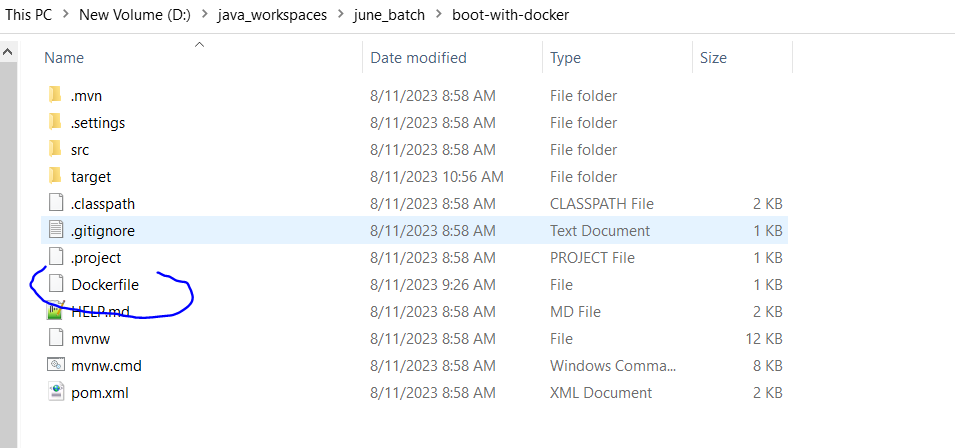
Content of the Dockerfile is as below
FROM openjdk:18
WORKDIR /app
COPY ./target/boot-with-docker-0.0.1-SNAPSHOT.jar /app
EXPOSE 8080
CMD ["java", "-jar", "boot-with-docker-0.0.1-SNAPSHOT.jar"]
Create the docker image
Run the below command
docker build -t docker-boot-app:firstImage .
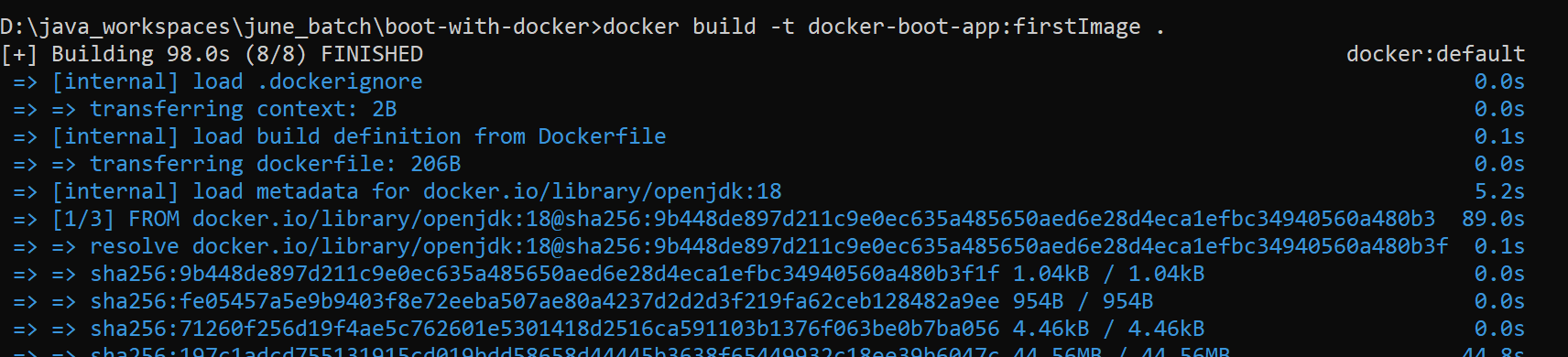
Create docker container by running below command
docker run -d -p 8080:8080 –name firstImage docker-boot-app:firstImage

You can verify on Docker Desktop that the container is running now
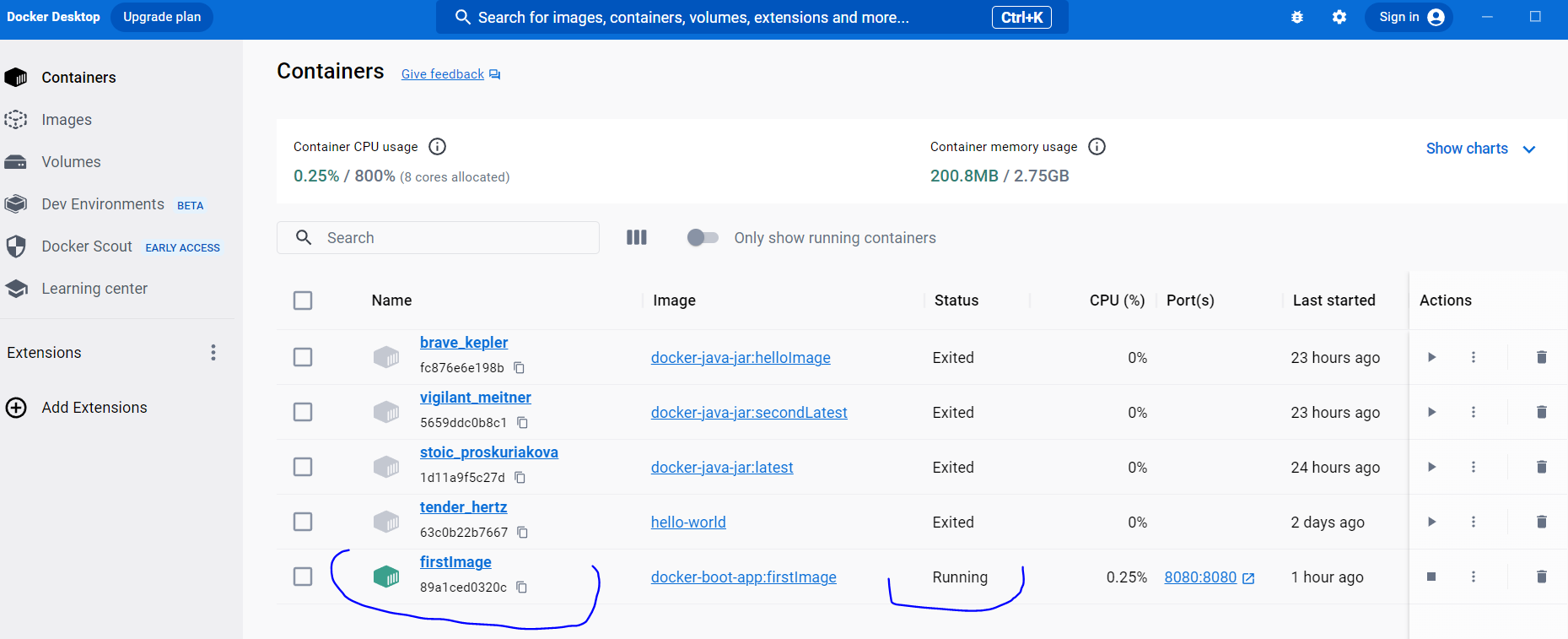
Test on the browser
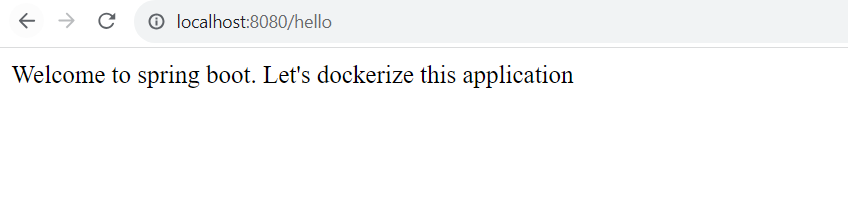
Please note that this application is running on Docker. You can see that your local server is stopped, still you can access the url. This is because the app is running of the Docker.