Super Keyword
Super keyword
The super keyword in Java is used to refer immediate parent class object’s data member, methods, and constructors.
Whenever an instance of a subclass is created, an instance of parent class is also created implicitly. This can be referred by super reference variable.
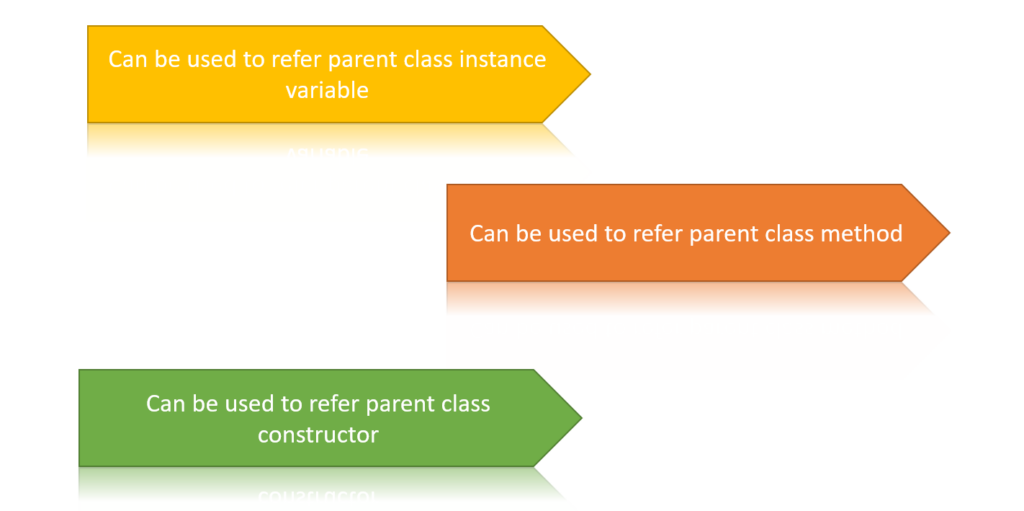
Super example that refers parent class’ instance variable
package com.javatrainingschool;
public class Beverage {
protected String state = "Liquid";
}
package com.javatrainingschool;
public class Tea extends Beverage {
String state = "Brown Liquid";
public void showBeverageState() {
System.out.println("State of tea - " + state);
//using super, we are accessing state variable of parent class Beverage
System.out.println("State of beverage - " + super.state);
}
public static void main(String[] args) {
Tea tea = new Tea();
tea.showBeverageState();
}
}
Output :
State of tea - Brown Liquid
State of beverage - Liquid
Super keyword example for accessing super class method
Super keyword can also be used to access super class’ method.
package com.javatrainingschool;
public class Beverage {
protected String state = "Liquid";
public void showBeverageState() {
System.out.println("State of beverage - " + this.state);
}
}
package com.javatrainingschool;
public class Tea extends Beverage {
String state = "Brown Liquid";
public void showBeverageState() {
System.out.println("State of tea - " + state);
}
public void showState() {
showBeverageState();
//here super is used to refer to super class method
super.showBeverageState();
}
public static void main(String[] args) {
Tea tea = new Tea();
tea.showState();
}
}
Output :
State of tea - Brown Liquid
State of beverage - Liquid
Super keyword example to access super class constructor
package com.javatrainingschool;
public class Beverage {
public Beverage() {
System.out.println("From inside Beverage class constructor");
}
}
package com.javatrainingschool;
public class Tea extends Beverage {
public Tea() {
super(); //this statement will call Beverage class' constructor
System.out.println("From inside Tea class constructor");
}
public static void main(String[] args) {
Tea tea = new Tea();
}
}
Output :
From inside Beverage class constructor
From inside Tea class constructor