Factory Design Pattern
Factory design pattern separates the similar type of objects creation from their implementation. It is used where there are classes which inherits the same class or implement the same interface. A factory class provides the method to get object of a specific class from this hierarchy based on the client requirement.
Let’s understand this from the below example.
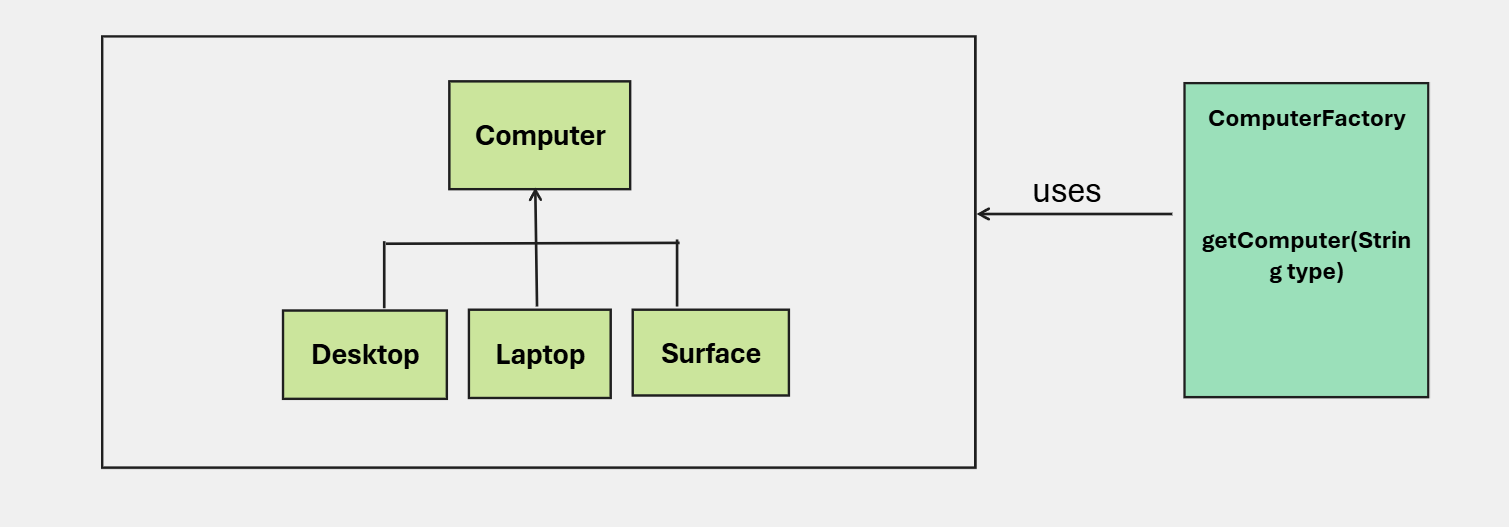
In the above diagram, whichever type of object the client needs, it will ask the ComputerFactory by calling its getComputer(String type) method, and the ComputerFactory will give it that object. Let’s see the example below.
Computer.java
package com.sks;
public abstract class Computer {
protected String OS;
protected String brand;
//getters and setters
//constructors
//toString
}
Desktop.java
package com.sks;
public class Desktop extends Computer {
private String keyboardType;
//getters and setters
public Desktop(String oS, String brand, String keyboardType) {
super(oS, brand);
this.keyboardType = keyboardType;
}
@Override
public String toString() {
return "Desktop [keyboardType=" + keyboardType + ", OS=" + OS + ", brand=" + brand + "]";
}
}
Laptop.java
package com.sks;
public class Laptop extends Computer {
private boolean isMouseProvided;
//getters and setters
public Laptop(String oS, String brand, boolean isMouseProvided) {
super(oS, brand);
this.isMouseProvided = isMouseProvided;
}
@Override
public String toString() {
return "Laptop [isSeparateMouseProvided=" + isMouseProvided + "]";
}
}
Surface.java
package com.sks;
public class Surface extends Computer {
private boolean isKeyboardProvided;
//getters and setters
public Surface(String oS, String brand, boolean isKeyboardProvided) {
super(oS, brand);
this.isKeyboardProvided = isKeyboardProvided;
}
@Override
public String toString() {
return "Surface [isKeyboardProvided=" + isKeyboardProvided + ", OS=" + OS + ", brand=" + brand + "]";
}
}
ComputerFactory.java
package com.sks;
public class ComputerFactory {
public static Computer getComputer(String type) {
Computer c = null;
switch (type) {
case "Desktop":
c = new Desktop("Windows", "HP", "querty");
break;
case "Laptop":
c = new Laptop("Windows", "HP", true);
break;
case "Surface":
c = new Surface("Windows", "HP", true);
}
if(c == null) {
throw new RuntimeException("Please provide a valid input");
}
return c;
}
}
FactoryPatternTest.java
package com.sks;
public class FactoryPatternTest {
public static void main(String[] args) {
Computer surface = ComputerFactory.getComputer("Surface");
System.out.println("Computer details : " + surface);
Computer desktop = ComputerFactory.getComputer("Desktop");
System.out.println("Computer details : " + desktop);
}
}
Output:
