ArrayList in Java
ArrayList is the class that implements List interface. Some important points about ArrayList are :
- ArrayList stores objects in an ordered way based on the indexes. The first object is stored at ‘0’ index and the next object is stored at index ‘1’, next object at ‘2’ and so on.
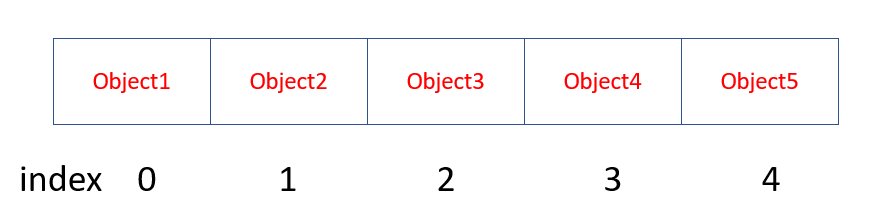
- ArrayList are like arrays in java, but they are dynamic in nature unlike arrays. They can grow dynamically as we add objects to them.
- It maintains indexed based order of elements. If we add an element at a particular index where there is already an element, then all other elements are adjusted accordingly.
- ArrayList does not guarantee uniqueness of elements, which means we can add duplicate objects to it.
Ways to Create an ArrayList
Plain old way
List list = new ArrayList();
Generic way
List<String> list = new ArrayList<String>();
Note -> When you make a list generic, compiler itself will give error if you try to add an object of different type than mentioned in the List<>. In the above example, there will be a compiler error if you try to add an integer object to it.
Let us see one example how we can create a list of objects. In the below example, we are going to create a list of subjects.
package com.javatrainingschool;
import java.util.ArrayList;
import java.util.List;
public class ArrayListExample {
public static void main(String[] args) {
List<String> subjects = new ArrayList<String>();
//add method is used to add elements to the list
subjects.add("Mathematics");
subjects.add("Hindi");
subjects.add("English");
System.out.println("List of subjects is : " + subjects);
}
}
Output :
List of subjects is : [Mathematics, Hindi, English]
How to Iterate Over a List
We can iterate over a list using :
- An iterator – it is an interface in collections framework using which we can traverse or iterate over a collection like list, set, queue.
- For each loop – This loop is used to traverse over a collection. The syntax to use this loop is like below
for (<Object-type-the-collection-stores> <variable-name> : <collection-object>) { //loop body }
e.g. for (String subject : subjects) { //loop body}
Iterator example :
package com.javatrainingschool;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class ArrayListExample {
public static void main(String[] args) {
List<String> subjects = new ArrayList<String>();
subjects.add("Mathematics");
subjects.add("Hindi");
subjects.add("English");
Iterator<String> it = subjects.iterator();
while(it.hasNext()) {
System.out.println("The element is : " + it.next());
}
}
}
Output :
The element is : Mathematics
The element is : Hindi
The element is : English
For Each Loop example :
package com.javatrainingschool;
import java.util.ArrayList;
import java.util.List;
public class ArrayListExample {
public static void main(String[] args) {
List<String> subjects = new ArrayList<String>();
subjects.add("Mathematics");
subjects.add("Hindi");
subjects.add("English");
for (String subject : subjects) {
System.out.println("The element is : " + subject);
}
}
}
Add an Object at a Particular Index
When we are adding an element at a particular index in a list, then we have to make sure that the index is greater than 0 and less than (size of the list – 1). If the index is beyond this boundary, then IndexOutOfBoundsException will be thrown. Consider the below example
package com.javatrainingschool;
import java.util.ArrayList;
import java.util.List;
public class ArrayListExample {
public static void main(String[] args) {
List<String> subjects = new ArrayList<String>();
subjects.add("Mathematics");
subjects.add("Hindi");
subjects.add("English");
int index = 1;
if(index > 0 && index <= (subjects.size()) ) {
subjects.add(index, "Economics");
}
else {
System.out.println("The index " + index + " is greater than the size " + subjects.size() + " of the list.");
}
for (String subject : subjects) {
System.out.println("The element is : " + subject);
}
}
}
Output :
The element is : Mathematics
The element is : Economics
The element is : Hindi
The element is : English
In the above example, if the index is 5. Then the output will be as shown below
Output :
The index 5 is greater than the size 3 of the list.
The element is : Mathematics
The element is : Hindi
The element is : English
How to Get the Size of ArrayList
Using size() method.
List<String> subjects = new ArrayList<String>();
subjects.add("Hindi");
subjects.add("English");
int size = subjects.size();
System.out.println("The size of the list = " + size);
How to Update an Element
Using set(int index, object) method. But we have to make sure that the index is not greater than the size of the list.
subjects.set(2, "Economics");
How to Remove an Element
Using remove(Object o) method
Using remove(int index) method
subjects.remove("Hindi"); //Will remove Hindi from the list
subjects.remove(1); //Will remove object at index 1.
ArrayList Example with Custom Object
package com.javatrainingschool;
import java.util.ArrayList;
import java.util.List;
public class ArrayListCustomObjectExample {
public static void main(String[] args) {
List<Address> addressList = new ArrayList<Address>();
Address add1 = new Address();
add1.setAddressLine("Pitambar Nagar");
add1.setCity("Delhi");
Address add2 = new Address();
add2.setAddressLine("Azad Nagar");
add2.setCity("Mumbai");
addressList.add(add1);
addressList.add(add2);
for (Address address : addressList) {
System.out.println("The address is : " + address);
}
}
}
package com.javatrainingschool;
public class Address {
private String addressLine;
private String city;
public String getAddressLine() {
return addressLine;
}
public void setAddressLine(String addressLine) {
this.addressLine = addressLine;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
@Override
public String toString() {
return "Address [addressLine=" + addressLine + ", city=" + city + "]";
}
}
Output :
The address is : Address [addressLine=Pitambar Nagar, city=Delhi]
The address is : Address [addressLine=Azad Nagar, city=Mumbai]