Hibernate Example
In this tutorial, we will create one simple java application with hibernate capabilities. Also, we will use Orace as the database. We will do the following steps:
- Create a maven project and add hibernate dependency, Oracle driver dependency
- Create hibernate mapping file
- Create the pojo class whose objects will be persisted in the database
- Create mapping file for pojo class
- Create the main class for testing
1. Create maven project
Create a maven project and add hibernate-core dependency
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.javatrainingschool</groupId>
<artifactId>hibernate-example</artifactId>
<version>0.0.1-SNAPSHOT</version>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.3.1.Final</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.30</version>
</dependency>
<dependency>
<groupId>jakarta.xml.bind</groupId>
<artifactId>jakarta.xml.bind-api</artifactId>
<version>2.3.2</version>
</dependency>
<!-- Runtime, com.sun.xml.bind module -->
<dependency>
<groupId>org.glassfish.jaxb</groupId>
<artifactId>jaxb-runtime</artifactId>
<version>2.3.2</version>
</dependency>
</dependencies>
</project>
Note -> If you are using MySQL database, you need to add mysql-connector dependency as below
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.30</version>
</dependency>
2. Create hibernate mapping file
Create a file named hibernate.cfg.xml in the resources folder of your maven application. In this file, we will provide connection details to connect to the database (Oracle in this case). Please note that this file’s name should be hibernate.cfg.xml only.
In the below file xepdb1 is the database name.
2.1 Mapping file for oracle database
<?xml version='1.0' encoding='UTF-8'?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hbm2ddl.auto">update</property>
<property name="dialect">org.hibernate.dialect.Oracle9iDialect</property>
<property name="connection.url">jdbc:oracle:thin:@localhost:1521/xepdb1</property>
<property name="connection.username">system</property>
<property name="connection.password">test</property>
<property name="connection.driver_class">oracle.jdbc.driver.OracleDriver</property>
<mapping resource="player.hbm.xml"/>
</session-factory>
</hibernate-configuration>
2.2 Mapping file for MySQL database
<?xml version='1.0' encoding='UTF-8'?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hbm2ddl.auto">update</property>
<property name="dialect">org.hibernate.dialect.MySQL8Dialect</property>
<property name="connection.url">jdbc:mysql://localhost:3306/employee_db</property>
<property name="connection.username">root</property>
<property name="connection.password">password</property>
<property name="connection.driver_class">com.mysql.jdbc.Driver</property>
<property name="show_sql">true</property>
<mapping resource="player.hbm.xml"/>
</session-factory>
</hibernate-configuration>
3. Create pojo class Player
Object of this class will be mapped in the database
package com.javatrainingschool;
public class Player {
private int id;
private String name;
private String country;
//getters/setters
//constructors
//toString()
}
4. Create mapping file for Player class
Create a mapping file for persistent class Player. Naming convention for this file is <class-name>.hbm.xml
player.hbm.xml
<?xml version='1.0' encoding='UTF-8'?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="com.javatrainingschool.Player" table="player">
<id name="id">
<generator class="assigned"></generator>
</id>
<property name="name"></property>
<property name="country"></property>
</class>
</hibernate-mapping>
5. Create the main class
In this class, we will create main method.
package com.javatrainingschool;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.boot.Metadata;
import org.hibernate.boot.MetadataSources;
import org.hibernate.boot.registry.StandardServiceRegistry;
import org.hibernate.boot.registry.StandardServiceRegistryBuilder;
public class PlayerMain {
public static void main(String[] args) {
//Create ServiceRegistry object
StandardServiceRegistry ssr = new StandardServiceRegistryBuilder().configure("hibernate.cfg.xml").build();
Metadata meta = new MetadataSources(ssr).getMetadataBuilder().build();
//Create session factory object
SessionFactory factory = meta.getSessionFactoryBuilder().build();
Session session = factory.openSession();
Transaction t = session.beginTransaction();
Player p1 = new Player(1, "Neymar", "Brazil");
session.save(p1);
Player p2 = new Player(2, "Christiano Ronaldo", "Portugal");
session.save(p2);
Player p3 = new Player(3, "Lionel Messi", "Argentina");
session.save(p3);
t.commit();
System.out.println("Players saved successfully.");
factory.close();
session.close();
}
}
Output :
Players saved successfully.
Connect to the database and check the records in the table player. There should be 3 entries of players as shown below.
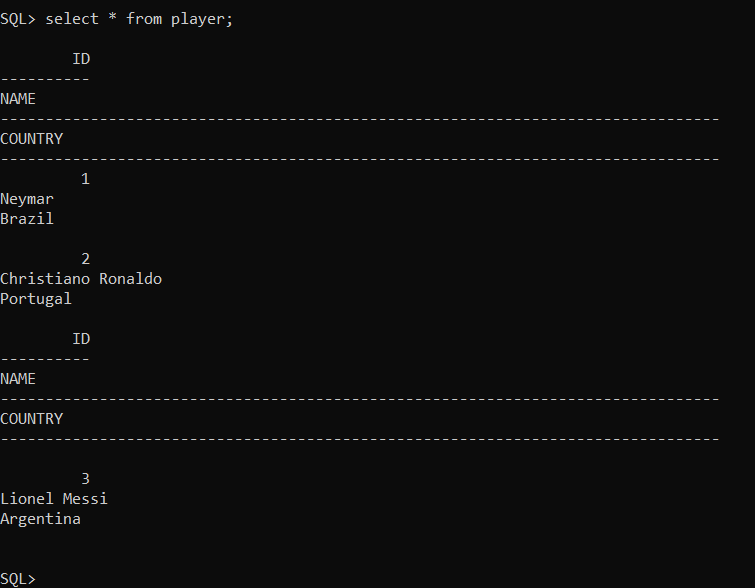