Interservice Communication
In this tutorial, we will see how to call one service from another. For that, let’s first create a service with one simple get mapping and one post mapping.
Step 1 : Create one microservice using spring starter project in sts.
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.4</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.javatrainingschool</groupId>
<artifactId>calculationService</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>calculationService</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Let’s define one getMapping and one PostMapping in the controller class
CalculationController.java
package com.javatraining;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/calc")
public class CalculationController {
@GetMapping("/info")
public String info() {
return "This is a simple get mapping from Calculation controller";
}
@PostMapping("/multiply")
public String multiply(@RequestBody Numbers num) {
int result = num.getNum1() * num.getNum2();
return "the result = " + result;
}
}
Numbers.java
package com.javatraining;
public class Numbers {
private int num1;
private int num2;
public int getNum1() {
return num1;
}
public void setNum1(int num1) {
this.num1 = num1;
}
public int getNum2() {
return num2;
}
public void setNum2(int num2) {
this.num2 = num2;
}
}
Define a port on which the server is to run
application.properties file
server.port=8081
Run and test the service
http://localhost:8081/calc/info

Step 2 : Create second Service
Create another service. From this service, we will call the above service
pom.xml for this service is like below
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.4</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.javatrainingschool</groupId>
<artifactId>getService</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>getService</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Add Below to the main class
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
package com.javatraining;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class GetServiceApplication {
public static void main(String[] args) {
SpringApplication.run(BootWithMongoApplication.class, args);
}
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
Controller for this service
package com.javatraining;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.client.RestTemplate;
@RestController
@RequestMapping("/get")
public class GetController {
@Autowired
RestTemplate restTemplate;
@GetMapping("/calcInfo")
public String getCalcInfo() {
String url="http://localhost:8081/calc/info";
return restTemplate.getForObject(url, String.class);
}
@PostMapping("/multiply")
public ResponseEntity<String> multiply(@RequestBody Numbers num){
String url="http://localhost:8081/calc/multiply";
ResponseEntity<String> result = restTemplate.postForEntity
(url, num, String.class);
return result;
}
}
Numbers.java
package com.javatraining;
public class Numbers {
private int num1;
private int num2;
public int getNum1() {
return num1;
}
public void setNum1(int num1) {
this.num1 = num1;
}
public int getNum2() {
return num2;
}
public void setNum2(int num2) {
this.num2 = num2;
}
}
add below property
server.port=8082
Run the service and test
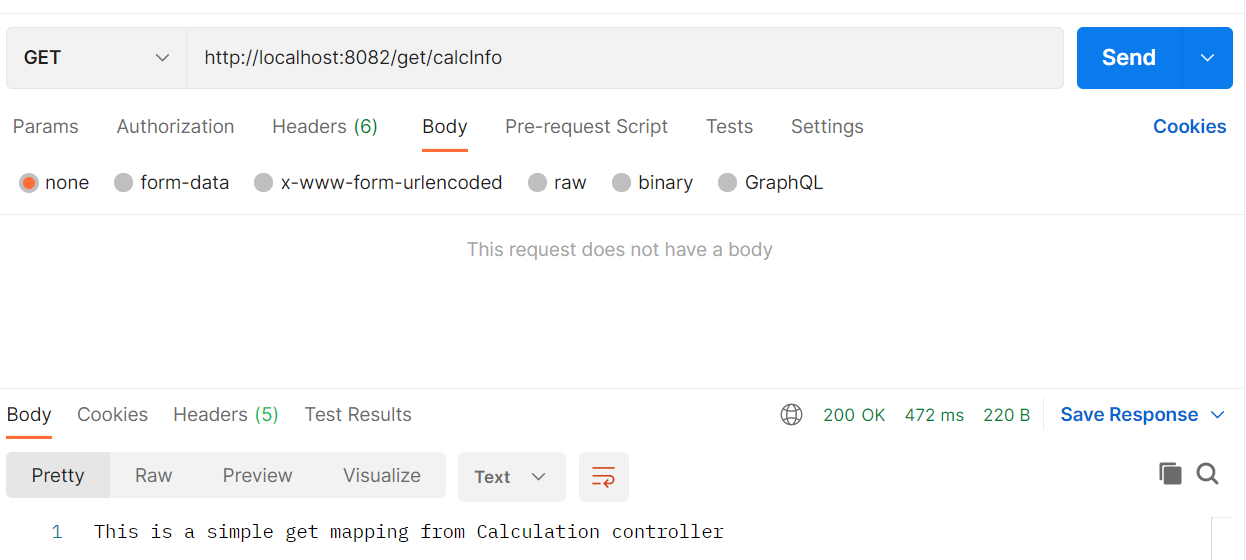
Post Request
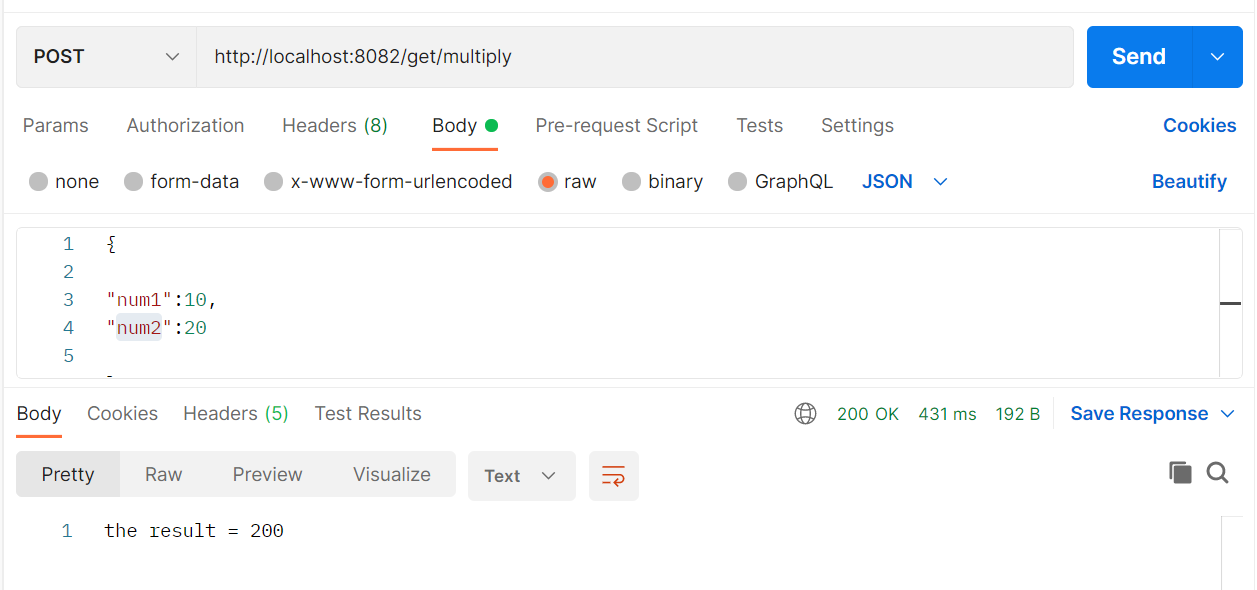