Prototype Design Pattern
Prototype design pattern is used when several similar objects of a class are required with slight or no modifications.
Let’s understand from this with an example. A client needs 100 cricket bats of exactly same dimensions. The only change can be the sticker on it. Some bats may have MRF sticker, some BDM, some A Sports, some Puma etc. So, the client goes to a cricket bat manufacturer with a prototype bat and asks it to make 100 similar bats. Let’s implement this scenario with Prototype pattern.
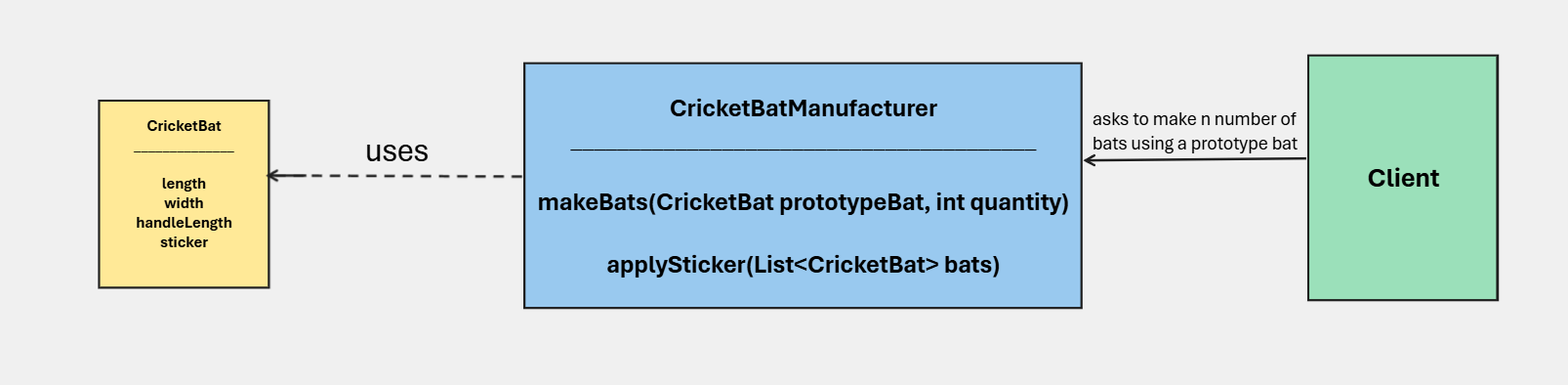
CricketBat.java
package com.sks.prototype;
public class CricketBat {
private int length;
private float width;
private int handleLength;
//sticker can be different
private String sticker;
public CricketBat clone() {
return new CricketBat(this.length, this.width, this.handleLength);
}
public CricketBat(int length, float width, int handleLength) {
super();
this.length = length;
this.width = width;
this.handleLength = handleLength;
}
public void setSticker(String sticker) {
this.sticker = sticker;
}
@Override
public String toString() {
return "CricketBat [length=" + length + ", width=" + width + ", handleLength=" + handleLength + ", sticker="
+ sticker + "]";
}
}
CricketBatManufacturer.java
package com.sks.prototype;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class CricketBatManufacturer {
enum stickers {
MRF, CEAT, PUMA, ADDIDAS
};
public static List<CricketBat> makeBats(CricketBat prototypeBat, int quantity) {
List<CricketBat> totalBats = new ArrayList<>();
for (int i = 1; i <= quantity; i++) {
totalBats.add(prototypeBat.clone());
}
return totalBats;
}
//apply stickers randomly from the enum list
public static void applySticker(List<CricketBat> bats) {
for (CricketBat b : bats) {
int index = new Random().nextInt(stickers.values().length);
String sticker = stickers.values()[index].name();
b.setSticker(sticker);
}
}
}
CricketBatPrototypeTest.java
package com.sks.prototype;
import java.util.List;
public class CricketBatPrototypeTest {
public static void main(String[] args) {
CricketBat prototypeBat = new CricketBat(36, 4.25f, 11);
int quantity = 10;
List<CricketBat> bats = CricketBatManufacturer.makeBats(prototypeBat, quantity);
CricketBatManufacturer.applySticker(bats);
bats.forEach(b -> System.out.println(b));
}
}
Output:
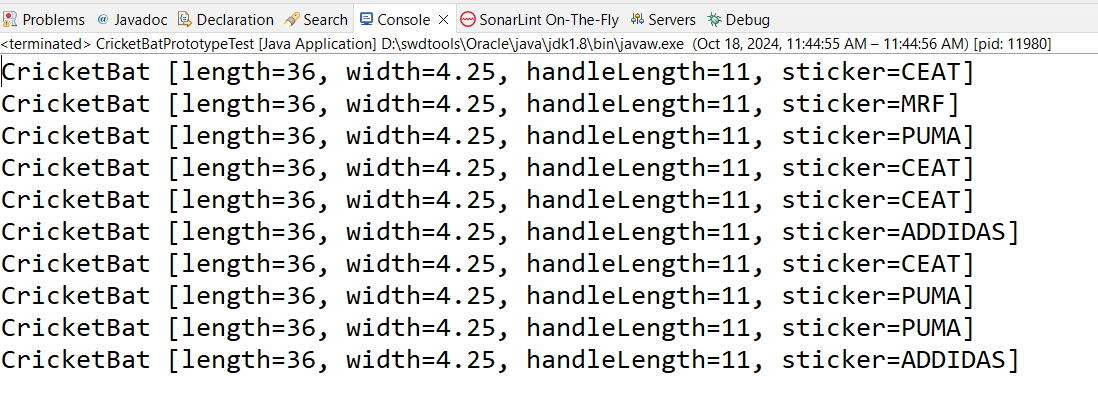