Lombok api
In java, we need to write a lot of boilerplate code like getters/setters method, toString methd, constructors etc. The solution to reduce repititive code is lombok api. Add below dependency in your pom.xml
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.20</version>
<scope>provided</scope>
</dependency>
Getters/ setters and no-arg constructor using lombok
To generate getters and setters, add below annotations
@Entity
@Getter @Setter @NoArgsConstructor // <--- THIS is it
public class User implements Serializable {
private @Id Long id; // will be set when persisting
private String firstName;
private String lastName;
private int age;
public User(String firstName, String lastName, int age) {
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
}
}
Constructors with arguments
@RequiredArgsConstructor
@Accessors(fluent = true) @Getter
public class Scientist {
private final @NonNull int id;
private final @NonNull String name;
private final @NonNull String field;
}
Equals and hashcode and toString method
Use @ToString and @EqualsAndHashCode
For Thread safe methods
Use @Synchronized on the method you want to synchronize
How to install lombok in spring tool suite (STS)
Install lombok library from maven central website
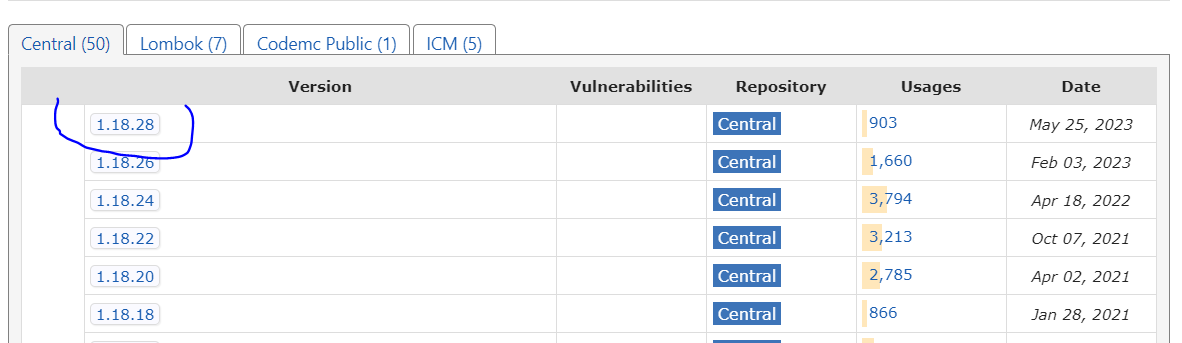
Now, open the command prompt and go to the folder where you have downloaded the jar file and run the below command
java -jar lombok-1.18.28.jar
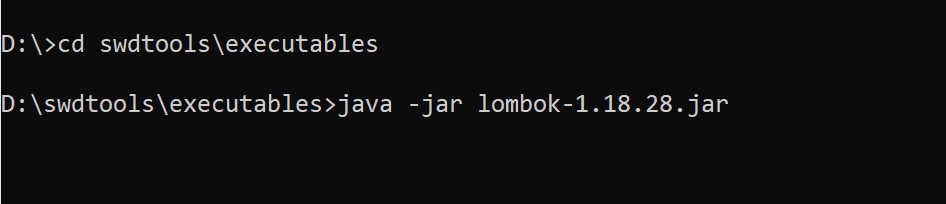
Following window will open
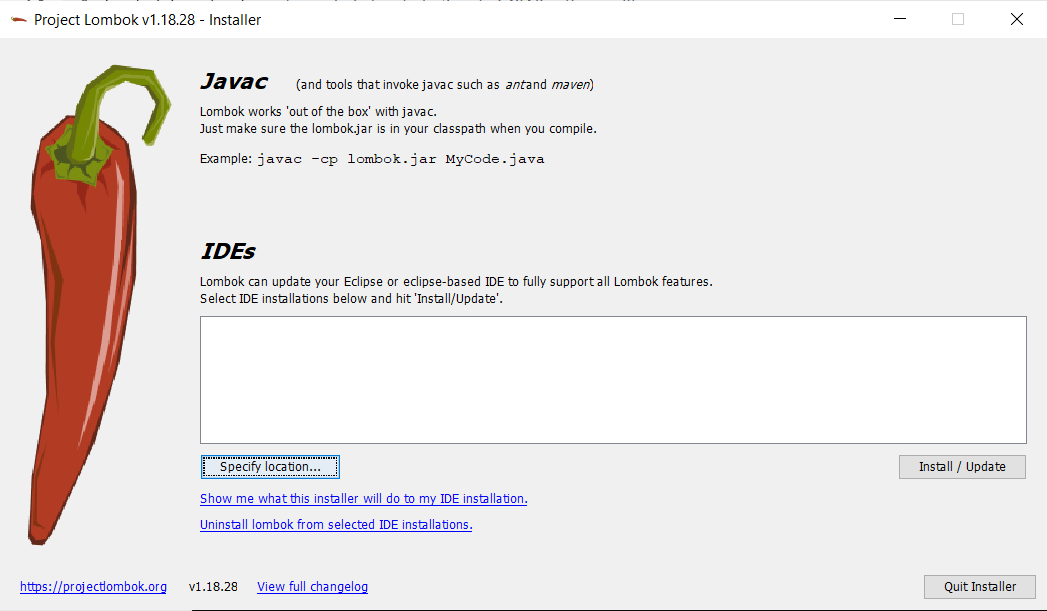
Clink on Specify location and select the .ini file
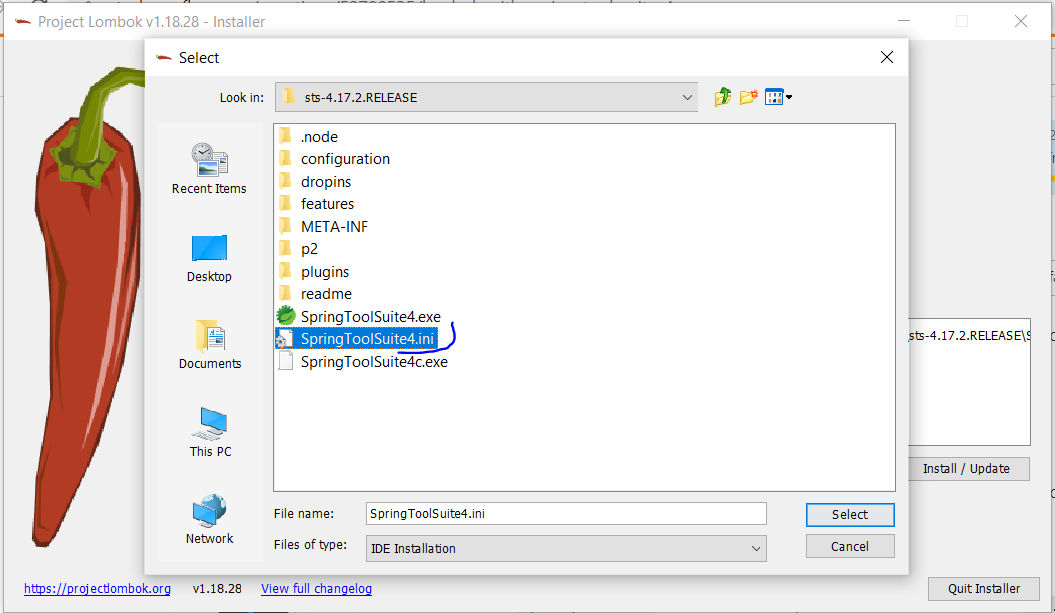
Installation Successful
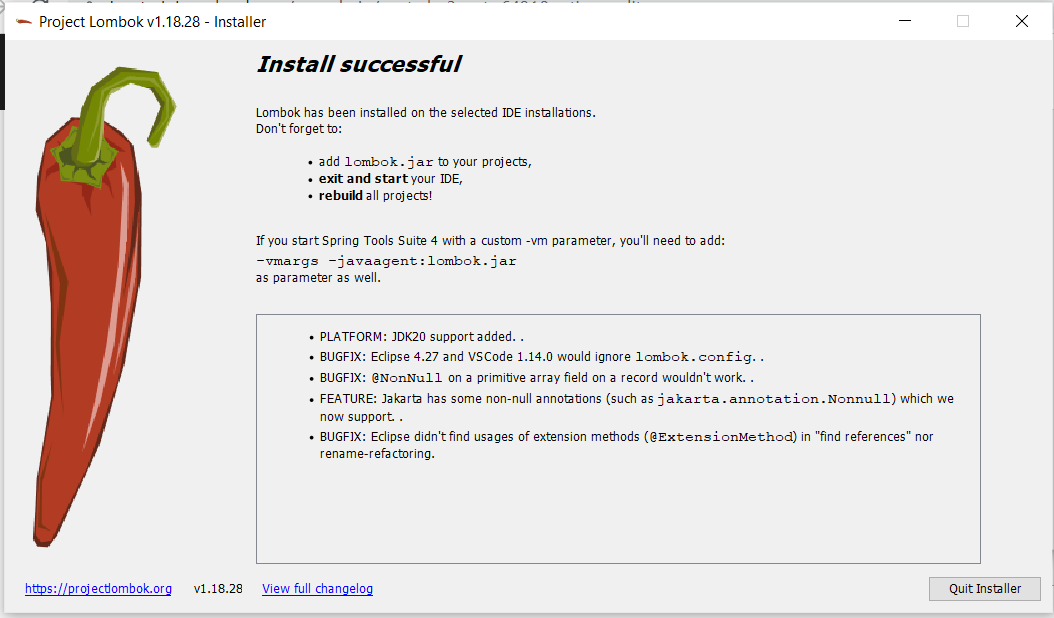
Now, restart you IDE and include lombok.jar in pom.xml of your project and use it.