StringBuilder
StringBuilder class is similar to StringBuffer except for the fact that it is non-synchronized (not thread-safe). So, we need to be careful while using StringBuilder class.
- StringBuilder objects are mutable like StringBuffer objects
- StringBuilder class is not thread-safe
Note -> StringBuilder class should be avoided in multithreaded applications. It can be a good choice to be used in single threaded applications since it is faster compared to StringBuffer.
StringBuilder Constructors
StringBuilder()
StringBuilder(String str)
StringBuilder(int length)
StringBuilder Example
package com.javatrainingschool;
public class StringBuilderExample {
public static void main(String[] args) {
StringBuilder sb = new StringBuilder("David");
//adding a space between firstname and lastname
sb.append(" ");
sb.append("Miller");
System.out.println("Name : " + sb);
System.out.println("Length : " + sb.length());
System.out.println("Index of ill : " + sb.indexOf("ill"));
System.out.println("Substring : " + sb.substring(2, 7));
}
}
Output:
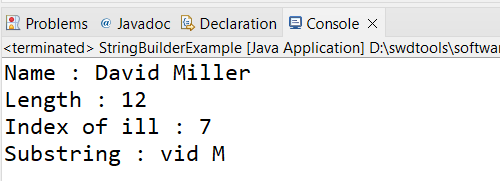
String vs StringBuffer vs StringBuilder
String | StringBuffer | StringBuilder |
---|---|---|
Strings are immutable | StringBuffer objects are mutable | StringBuilder Objects are mutable |
String objects are created in String constant pool. | StringBuffer objects are created in Heap memory | StringBuilder objects are created in Heap memory |
String operations are slower | StringBuffer operations are faster compared to String | StringBuilder operations are even faster compared to StringBuffer because they are non synchronized |
String class overrides equals() method from Object class. So, contents of two strings can be compared using equals() method. | StringBuffer doesn’t override equals method and thus, two StringBuffer objects can’t be compared using equals() method | StringBuilder doesn’t override equals method and thus, two StringBuffer objects can’t be compared using equals() method |